Introduction
This tip shows the use of AngularJS with .NET MVC5 application. Here is a simple step-by-step example for the use of AngularJs with .NET MVC application.
Background
While searching for an article for the use of AngularJS with .NET MVC technology, the search always shows AngularJS and explains its MVC methodology. But there is nothing much on the topic.
Using the Code - Step by Step
Create a new project and select MVC template and check Web API as well and select OK.
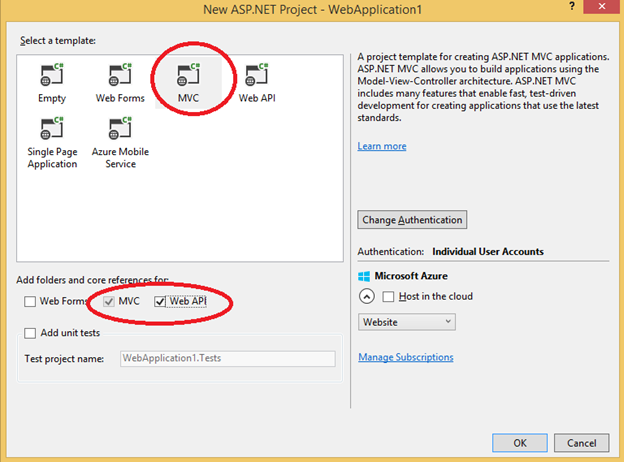
In Model, create a class Category
:
public class Category
{
[Key]
public int Id { get; set; }
public string Name { get; set; }
}
On Controller folder, add New Controller and select Web API2 Controller.

And then, select Category
as Model Class and in controller name, write CategoriesApiController
as shown in the following image:

The controller will automatically create the API methods.
Now, create a new empty Controller
and name it CategoriesController
.

In this controller, create ActionResult Index
.
public class CategoriesController : Controller
{
private ApplicationDbContext db = new ApplicationDbContext();
public ActionResult Index()
{
return View();
}
}
Now, it's time to write AngularJs code before creating the index view. Use Manage NuGet package and install AngularJS.
Now, right click Script folder -> Add -> New Folder and name folder Category.

In Category folder, right-click and Add new item JavaScript File and name the file categoryModule.js and write the following code in it:
var app1;
(function () {
app1 = angular.module("catModule", []);
})();
In Category folder, create a new JavaScript file named “service.js”and add the following code in it. In this file, we have created JavaScript methods to call methods defined in WebAPI controller.
app1.service("catService", function ($http) {
this.getCategories = function () { return $http.get("/api/CategoriesAPI"); }
this.get = function (id) { return $http.get("/api/CategoriesAPI/" + id); }
this.post = function(Category)
{
var request = $http({
method: "post",
url: "/api/CategoriesAPI",
data: Category
});
return request;
}
this.delete = function (Cat) {
var request = $http({
method: "delete",
url: "/api/CategoriesAPI/" + Cat
});
return request;
}
});
Now create another JavaScript file in category folder, name it “CategoryController.js” and paste the following code in it.
app1.controller("catController", function ($scope, catService) {
$scope.IsNewRecord = 1;
loadRecords();
function loadRecords() {
promiseGet = catService.getCategories();
promiseGet.then(function (p1) {
$scope.Categories = p1.data
},
function (errorP1) {
console.log("Error in getting category " + errorP1);
});
}
$scope.get = function (Cat) {
var promiseGetSingle = catService.get(Cat.Id);
promiseGetSingle.then(function (p1) {
var res = p1.data;
$scope.Id = res.Id;
$scope.Name = res.Name;
},
function (errorp1) {
console.log("Error in code " + errorp1);
} );
}
$scope.save = function () {
var Category = {
CatId: $scope.CatId,
Name: $scope.CategoryName
};
if($scope.IsNewRecord === 1){
var promisePost = catService.post(Category);
promisePost.then(function(p1){
$scope.CatId = p1.data.CatId;
$scope.CategoryName = "";
loadRecords();
},function(err){console.log('Error '+ err);
});
}else{
var promisePut = catService.put($scope.CatId,Name);
promisePut.then(function(p1){
$scope.Message = "Successfully Updated";
loadRecords();
},function(err){ console.log('Error ' + err); });
} }
$scope.delete = function (id) {
var promiseDelete = catService.delete(id);
promiseDelete.then(function (p1) {
$scope.Message = "Record Deleted successfully";
loadRecords();
},function(err){ console.log("Error "+ err); });
}});
Now we have written all the JavaScript we needed. Now go to CategoryController
created earlier, i.e.,
public ActionResult Index()
{
return View();
}
Right click on index()
and click Add View and add empty view. Add the following code in the index.cshtml file:
<div ng-app="catModule" >
@{ ViewBag.Title = "Index"; }
<div ng-controller="catController">
<h2>Categories</h2>
<table class="table">
<thead>
<tr>
<th><span>Id</span></th>
<th><span>Category</span></th>
</tr>
</thead>
<tbody ng-repeat="Cat in Categories">
<tr>
<td><span> {{Cat.Id}}</span></td>
<td><span>{{Cat.Name}}</span></td>
<td><span><input type="button"
class="btn btn-danger" value="Delete"
ng-click="delete(Cat.Id)" /> </span></td>
</tr>
</tbody>
</table>
<form ng-submit="">
Category <input type="text" ng-model="CategoryName" />
<input type="submit" value="Submit" ng-click="save()" />
</form>
<script src="~/Scripts/angular.js"></script>
<script src="~/Scripts/angular-route.js"></script>
<script src="~/Scripts/category/categoryModule.js"></script>
<script src="~/Scripts/category/Service.js"></script>
<script src="~/Scripts/category/CategoryController.js"></script>
</div>
</div>
Run the application. Navigate to /Category... Now add Categories and delete categories. Enjoy!
