Introduction
Basically, this method is working with all controls inherited.
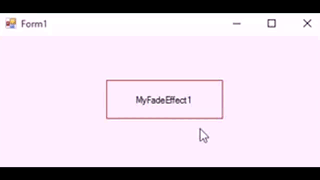
Background
It is very important to create Private Property SetImage() As Image
for fading effect.
Use the based Property
Image
only for drawing image to the control inherited.
Using the Code
Imports System.Drawing.Imaging
Public Class MyFadeEffect : Inherits Control
#Region " Private Declaration "
Private ImgAtt As New ImageAttributes
Private StartFade As Boolean, Alpha As Single = 0.0, SpeedFade As Single = 0.0
Private ImgHover As Bitmap, GrHover As Graphics, _SetImage As Image
Private _SetSpeed As SetSpeed = SetSpeed.Normal
#End Region
#Region " Enum Fade Speed "
Public Enum SetSpeed
Maximum
High
Normal
Slow
VerySlow
End Enum
#End Region
#Region " Properties "
Private Property SetImage() As Image
Get
Return _SetImage
End Get
Set(value As Image)
_SetImage = value
Invalidate()
End Set
End Property
Public Property FadingSpeed() As SetSpeed
Get
Return _SetSpeed
End Get
Set(value As SetSpeed)
_SetSpeed = value
Invalidate()
End Set
End Property
#End Region
#Region " Sub New "
Sub New()
SetStyle(ControlStyles.AllPaintingInWmPaint Or _
ControlStyles.OptimizedDoubleBuffer Or _
ControlStyles.UserPaint, True)
DoubleBuffered = True
Size = New Size(150, 50)
End Sub
#End Region
#Region " Drawing Fade Effect "
Private Sub DrawFadeEffect(G As Graphics)
If FadingSpeed = SetSpeed.VerySlow Then
SpeedFade = 0.03
ElseIf FadingSpeed = SetSpeed.Slow
SpeedFade = 0.06
ElseIf FadingSpeed = SetSpeed.Normal
SpeedFade = 0.11
ElseIf FadingSpeed = SetSpeed.High
SpeedFade = 0.21
ElseIf FadingSpeed = SetSpeed.Maximum
SpeedFade = 0.31
End If
Dim _Matrix As New ColorMatrix(New Single()() { _
New Single() {1, 0, 0, 0, 0}, _
New Single() {0, 1, 0, 0, 0}, _
New Single() {0, 0, 1, 0, 0}, _
New Single() {0, 0, 0, Alpha, 0}, _
New Single() {0, 0, 0, 0, 1}})
ImgAtt.SetColorMatrix(_Matrix, ColorMatrixFlag.Default, ColorAdjustType.Bitmap)
ImgHover = New Bitmap(Width, Height)
GrHover = Graphics.FromImage(ImgHover)
Dim _Brush As New SolidBrush(Color.FromArgb(255, Color.Blue))
GrHover.FillRectangle(_Brush, 0, 0, Width, Height)
G.DrawImage(ImgHover, New Rectangle(0, 0, ImgHover.Width, ImgHover.Height), _
0, 0, ImgHover.Width, ImgHover.Height, GraphicsUnit.Pixel, ImgAtt)
ImgHover.Dispose()
GrHover.Dispose()
End Sub
#End Region
#Region " On Paint/Background Paint Event "
Protected Overrides Sub OnPaint(e As PaintEventArgs)
MyBase.OnPaint(e)
e.Graphics.DrawRectangle(Pens.Red, 0, 0, Width - 1, Height - 1)
TextRenderer.DrawText(e.Graphics, Text, Font, ClientRectangle, ForeColor)
End Sub
Protected Overrides Sub OnPaintBackground(e As PaintEventArgs)
MyBase.OnPaintBackground(e)
DrawFadeEffect(e.Graphics)
End Sub
#End Region
#Region " On Mouse Enter/Leave Events "
Protected Overrides Sub OnMouseEnter(e As EventArgs)
MyBase.OnMouseEnter(e)
StartFade = True
TmrFade.Enabled = True
Invalidate()
End Sub
Protected Overrides Sub OnMouseLeave(e As EventArgs)
MyBase.OnMouseLeave(e)
StartFade = False
TmrFade.Enabled = True
Invalidate()
End Sub
#End Region
#Region " Timer Fade Tick "
Private Sub TmrFade_Tick(sender As Object, e As EventArgs) Handles TmrFade.Tick
If StartFade Then
Alpha += SpeedFade
Else
Alpha -= SpeedFade
End If
SetImage = ImgHover
If Alpha >= 1 Then
Alpha = 1
TmrFade.Enabled = False
ElseIf Alpha <= 0
Alpha = 0
TmrFade.Enabled = False
End If
End Sub
#End Region
Friend WithEvents TmrFade As New Timer With {.Enabled = False, .Interval = 1}
End Class
History
- 23rd October, 2015: Initial version
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.