Introduction
There could be a lot of simulators that could simulate network structures and even can build a virtual test bed to simulate Self Defined Network. But there are a few simulates which are available for building wireless networks. Miniet-wifi is a small but functional software for developers to simulate their own wireless networks with a Linux-installed laptop.
Most of the codes in mininet were written in Python language. Due to this, it might be much easier for developers to read them and modify them. And I would explain most part of the codes for you to make sure you can get a quick start when you can access to it. By the way, I have uploaded the zipped version.
Install Mininet-wifi
- Step 1: git clone https://github.com/intrig-unicamp/mininet-wifi
- Step 2: cd mininet-wifi
- Step 3: sudo ./util/install.sh -Wnfv
Code a Topology
Topology file must be written in Python form.
"""
Setting the position of Nodes (only for Stations and Access Points) and providing mobility.
"""
from mininet.net import Mininet
from mininet.node import Controller,OVSKernelSwitch
from mininet.link import TCLink
from mininet.cli import CLI
from mininet.log import setLogLevel
def topology():
"Create a network."
net = Mininet( controller=Controller, link=TCLink, switch=OVSKernelSwitch )
print "*** Creating nodes"
h1 = net.addHost( 'h1', mac='00:00:00:00:00:01', ip='10.0.0.1/8' )
sta1 = net.addStation( 'sta1', mac='00:00:00:00:00:02', ip='10.0.0.2/8' )
sta2 = net.addStation( 'sta2', mac='00:00:00:00:00:03', ip='10.0.0.3/8' )
ap1 = net.addBaseStation( 'ap1', ssid= 'new-ssid1',
mode= 'g', channel= '6', position='10,20,0' )
ap2 = net.addBaseStation( 'ap2', ssid= 'new-ssid2',
mode= 'g', channel= '6', position='10,50,0' )
ap3 = net.addBaseStation( 'ap3', ssid= 'new-ssid2',
mode= 'g', channel= '6', position='40,20,0' )
ap4 = net.addBaseStation( 'ap4', ssid= 'new-ssid2',
mode= 'g', channel= '6', position='40,50,0' )
ap5 = net.addBaseStation( 'ap5', ssid= 'new-ssid2',
mode= 'g', channel= '6', position='70,20,0' )
ap6 = net.addBaseStation( 'ap6', ssid= 'new-ssid6',
mode= 'g', channel= '6', position='70,50,0' )
c1 = net.addController( 'c1', controller=Controller )
print "*** Associating and Creating links"
net.addLink(ap1, h1)
net.addLink(ap1, sta1)
net.addLink(ap1, sta2)
net.addLink(ap1, ap2)
print "*** Starting network"
net.build()
c1.start()
ap1.start( [c1] )
"""uncomment to plot graph"""
net.plotGraph(max_x=100, max_y=100)
net.startMobility(startTime=0)
net.mobility('sta1', 'start', time=1, position='10.0,20.0,0.0')
net.mobility('sta2', 'start', time=2, position='30.0,30.0,0.0')
net.mobility('sta1', 'stop', time=12, position='70.0,0.0,0.0')
net.mobility('sta2', 'stop', time=56, position='80.0,100.0,0.0')
net.stopMobility(stopTime=23)
print "*** Running CLI"
CLI( net )
print "*** Stopping network"
net.stop()
if __name__ == '__main__':
setLogLevel( 'info' )
topology()
The Graph
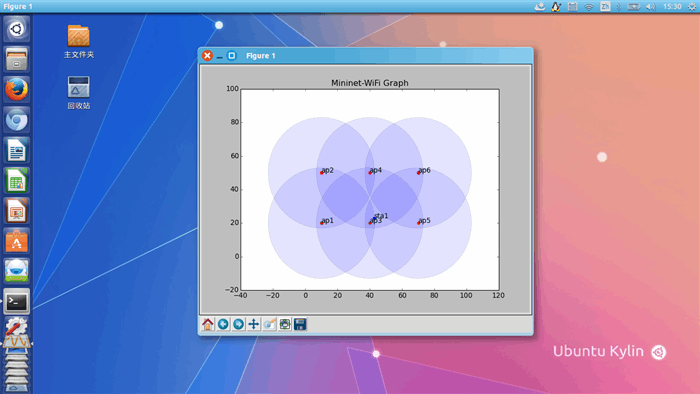
This graph shows how miminet-wifi would works. And if you have followed all the steps above, you could also see the 'sta' is roaming in the graph. And now, you can do 'Ping Test', 'IPerf', etc.
Source Code Explanation
The most essential file is wifi.py. It is in the path 'mininet-wifi/mininet/'.
File Name wifi.py
Dependence
import os
import socket
import struct
import fcntl
import fileinput
import subprocess
import glob
import math
import time
import numpy as np
import scipy.spatial.distance as distance
import matplotlib.patches as patches
import matplotlib.pyplot as plt
from mininet.mobility import gauss_markov, \
truncated_levy_walk, random_direction, random_waypoint, random_walk
Class
class checkNM ( object ) // To write mac addresses which were created for
// virtual aps into file /etc/NetworkManager/NetworkManager.conf
def checkNetworkManager(self, mac)
def getMacAddress(self, ap) //get mac address from interfaces
def APfile(self, cmd, ap)
class getWlan( object ) //get Wlan interfaces
def physical(self)
def virtual(self)
class association( object ) //set wifi parameters to network interface and associate mobile stations to aps
def setAdhocParameters(self, sta, iface) //set WIFI model
def parameters(self, sta, ap, distance, wlan) //define wifi interfaces and parameters
def setInfraParameters(self, sta, ap, distance, wlan)
def doAssociation(self, mode, ap, distance) //associate stations to aps, according to distance
class station ( object ) //denote details about moblie stations
addressingSta = {}
indexStaIface = {}
fixedPosition = []
apIface = []
printCon = True
def ifconfig(self, sta)
def iwCommand(self, sta, wlan, *args) //receive commands to assicate to aps or
// disconnect from aps
def ipLinkCommand(self, sta, wlan, *args) //link up stations to aps
def setMac(self, sta)//set macaddress for stations
def assingIface(self, stations)
def getWiFiParameters(self, sta, interface)
def confirmMeshAssociation(self, sta, interface, mpID)
class accessPoint ( object )
list = []
number = 0
exists = False
manual_apRange = -10
def range(self, mode)
def rename( self, intf, newname )
def numberOfAssociatedStations( self, ap )
class mobility ( object )
plotap = {}
plotsta = {}
plottxt = {}
nodesPlotted = []
plotGraph = False
cancelPlot = False
ismobility = False
leastLoadFirst = False
DRAW = False
MAX_X = 50
MAX_Y = 50
def move(self, sta, diffTime, speed, startposition, endposition)
def plotAccessPoint(self, ap, position, ax)
def plot(self, src, dst, pos_src, pos_dst)
def getDistance(self, src, dst)
def handover(self, sta, ap, wlan, distance, changeAP, reason=None, **params)
WenQiang Jin received his B.A in Information and Telecommunication Engineering from CQUPT, China. He is currently a Masters student in Telecommunication at CQUPT. He received network engineering certificate from China Government. His research interest is SDN, wireless network programming,Network Calculus,Network Computing,and software testing.
HE IS CONSIDERING TO APPLY A Ph.D DEGREE IN ENGLISH-SPEAKING COUNTRIES. PLEASE CONTACT HIM.