Colors Just Wanna Have Fu-un
There is a 'seminar' of sorts (not sure what it really should be called) where you learn about different personality types (your own and others) which is supposed to help you understand yourself and others better, and thus be able to work more effectively together. It is called "True Colors".
I wondered, after attending this class/session, what my "composite" color would be. I found out that "my" "primary" color is green, and my "palest" color is orange, but what about gradations in between (which we all are, made up of "a little of this color and a little of that color")?
I wanted to visualize "my" color. So I created a simple util that purports to do that.
Note: A way to accomplish this using web technologies can be seen in the accepted answer (by Michael Laszlo) here.
Let it be said first, that the colors used here (red, blue, orange, and gold) are not necessarily what a professional artist, printer, interior decorator, and so forth, would call those colors. They are the "True Colors" hues as extracted from their website using the ColorZilla browser plugin.
But, using this code, you could use the principles and practices herein to use your own custom colors (or the "official" versions of red, blue, orange, gold, etc.) to come up with different representations of coordinates on the electromagnetic spectrum.
This is only a tip, so I'm just adding the code from this point; there are a handful of comments in it, though - let them serve as the tip explanation; As Mark Twain would have said: "Let it go." And so here it is, without further ado or adon't, the code:
versions of those colors, as found on the True Colors website
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace CyndiLauper
{
public partial class FormTrueColorsMain : Form
{
const int GREEN_RED = 15;
const int GREEN_GREEN = 167;
const int GREEN_BLUE = 73;
const int BLUE_RED = 0;
const int BLUE_GREEN = 152;
const int BLUE_BLUE = 215;
const int ORANGE_RED = 243;
const int ORANGE_GREEN = 123;
const int ORANGE_BLUE = 38;
const int GOLD_RED = 255;
const int GOLD_GREEN = 230;
const int GOLD_BLUE = 101;
Color tcGreen = Color.FromArgb(15, 167, 73);
Color tcGreenComplement = Color.FromArgb(240, 88, 182);
Color tcBlue = Color.FromArgb(0, 152, 215);
Color tcOrange = Color.FromArgb(243, 123, 38);
Color tcGold = Color.FromArgb(255, 230, 101);
public FormTrueColorsMain()
{
InitializeComponent();
InitializeControls();
}
private void InitializeControls()
{
lblGreen.ForeColor = tcGreen;
lblGreen.BackColor = Color.White;
lblBlue.ForeColor = tcBlue;
lblBlue.BackColor = Color.White;
lblOrange.ForeColor = tcOrange;
lblOrange.BackColor = Color.Black;
lblGold.ForeColor = tcGold;
lblGold.BackColor = Color.Black;
progressBarGreen.ForeColor = tcGreen;
progressBarGreen.Style = System.Windows.Forms.ProgressBarStyle.Continuous;
progressBarBlue.ForeColor = tcBlue;
progressBarBlue.Style = System.Windows.Forms.ProgressBarStyle.Continuous;
progressBarOrange.ForeColor = tcOrange;
progressBarOrange.Style = System.Windows.Forms.ProgressBarStyle.Continuous;
progressBarGold.ForeColor = tcGold;
progressBarGold.Style = System.Windows.Forms.ProgressBarStyle.Continuous;
}
private void button1_Click(object sender, EventArgs e)
{
SetGreenProgressBar();
SetBlueProgressBar();
SetOrangeProgressBar();
SetGoldProgressBar();
int greenVal = (int)numericUpDownGreen.Value;
int blueVal = (int)numericUpDownBlue.Value;
int orangeVal = (int)numericUpDownOrange.Value;
int goldVal = (int)numericUpDownGold.Value;
Color trueColor = GetTrueCombinedColor(greenVal, blueVal, orangeVal, goldVal);
pictureBoxTCCombo.BackColor = trueColor;
}
private Color GetTrueCombinedColor(int greenVal, int blueVal, int orangeVal, int goldVal)
{
int redTotal = ((greenVal * GREEN_RED) +
(orangeVal * ORANGE_RED) + (goldVal * GOLD_RED));
int greenTotal = ((greenVal * GREEN_GREEN) +
(blueVal * BLUE_GREEN) + (orangeVal * ORANGE_GREEN) + (goldVal * GOLD_GREEN));
int blueTotal = ((greenVal * GREEN_BLUE) +
(blueVal * BLUE_BLUE) + (orangeVal * ORANGE_BLUE) + (goldVal * GOLD_BLUE));
int redWeight = redTotal / 50;
int greenWeight = greenTotal / 50;
int blueWeight = blueTotal / 50;
if (redWeight <= 0) redWeight = 1;
if (greenWeight <= 0) greenWeight = 1;
if (blueWeight <= 0) blueWeight = 1;
if (redWeight >= 256) redWeight = 255;
if (greenWeight >= 256) greenWeight = 255;
if (blueWeight >= 256) blueWeight = 255;
Color trueColorCombo = Color.FromArgb(redWeight, greenWeight, blueWeight);
return trueColorCombo;
}
private void numericUpDownGreen_ValueChanged(object sender, EventArgs e)
{
SetGreenProgressBar();
}
private void SetGreenProgressBar()
{
progressBarGreen.Value = (int)numericUpDownGreen.Value;
}
private void numericUpDownBlue_ValueChanged(object sender, EventArgs e)
{
SetBlueProgressBar();
}
private void SetBlueProgressBar()
{
progressBarBlue.Value = (int)numericUpDownBlue.Value;
}
private void numericUpDownOrange_ValueChanged(object sender, EventArgs e)
{
SetOrangeProgressBar();
}
private void SetOrangeProgressBar()
{
progressBarOrange.Value = (int)numericUpDownOrange.Value;
}
private void numericUpDownGold_ValueChanged(object sender, EventArgs e)
{
SetGoldProgressBar();
}
private void SetGoldProgressBar()
{
progressBarGold.Value = (int)numericUpDownGold.Value;
}
}
}
And here's what it looks like, in action (inaction?):
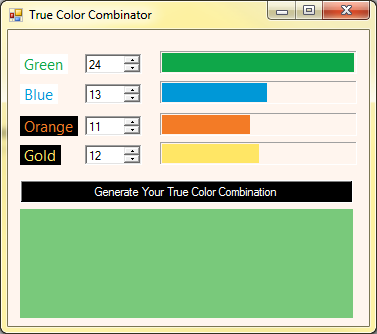
Time After Time
Let your True Colors shine through by sending me a Persian Rug made up of the True Colors of yourself, your family, your workmates, and your local police dog.
I am in the process of morphing from a software developer into a portrayer of Mark Twain. My monologue (or one-man play, entitled "The Adventures of Mark Twain: As Told By Himself" and set in 1896) features Twain giving an overview of his life up till then. The performance includes the relating of interesting experiences and humorous anecdotes from Twain's boyhood and youth, his time as a riverboat pilot, his wild and woolly adventures in the Territory of Nevada and California, and experiences as a writer and world traveler, including recollections of meetings with many of the famous and powerful of the 19th century - royalty, business magnates, fellow authors, as well as intimate glimpses into his home life (his parents, siblings, wife, and children).
Peripatetic and picaresque, I have lived in eight states; specifically, besides my native California (where I was born and where I now again reside) in chronological order: New York, Montana, Alaska, Oklahoma, Wisconsin, Idaho, and Missouri.
I am also a writer of both fiction (for which I use a nom de plume, "Blackbird Crow Raven", as a nod to my Native American heritage - I am "½ Cowboy, ½ Indian") and nonfiction, including a two-volume social and cultural history of the U.S. which covers important events from 1620-2006: http://www.lulu.com/spotlight/blackbirdcraven