Multiple selections and changing selected row color
Gridview provides built in support for single row selection. Selecting multiple rows from the Gridview control is not difficult at all, however it will be nice if we can change the background Color of the selected row and can easily identify multiple selected rows. To implement this idea I searched on the internet but unfortunately couldn’t find any easy and optimal way to accomplish the task. There can be many solutions to implement the same idea however I believe the approach I am using is very easy and efficient.
The HTML below shows a Gridview control with Data from Northwind Employees table.
<asp:GridView ID="DataGV" runat="server" AutoGenerateColumns="False" cellpadding="4"
GridLines="Horizontal" BackColor="White" BorderColor="#336666" BorderStyle="Double"
BorderWidth="3px" Font-Bold="True" Font-Names="Verdana" Font-Size="X-Small" Height="1px"
Width="100%" AllowSorting="True" HorizontalAlign="Center" DataKeyNames="EmployeeID"
DataSourceID="SqlDataSource1" AllowPaging="True" PageSize="50">
<RowStyle BackColor="White" ForeColor="#333333" />
<Columns>
<asp:TemplateField HeaderText="Select">
<ItemTemplate>
<asp:CheckBox ID="CheckBox1" runat="server"/>
<asp:Label ID="LBLEmpID" runat="server" Text='<%# container.dataitem("EmployeeID") %>'
Visible="false"></asp:Label>
</ItemTemplate>
<HeaderStyle HorizontalAlign="Left" />
<FooterStyle HorizontalAlign="Left" />
</asp:TemplateField>
<asp:TemplateField HeaderText="Name" SortExpression="LastName">
<HeaderStyle HorizontalAlign="Left" />
<ItemTemplate>
<asp:Label ID="LblName" runat="server"
Text='<%# trim(Eval ("LastName")) + ", " + trim( Eval ("FirstName"))%>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="Title" HeaderText="Title" SortExpression="Title" >
<HeaderStyle HorizontalAlign="Left" />
</asp:BoundField>
<asp:BoundField DataField="City" HeaderText="City" SortExpression="City" >
<HeaderStyle HorizontalAlign="Left" />
</asp:BoundField>
<asp:TemplateField HeaderText="HireDate" SortExpression="HireDate">
<ItemStyle HorizontalAlign="Right" />
<HeaderStyle HorizontalAlign="Right" />
<ItemTemplate>
<asp:Label ID="LblHireDate" runat="server" Text='<%# Bind("HireDate",
"{0:d}") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
</Columns>
<HeaderStyle BackColor="White" Font-Bold="True"
ForeColor="Black" Font-Size="Small" VerticalAlign="Top"/>
<AlternatingRowStyle BackColor="#D6E3F7" />
<FooterStyle BackColor="#009900" Font-Bold="True"
ForeColor="White" Font-Size="Small"
HorizontalAlign="Right" />
<PagerSettings PageButtonCount="30" Mode="NumericFirstLast"
NextPageText="" PreviousPageText="" Position="TopAndBottom" />
<PagerStyle BackColor="Silver" ForeColor="White" HorizontalAlign="Center"
Font-Names="Verdana" Font-Size="Small" />
</asp:GridView>
Now all you need to do is to paste these 5 lines of code in <code>RowDataBound
event handler of your Gridview control.
If e.Row.RowType = DataControlRowType.DataRow Then
Dim Chk As CheckBox = e.Row.FindControl("CheckBox1")
Page.ClientScript.RegisterStartupScript(Me.GetType(), "MyScript", " function
HighlightSelected(colorcheckboxselected, RowState) { if (colorcheckboxselected.checked) colorcheckboxselected.parentElement.parentElement.style.backgroundColor='#FFAA63'; else { if (RowState=='0') colorcheckboxselected.parentElement.parentElement.style.backgroundColor='white'; else colorcheckboxselected.parentElement.parentElement.style.backgroundColor='#D6E3F7'; } }", True)
Chk.Attributes.Add("onclick", "HighlightSelected(this,'" + Convert.ToString(e.Row.RowState) + "' );")
End If
The code also accounts for Alternating Row. If you are not using Alternating Row in your Gridview, you can easily modify the code accordingly.
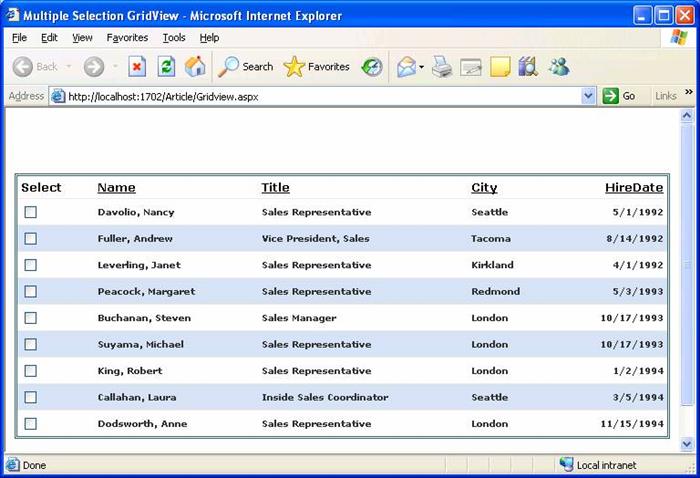

Multi column sort
Gridview provides built in support for single column sorting. In some situations multiple column sort can be very helpful for the users e.g. sorting employees by Hire Date and then by Last name or City name. Again implementing multiple columns sort is quite simple task.

I implemented multiple sort by Checkboxlist, Label and button controls. The label displays the sorting criteria user selects by checking/unchecking Checkboxes, and Click event handler of button implements the multiple sort. I wrote the following code in button’s click event handler.
If ViewState("Direction") = SortDirection.Ascending Then
ViewState("Direction") = SortDirection.Descending
Else
ViewState("Direction") = SortDirection.Ascending
End If
DataGV.Sort(SortingLBL.Text, ViewState("Direction"))
I also wanted to show the sort direction in which the columns were sorted so I added the following code in Grridview Sorting event handler to change the text/value property of the button.
If ViewState("Direction") Is Nothing Then
ElseIf ViewState("Direction") = SortDirection.Ascending Then
MultipleSortBtn.Value = "Sort Descending"
Else
MultipleSortBtn.Value = "Sort Ascending"
End If
I work for Hertz Corporation as System Developer /Analyst and have exposure to various development environments, web applications, databases, along with information and project management techniques.