Table of Contents
1.0 Introduction
Gone are the days of fiddling with the system clock to simulate the passing of time. The Time Shift Utility helps to alter the application time without affecting the System time. This document gives the complete description to use the Time Shift WCF Service to achieve this. It has a built-in mechanism which abstracts the system time away from the underlying real (database system) time. By default, the System time is the same as the database time which is being used by the application. The database time can be moved forward and backward relative to real time using this utility, without even affecting the System time. So this utility enables easy transition from one time to another.
This utility can be of great use in projects where requirements include moving the application time back and forth. This can be easily achieved without even fiddling with the system clock.
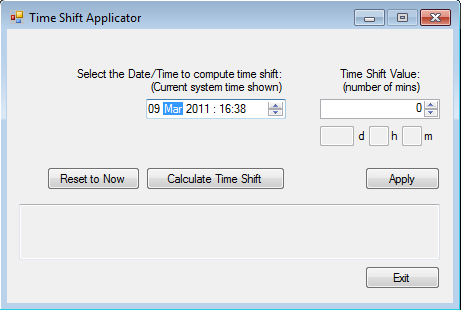
2.0 Time Shift Utility
It has a built-in mechanism to abstract the system time away from the underlying real (database system clock) time which is stored in the offset. By default, when system time is the same as the database time, the offset is zero. To move the application time (which uses the offset value from the database) forward relative to real time, the offset must be increased, and vice-versa to move the time backward relative to the real time.
The Features and Utility Flow
2.0.1 Features
The WCF Service performs the following operations to achieve alter the application time:
- Fetches the current offset value from the database
- Calculates the new offset value with respect to the current date time and the user specified date time
- Updates the offset value in the database
2.0.2 Utility Flow
A WCF Service named TimeOffsetService
enables the user to fetch or update the offset value in the database. To achieve this, the service calls the Business Logic layer TimeOffsetBusinessLogic
, which in turn calls the Data Access layer TimeOffsetDataAccess
. The Data Access layer interacts with the database to fetch or update the offset value.

Fetch Offset Value
The Data Access layer interacts with the database to fetch the current offset value. The offset value is then sent to the Business Logic layer which in turn sends it to the WCF Service.
Calculate New Offset Value
The service specifies the ‘Current date time’ of the application and the ‘User specified date time’ to the Business Logic layer. The calculation of the offset is done by the Business Logic layer. To move the application time forward relative to real time, the offset will be increased, and vice-versa to move the time backward relative to the real time.
Update Offset Value
The Service specifies the new Offset value to the Business Logic Layer, which calls the Data Access layer to update the Offset value to the database. The Data Access Layer after successfully updating the offset value sends confirmation back to the calling layers.
3.0 Implementing the Time Offset Utility
The Time Offset utility can be incorporated in your application through various ways. In this utility, there are three layers:
- Service Layer
- Business Logic Layer
- Data Access Layer
As per the requirement, you can call either the Service Layer or the Business Logic Layer or the Data Access Layer to alter the application time relative to the system time.
Time Offset Utility – Windows Application Example
A method to incorporate the utility with your application has also been shown along with the utility. A Windows based application ‘Time Shift Applicator’ fetching and updating the time offset value has been shown as an example to use this utility.
Show Current Date Time
By default, the Time Shift Applicator shows the System date/time and the time shift value at this point is 0 (the application time is the same as the System time).

Change the Application Time
i. Calculate the New Time Shift
Select the new date and time to which you want to change the application time to, and click ‘Calculate Time Shift’. This will calculate the time shift value for the selected date/time.
- If the application time is the same as the System time, the new time shift value will be calculated relative to the System date and time.
- If the application time moves forward relative to the current time, the offset will be increased, and vice-versa to move the time backward relative to the current time.

Example: If the System date/time is 01 April 2011 18:56, and the new date/time is 01 April 2011 19:00, the time shift value calculated will be 1443.
ii. Apply New Time Shift
Once the time shift value is calculated based on the new date and time, click the Apply button. This will update the offset value in the database. And the next time the application time is fetched, it will show this new value of date and time. So changing the application time without altering the System time becomes an easy transition by using this utility.

Reset the Application Time
The application time can be moved back to the original time. Click ‘Reset to Now’ and it will set the offset value to 0. Click the Apply button to update the offset value to the database. The application will show the current date time now.

4.0 Time Offset Utility Code Snippet
Time Offset Service
The time offset service performs the following operations:
- Fetch time shift
- Calculate new time shift
- Update time shift

internal partial class SystemTimeServiceImpl : ISystemTime
{
private TimeOffsetBusinessLogic.TimeOffsetLogic logic;
public SystemTimeServiceImpl()
{
logic = new TimeOffsetBusinessLogic.TimeOffsetLogic();
}
public SystemTime.TimeOffsetContractGroup.Response
UpdateOffsetValue(System.Int32 timeShitfValue)
{
TimeOffsetEntities.Response responseLogic =
logic.UpdateTimeShift(timeShitfValue);
SystemTime.TimeOffsetContractGroup.Response response = new Response();
response.result = responseLogic.Result;
return response;
}
public SystemTime.TimeOffsetContractGroup.Response
CalculateTimeShift(SystemTime.TimeOffsetContractGroup.Request request)
{
TimeOffsetEntities.Response responseLogic =
logic.CalculateTimeShift(request.userDateTime, request.currentDateTime);
SystemTime.TimeOffsetContractGroup.Response response = new Response();
response.value = Convert.ToInt32(responseLogic.Value);
response.result = responseLogic.Result;
return response;
}
public SystemTime.TimeOffsetContractGroup.Response GetSystemTimeShift()
{
TimeOffsetEntities.Response response = logic.GetSystemTimeShift();
SystemTime.TimeOffsetContractGroup.Response result = new Response();
result.value = Convert.ToInt32(response.Value);
return result;
}
}
Time Offset Business Logic Layer
The Time Offset Business Logic Layer performs the following operations:
- Fetch time shift
- Calculate new time shift
- Update time shift

public class TimeOffsetLogic
{
public TimeOffsetEntities.Response GetSystemTimeShift()
{
return TimeOffsetDataAccess.TimeOffsetDataClient.GetSystemTimeShift();
}
public TimeOffsetEntities.Response UpdateTimeShift(decimal value)
{
return TimeOffsetDataAccess.TimeOffsetDataClient.UpdateTimeShift(value);
}
public TimeOffsetEntities.Response CalculateTimeShift(DateTime userDateTime,
DateTime currentTime)
{
bool negativeShift = false;
TimeSpan timeShift;
TimeOffsetEntities.Response response = new TimeOffsetEntities.Response();
if (DateTime.Compare(userDateTime, currentTime) > 0)
{
timeShift = userDateTime.Subtract(currentTime);
}
else
{
timeShift = userDateTime.Subtract(currentTime);
negativeShift = true;
}
response.Value = Convert.ToDecimal(timeShift.TotalMinutes);
response.Result = negativeShift;
return response;
}
}
Time Offset Data Access Layer
The Time Offset Data Access Layer performs the following operations:
- Fetch time shift from the database
- Update the time shift value to the database
public static class TimeOffsetDataClient
{
private const String DATABASE_KEY = "FoundationConnectionString";
public static TimeOffsetEntities.Response GetSystemTimeShift()
{
decimal result = 0;
TimeOffsetEntities.Response response = new TimeOffsetEntities.Response();
String connStr =
ConfigurationManager.ConnectionStrings[DATABASE_KEY].ConnectionString;
using (FoundationDataContext db = new FoundationDataContext(connStr))
{
var value = from s in db.ReferenceDatas
select new TimeOffsetEntities.Response
{
Value = s.Value.Value
};
result = value.First().Value;
response.Value = result;
}
return response;
}
public static TimeOffsetEntities.Response UpdateTimeShift(decimal value)
{
TimeOffsetEntities.Response response = new TimeOffsetEntities.Response();
String connStr =
ConfigurationManager.ConnectionStrings[DATABASE_KEY].ConnectionString;
using (FoundationDataContext db = new FoundationDataContext(connStr))
{
var result = db.ReferenceDatas.Single(v => v.ReferenceDataID == 1);
result.Value = value;
db.SubmitChanges();
}
response.Result = true;
return response;
}
}
The complete code for the WCF Service, Windows application, and the database script is available for download from the link above. Refer to Steps to run Utility (inside the Zip file) to start using the utility.
5.0 Conclusion
The above specified code helps a developer to easily alter the application time without fiddling with the System time. One caution point to remember while moving the application time backwards, relative to the System time, is that if your application data heavily relies on time, be careful while moving the time backwards, as it may lead to data corruption in the database.
This utility can be of great use in projects where requirements include moving the application time back and forth. This utility enables easy transition from application time to System time and vice versa.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.