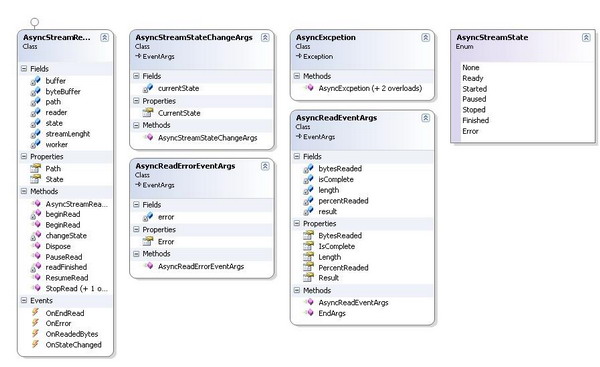
Introduction
This piece of code does async stream reading enabling you to use a progress bar in your application. On every byte read, it fires an event that contains the percentage of current read progress.
This is just an idea of how to make an async reader, so if you have any suggestions on how make it better or faster, let me now.
Using the code
The following are the AsyncStreamReader
events:
EventHandler<AsyncReadEventArgs> OnReadedBytes
- Occurs when the byte is read from the System.IO.StreamReader
, and contains the percentage of total read bytes.EventHandler<AsyncReadEventArgs> OnEndRead
- Occurs when all bytes are read from the System.IO.StreamReader
and contains the byte array of the read content.EventHandler<AsyncReadErrorEventArgs> OnError
- Occurs when an AsyncStream.AsyncExcpetion
happens and contains the exception.EventHandler<AsyncStreamStateChangeArgs> OnStateChanged
- Occurs when the state of AsyncStream.AsyncStreamReader
changes and contains the current state.
The following are the AsyncStreamReader public properties:
AsyncStreamState State
- Specifies the identifiers to indicate the the state of AsyncStream.AsyncStreamReader
.string Path
- Gets the complete file path to be read.
The following are the AsyncStreamReader
pubic methods:
BeginRead()
- Begins an asynchronous read operation and sets the state to Started
.StopRead()
- Stops an asynchronous read operation and sets the state to Stopped
.PauseRead()
- Pauses an asynchronous read operation and sets the state to Paused
.ResumeRead()
- Resumes a paused asynchronous read operation and sets the state back to Started
.


This is how we create a new asynchronous stream:
AsyncStreamReader reader = new AsyncStreamReader(fileName);
reader.OnReadedBytes += new EventHandler<AsyncReadEventArgs>(reader_OnReadedBytes);
reader.OnEndRead += new EventHandler<AsyncReadEventArgs>(reader_OnEndRead);
reader.OnStateChanged +=
new EventHandler<AsyncStreamStateChangeArgs>(reader_OnStateChanged);
reader.OnError += new EventHandler<AsyncReadErrorEventArgs>(reader_OnError);
reader.BeginRead();
Here is the OnReadedBytes
event:
void reader_OnReadedBytes(object sender, AsyncReadEventArgs e)
{
int percents = e.PercentReaded;
bool isComplete = e.IsComplete;
long bytesReaded = e.BytesReaded;
long length = e.Length;
byte[] bytes = e.Result;
}
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.