Introduction
The design pattern can be described as solving some piece of problem in a wise manner. We can solve an entire class of similar problems using a specific design pattern. There are three basic categories of design patterns, namely: Creational, Structural and Behavioral.
In this article, I have tried to explain one of the important creational design patterns, i.e., Abstract Factory Pattern, with a simple example. Creational pattern deals with how an object can be created. This means that the object creation process will be isolated from the details of its creation, and as a result, we don’t have to change the code whenever a new type of product is to be created.
Abstract Factory method “provides an interface for creating families of related or dependent objects without specifying their concrete classes”. Abstract Factory pattern can be used when a system should be independent of how its products are created.
The main participants of the Abstract Factory are:
- Abstract Factory: In the example, the class
CShapeFactory
is the abstract factory. This class just exposes an interface to be realized in the concrete factory classes.
- Concrete Factory: Implements the operations to create concrete product objects. In the example,
CNormalShapeFactory
and CEnhancedShapeFactory
are working as two concrete factories.
- Product: Here,
CMyLine
and CMyTriangle
, CMyDashedLine
and CMyFilledTriangle
are the classes to produce these products.
- Client: Uses the interface exposed by the Abstract Factory class. Here,
canvass
is working as the client class.
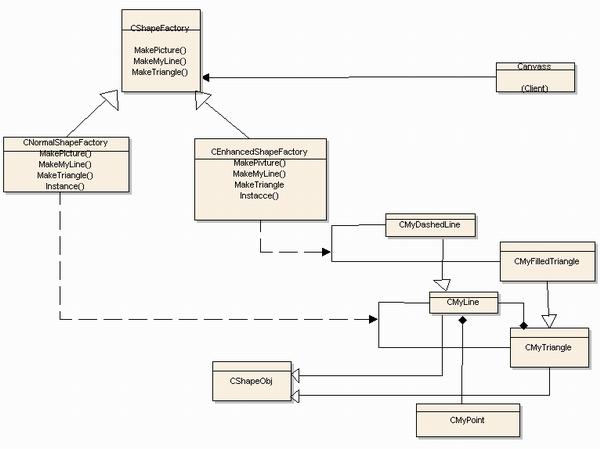
Fig: Class diagram of the example code
The concrete factory classes are usually implemented as Singleton. If we look at the example, we will find how CNormalShapefactory
and CEnhancedShapefactory
have been implemented as Singletons. Look at the following implementation of the CNormalShapefactory ::Instance
function. It returns a pointer to the one and only one instance. And the client code always accesses this instance through CNormalShapeFactory ::Instance()
function. The same thing is happening for the CEnhancedShapeFactory
class.
static CNormalShapeFactory* Instance()
{
if(NormalShapeFactoryInstance == 0)
NormalShapeFactoryInstance = new CNormalShapeFactory;
return NormalShapeFactoryInstance;
}
private:
static NormalShapeFactory* NormalShapeFactoryInstance;
In the example, the first concrete class CNormalShapeFactory
is creating a picture made up with solid lines and a triangle made up with those solid lines; whereas the second concrete class CEnhancedShapeFactory
is creating a picture with dashed lines and a filled triangle.
Note: To run the example in MSVC environment, please enable the RTTI option in the project settings.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.