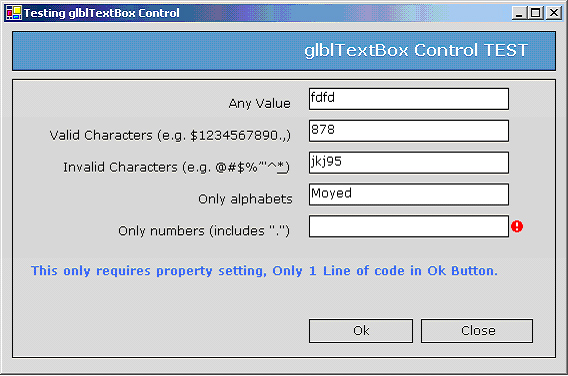
Introduction
glblControl Textbox
The use of this control eliminates various common validation issues in a form. Properties available with this control are:
Required
: Sets the field as required for validation. Required textbox’s background color will be changed to Old Lace.
ShowErrorIcon
: It is either True
or False
, it shows a flashing icon. If the required property is set to true and the field is left blank, it will show a red flashing icon. If invalid value is entered, it will flash a yellowish icon.
ValidationMode
: Sets the validation mode to use. They are the following:
- None
- Any value
- Valid characters e.g. “$1234567890.,”
- Invalid characters e.g. “@#$%’”^&*”
- Letters
- Only alphabets (includes “ , ; . ”)
- Numbers
- Only numbers (includes “.”)
Textbox
(valid/invalid sharacters): Sets this property with valid/invalid characters as per the ValidationMode
property.
Events handled
TextChanged
KeyPress
Validating
Validated
Enter
Leave
Limitations: It can check for the form level required property for only those controls where Container level is less than or equal to 4. I.e., if the textbox is in Panel4, under Panel3, under Panel2, under Panel1 under FORM
, the form level required property will not be checked when exiting form without any input in the textbox.
Pointing to a yellowish or red error icon will show you tips as to what has gone wrong. The only required code in the form is as follows:
On the OK button:
If glblControls.TextBox.requiredFieldsCheck(Me) = True Then
MsgBox("OK!", MsgBoxStyle.Information, Me.Text)
Me.Close()
Else
MsgBox("There are required fields in the form!",
MsgBoxStyle.Exclamation, Me.Text)
End If
On the Cancel button:
glblControls.TextBox.cancelrequiredFieldsCheck(Me)
Me.Close()
glblControl Masked Textbox
This is a very easy to use Masked Textbox for fixed length strings like phone numbers, social security, driver license, etc.
- The "#" character in the mask is used for digit only.
- The "&" character accepts letters only.
- The "!" character accepts a letter or digit.
- You can use any mask symbol, letter and digit other than # & and !.
E.g. ###-&!/&&(###) ###-!!!! &&XXX##Z12&&&
Using the component
Set the mask property with any combination of characters. At run time, you can read the unformatted Text
property to read only the user input. The Text
property returns the formatted string.

This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.