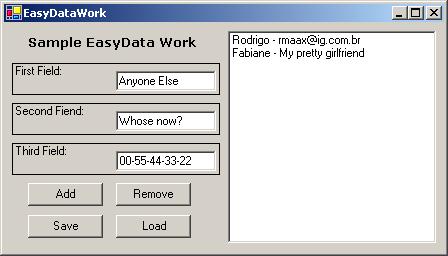
Introduction
I'll try to explain (if my poor English permits) the way I currently work with data, specially when I need to do this as fast as possible: design-create-fill-save-and-load data. I found this a good way if you don't have time or you don't want to work with SQL Server or Access databases. Into the article, I'll explain some interesting topics we'll need to accomplish the goal, how to create what we can call a DataClass, the serializing-n-deserializing tool (it comes with VB!), and a tricky tip on array serializing.. With this, we can make the EasyData work.
Background
Some time ago, I came here to find some code on XML saving and I had some problem trying to serialize my classes. I soon found that the XML serializer does not work with an ArrayList
. What to do now? I wanted to save my data without complication and into some pattern that would be easy to be "remembered" (that's why I chose XML), but I didn't want to lose the great facilities that the ArrayList
s give when you're a beginner to the data world. So that's the beginning of EasyData.
Using the code
Let's start from the beginning. The first pass is to create an easy and fast way to work with data. I found ArrayList
very useful and simple to do this. Let's see the two classes:
Public Class EasyData
Private Table As New ArrayList
End Class
Public Class SingleData
Public FirstInfo As String
Public SecondInfo As String
Public ThirdInfo As String
End Class
Explaining: the SingleData
class is where we actually insert the data into. The EasyData
class is where the whole fun is. As Table
is an ArrayList
, it's easy to add and remove objects from it. No connections needed, no need of start/end reading. The result is that it became an unusual way to manage large sets of data. (Well, if you're thinking of 1000 K lines, consider SQL or Access!)
Public Function Item(ByVal x As Short) As SingleData
Return Table.Item(x)
End Function
Public Function Count() As Short
Return Table.Count
End Function
Public Sub Add(ByVal SD As SingleData)
Table.Add(SD)
End Sub
Public Sub Remove(ByVal SD As SingleData)
Table.Remove(SD)
End Sub
I found that the functions Add
, Remove
, Count
and Item
are enough to manage the data.
Save Trick
So we made one table and we can work with the data. And now? How to save the information?
This was my question when I came here and I found some interesting articles on how to serialize classes in VB.NET and an overall view in "XML Data Files, XML Serialization, and .NET". Then to the classic Ctrl+C and Ctrl+V. And... it didn't work. ArrayList
has an advantage, you don't need to size the array and you don't need to bother about the way data is stored inside it. It is great for beginners or for a fast data application, but as it "doesn't have" a size neither an internal order, we cannot serialize it. Then I got some simple idea. Well, when I need to work with data, I'll use ArrayList
, and while saving, I'll use a normal vector and then make one XML file during serialization.
In order to maintain some learning aspect, I've separated the functions of saving/loading and converting, into one class: FileFunction
. Here is the main aspect of the program:

Picture 2 - An overview of the saving process (the Load is analog).
Function ArrayToList(ByVal Table As ArrayList) As SingleData()
Dim i As Short
Dim p(Table.Count) As SingleData
For i = 0 To ed.Count - 1
p(i) = ed.Item(i)
Next
Return p
End Function
Here we have the "implemented functions" shown on Picture 2. The function is simple, get one ArrayList
and create a SingleData
vector p
with Table.Count
elements. Then all we need is to fulfill the vector with the data from the Array
.
Public Sub Save(ByVal filename As String, ByVal ED As ArrayList)
Dim SD() As SingleData
SD = ArrayToList(ED)
Dim serializer As New XmlSerializer(SD.GetType)
Dim ns As New XmlSerializerNamespaces
Dim fs As New FileStream(filename, FileMode.Create)
Dim writer As New XmlTextWriter(fs, New UTF8Encoding)
serializer.Serialize(writer, SD, ns)
writer.Close()
End Sub
Here is the save process. Its' simple. If you have any doubt, may be a first look on "XML Data Files, XML Serialization, and .NET" may help a lot. But the main work is simple. The function gets the Table-ArrayList
and uses the function ArraytoList
so create an exact copy but in a Vector
form, so no more trouble. We create one object responsible to serialize and a writer that is the responsible for creating the XML archive itself.
The load process is identical to the saving one, I think I don't need to explain because it is really simple and is commented on the source code.
Demo project data work
In the demo, I made a simple way to work with the data. The rule here is to be simple. I'd put some textboxes to input the data and one listbox to visualize the data. The other four buttons are just Add, Delete, Save and Load.
Points of Interest
Some may see this code as completely useless, I may agree with you but it really saves a lot of time, and after all, I learned a lot from it! That's the fun!
Lots of improvements are needed. Maybe the first thing would be velocity, maybe changing some functions. As soon as I get something new, I'll edit this topic.
If any of make some improvements to this, send an e-mail to me rmaax@ig.com.br. I'll be glad to see someone out there like this crazy thing too.
Oh and as it is, it's almost ready to use as one phones or emails data.
History
First version online.
Control and Automation Student.
Working with VB.Net in the past 2 years.
Loves microeletronic and AI.