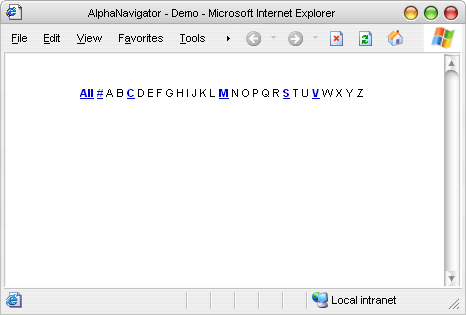
Introduction
I was asked to create a hotmail style (contacts section) alpha navigation strip. Seeing an opportunity for reuse I created a control instead of just a straight code. This control is in beta mode, as I’ll be adding more to its robustness, but I want to say that the current state of the control is completely stable and usable. This control takes a couple of variables, specifically the DB table and the column from which it will be compose its list of letters with links.
Using the code
The following is an example of the code needed to use the control. First reference the AlphaNavigator.dll, then add it to your Toolbox. Next drag a new instance onto the page and in the properties/events panel set the name for the LetterClick
event.
protected AlphaNavigator.AlphaNavigator AlphaNavigator1;
private void Page_Load(object sender, System.EventArgs e){
this.AlphaNavigator1.LetterClick +=
new System.EventHandler(this.AlphaNavigator1_LetterClick);
string sSql = "SELECT DISTINCT SUBSTRING(Column,1,1) " +
"FROM Table ORDER BY SUBSTRING(Column,1,1) ";
OdbcConnection sqlConn = null;
sqlConn = new OdbcConnection("Driver={MySQL ODBC 3.51 Driver};" +
"dsn=DNSNAME;database=DBNAME;uid=USER;password=PASS;");
sqlConn.Open();
OdbcCommand sqlCmd;
OdbcDataReader myReader;
sqlCmd = new OdbcCommand(sSql,sqlConn);
sqlCmd.CommandTimeout = 10;
myReader = sqlCmd.ExecuteReader();
AlphaNavigator1.DataSource = myReader;
}
private void AlphaNavigator1_LetterClick(object sender,System.EventArgs e){
Response.Write("Selected Letter:" + AlphaNavigator1.SelectedLetter);
}
AlphaNavigator control
This is the main code of the control. The Render
method is overridden. The code simply queries the DB and loops through the rows while adding to an array the items that exist. Once this is complete the GUI is drawn:
protected override void Render(System.Web.UI.HtmlTextWriter writer) {
StringDictionary abc = AlphaLinks();
string alphabet = "#ABCDEFGHIJKLMNOPQRSTUVWXYZ";
if (this.SelectedLetter != ""){
System.Web.UI.HtmlControls.HtmlAnchor hypLink =
new System.Web.UI.HtmlControls.HtmlAnchor();
hypLink.HRef =
"javascript:" + this.Page.GetPostBackEventReference(this,
"LetterClick");
hypLink.Name = this.UniqueID;
hypLink.InnerHtml = "All";
Controls.Add(hypLink);
} else {
Label lblAll = new Label();
lblAll.Text = "All";
Controls.Add(lblAll);
}
foreach (char C in alphabet){
Label lblSpace = new Label();
lblSpace.Text = " ";
Controls.Add(lblSpace);
string sCurrentChar = C.ToString();
if ((abc[sCurrentChar] == "on") && (this.SelectedLetter != sCurrentChar)){
System.Web.UI.HtmlControls.HtmlAnchor hypLink =
new System.Web.UI.HtmlControls.HtmlAnchor();
hypLink.HRef ="javascript:" + this.Page.GetPostBackEventReference(this,
"LetterClick" + sCurrentChar);
hypLink.Name = this.UniqueID + "_" + sCurrentChar;
hypLink.InnerHtml = "" + sCurrentChar + "";
Controls.Add(hypLink);
} else {
Label lblLetter = new Label();
lblLetter.Text = sCurrentChar;
Controls.Add(lblLetter);
}
}
base.Render(writer);
}
private StringDictionary AlphaLinks(){
StringDictionary abc = new StringDictionary();
if (_dataSource != null){
if (_dataSource is DataView) {
DataView dv = (DataView)_dataSource;
foreach(DataRow dr in dv.Table.Rows){
string letter = dr[0].ToString().ToUpper();
if (Char.IsDigit(letter[0]))
abc["#"] = "on";
else
abc[letter] = "on";
}
}else if (_dataSource is IDataReader) {
IDataReader reader = (IDataReader)_dataSource;
while (reader.Read()) {
string letter = reader[0].ToString().ToUpper();
if (Char.IsDigit(letter[0]))
abc["#"] = "on";
else
abc[letter] = "on";
}
}
}
abc["All"] = "on";
return abc;
}
Future plans
More robustness.
History
- Version 1.0, May 2005.
- Version 1.1, May 2005 - Rewritten, now uses
DataSource
to build self.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.