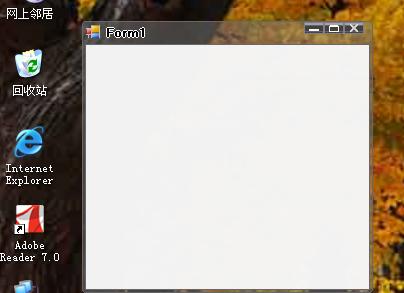
Introduction
This is article about how to make a transparent form in VS2005 like a Vista form. When I saw the Vista form the very first time, I started thinking about how to make a form like that. I searched a lot of web sites for a good solution, but didn't find an answer. Some guys told me to make a form using three different forms, but I think that is a crazy idea. We could do that, but I don't know how we cold then use it in a dialog model. I tried several ways to implement this form, and the solution I provide here is a good and simple one. I'm not really making the form transparent. The code follows. The most important part is the Graphics.CopyFromScreen
method.
Source Code
e.Graphics.CopyFromScreen(this.PointToScreen(new Point(0, 0)),
new Point(0, 0), new Size(this.Width, 23));
e.Graphics.CopyFromScreen(this.PointToScreen(new Point(0, 0)),
new Point(0, 0), new Size(3, this.Height));
e.Graphics.CopyFromScreen(this.PointToScreen(new Point(this.Width - 4, 0)),
new Point(this.Width - 4, 0), new Size(3, this.Height));
e.Graphics.CopyFromScreen(this.PointToScreen(new Point(0,
this.Height - 4)), new Point(0, this.Height - 4),
new Size(this.Width, 3));
Key Point
public GlassForm()
{
InitializeComponent();
base.Padding = new Padding(4, 24, 5, 4);
base.Opacity = 0.99;
this.FormBorderStyle = FormBorderStyle.None;
SetStyle(ControlStyles.UserPaint, true);
SetStyle(ControlStyles.AllPaintingInWmPaint, true);
SetStyle(ControlStyles.OptimizedDoubleBuffer, true);
SetStyle(ControlStyles.SupportsTransparentBackColor, true);
SetStyle(ControlStyles.ResizeRedraw, true);
}
public new double Opacity
{
get
{
return 1;
}
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
e.Graphics.Clear(System.Drawing.Color.White);
e.Graphics.CopyFromScreen(this.PointToScreen(new Point(0, 0)),
new Point(0, 0), new Size(this.Width, 23));
e.Graphics.CopyFromScreen(this.PointToScreen(new Point(0, 0)),
new Point(0, 0), new Size(3, this.Height));
e.Graphics.CopyFromScreen(this.PointToScreen(new Point(this.Width - 4, 0)),
new Point(this.Width - 4, 0),
new Size(3, this.Height));
e.Graphics.CopyFromScreen(this.PointToScreen(new Point(0,
this.Height - 4)),
new Point(0, this.Height - 4),
new Size(this.Width, 3));
if (FocusForm==this)
{
e.Graphics.FillRectangle(new SolidBrush(
this.ColorSetting.ActiveHeaderColor),
new Rectangle(new Point(0, 0), this.Size));
}
else
{
e.Graphics.FillRectangle(new SolidBrush(
this.ColorSetting.HeaderColor),
new Rectangle(new Point(0, 0), this.Size));
}
e.Graphics.SmoothingMode =
System.Drawing.Drawing2D.SmoothingMode.HighSpeed;
System.Drawing.Drawing2D.GraphicsPath path =
new System.Drawing.Drawing2D.GraphicsPath();
path.AddLine(2, 0, this.Width - 4, 0);
path.AddLine(this.Width - 4, 0, this.Width - 2, 2);
path.AddLine(this.Width - 2, 2, this.Width - 2, this.Height - 4);
path.AddLine(this.Width - 2, this.Height - 4,
this.Width - 4, this.Height - 2);
path.AddLine(this.Width - 4, this.Height - 2, 2, this.Height - 2);
path.AddLine(2, this.Height - 2, 0, this.Height - 4);
path.AddLine(0, this.Height - 4, 0, 2);
path.AddLine(0, 2, 2, 0);
System.Drawing.Drawing2D.GraphicsPath pathFrame =
new System.Drawing.Drawing2D.GraphicsPath();
pathFrame.AddLine(2, 0, this.Width - 3, 0);
pathFrame.AddLine(this.Width - 3, 0, this.Width - 1, 2);
pathFrame.AddLine(this.Width - 1, 2, this.Width - 1, this.Height - 3);
pathFrame.AddLine(this.Width - 1, this.Height - 4,
this.Width - 4, this.Height - 1);
pathFrame.AddLine(this.Width - 4, this.Height - 1, 2, this.Height - 1);
pathFrame.AddLine(2, this.Height - 1, 0, this.Height - 4);
pathFrame.AddLine(0, this.Height - 4, 0, 2);
pathFrame.AddLine(0, 2, 2, 0);
this.Region = new Region(pathFrame);
this.BackColor = this.ColorSetting.BackColor;
if (FocusForm == this)
e.Graphics.DrawPath(new
Pen(this.ColorSetting.ActiveBorderColor), path);
else
e.Graphics.DrawPath(new Pen(this.ColorSetting.BorderColor), path);
Rectangle clientRegion = new Rectangle(3, 23,
this.Width - 8, this.Height - 28);
e.Graphics.FillRectangle(new SolidBrush(
this.ColorSetting.BackColor), clientRegion);
if (FocusForm == this)
e.Graphics.DrawRectangle(new
Pen(this.ColorSetting.ActiveBorderColor), clientRegion);
else
e.Graphics.DrawRectangle(new
Pen(this.ColorSetting.BorderColor), clientRegion);
if(this.BackgroundImage!=null)
e.Graphics.DrawImage(this.BackgroundImage, clientRegion);
if(this.Icon!=null)
e.Graphics.DrawIcon(this.Icon, new Rectangle(3, 3, 16, 16));
Font f = new Font("ArialBlack", (float)9,FontStyle.Bold);
e.Graphics.DrawString(this.Text, f, Brushes.White, 21, 3);
e.Graphics.DrawString(this.Text, f, Brushes.White, 23, 3);
e.Graphics.DrawString(this.Text, f, Brushes.White, 22, 2);
e.Graphics.DrawString(this.Text, f, Brushes.White, 22, 4);
e.Graphics.DrawString(this.Text, f, Brushes.Black, 22, 3);
if (this.IsMinOn)
{
e.Graphics.DrawImage(
global::Sayes.Controls.Vista.Properties.Resources.MinHigh,
this.MinRegion.GetBounds(e.Graphics));
}
else
{
e.Graphics.DrawImage(
global::Sayes.Controls.Vista.Properties.Resources.Min,
this.MinRegion.GetBounds(e.Graphics));
}
if (this.IsCloseOn)
{
e.Graphics.DrawImage(
global::Sayes.Controls.Vista.Properties.Resources.CloseHigh,
this.CloseRegion.GetBounds(e.Graphics));
}
else
{
e.Graphics.DrawImage(
global::Sayes.Controls.Vista.Properties.Resources.Close,
this.CloseRegion.GetBounds(e.Graphics));
}
if (this.IsMaxOn)
{
e.Graphics.DrawImage(
global::Sayes.Controls.Vista.Properties.Resources.MaxHigh,
this.MaxRegion.GetBounds(e.Graphics));
}
else
{
e.Graphics.DrawImage(
global::Sayes.Controls.Vista.Properties.Resources.Max,
this.MaxRegion.GetBounds(e.Graphics));
}
}
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.