Introduction
The TB.TreeGrid
is an ASP.NET Webcontrol used to show hierarchy data in grid view.
Usually, we use GridView
Webcontrol to show hierarchy data, but it has only one column. It is not suitable for BOM or schedule plan, etc.
This control combines the advantages of GridView
and TreeView
, can show hierarchy data in multiple columns. It supports databind
, postback
, callback
, Microsoft Ajax, Event
(Edit, Select, Expand, Populate, Collapse, Remove, Update, Cancel, etc).
Please go here to download the latest source and demo.
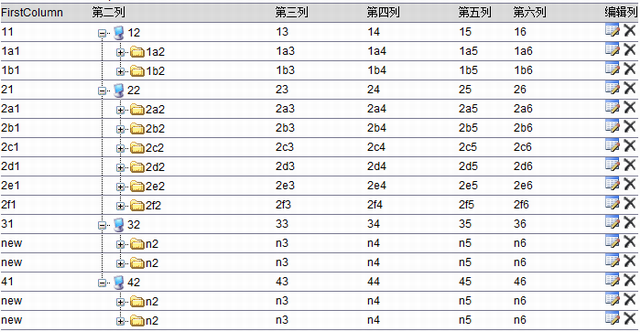
Using the Code
It has similar syntax to TreeView
and GridView
.
First, add TB.Web.UI.TreeGrid.dll as reference, you can find it in the bin directory.
Second, create a *.aspx file, add the control to the page.
<tbwc:treegrid ID="TreeGrid" runat="server" ShowHeader="true"
ShowFooter ="false" Caption="This is TreeGird's Caption"
ExpandDepth="1" PopulateNodesFromClient="false"
CaptionAlign="Left" NodeWrap="false" LineImagesFolder="~/TreeViewLines"
ShowLines="true" CssClass="tgDefault" ImageSet="XPFileExplorer"
NodeCellPosition="1" OnSelectedNodeChanged="TreeGrid_SelectedNodeChanged"
OnNodePopulate="TreeGrid_NodePopulate" OnRowCommand="TreeGrid_RowCommand"
onNodeDataBound="TreeGrid_NodeDataBound" >
<HeaderStyle BackColor="Gainsboro" />
<NodeStyle Display="Inline" />
<Columns>
<asp:TemplateField HeaderText="FirstColumn">
<itemtemplate><%# Eval("C1") %></itemtemplate>
<ItemStyle Width="100px" />
</asp:TemplateField>
<asp:TemplateField HeaderText="???">
<itemtemplate><%# Eval("C2") %></itemtemplate>
<ItemStyle Width="200px" />
</asp:TemplateField>
<asp:TemplateField HeaderText="???">
<itemtemplate><%# Eval("C3") %></itemtemplate>
<ItemStyle Width="100px" />
</asp:TemplateField>
<asp:TemplateField HeaderText="???">
<itemtemplate><asp:Literal ID="LtrForth" Text='<%# Eval("C4") %>'
runat="server"></asp:Literal></itemtemplate>
<ItemStyle Width="100px" />
</asp:TemplateField>
<asp:TemplateField HeaderText="???">
<itemtemplate><%# Eval("C5") %></itemtemplate>
<ItemStyle Width="60px" />
</asp:TemplateField>
<asp:TemplateField HeaderText="???">
<itemtemplate><%# Eval("C6") %></itemtemplate>
<edititemtemplate><asp:TextBox ID="TxtSixth" Text='<%# Eval("C6") %>'
runat="server" Width="60px" Height="12px">
</asp:TextBox></edititemtemplate>
<ItemStyle Width="100px" />
</asp:TemplateField>
<asp:TemplateField HeaderText="???">
<itemstyle cssclass="listOp" />
<itemtemplate>
<asp:ImageButton ID="LbtnEdit" runat="server" CommandName="Edit"
ToolTip="??" ImageUrl="img/opEdit.gif"></asp:ImageButton>
<asp:LinkButton ID="LbtnUpdate" runat="server" CommandName="Update"
Text="Update"></asp:LinkButton>
<asp:LinkButton ID="LbtnCancle" runat="server" CommandName="Cancel"
Text="Cancel"></asp:LinkButton>
<asp:ImageButton ID="LbtnDel" runat="server" CommandName="Remove"
ToolTip="??" ImageUrl="img/opDel.gif"></asp:ImageButton>
</itemtemplate>
</asp:TemplateField>
</Columns>
</tbwc:treegrid>
Third, open *.cs file, write databind code and event handler.
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
Builder.InitTreeGridManual(this.TreeGrid);
}
}
protected void TreeGrid_SelectedNodeChanged
(object sender, TB.Web.UI.WebControls.TreeGridNodeEventArgs e)
{
}
protected void TreeGrid_RowCommand
(object sender, TB.Web.UI.WebControls.TreeGridRowCommandEventArgs e)
{
this.LblHint.Text = "?????[Event Name:" + e.CommandName+ "] " +
DateTime.Now.ToString();
if (e.CommandName == "Edit")
{
TreeGridNode oNode = this.TreeGrid.EditingNode;
e.Row.Owner.Edit();
if (oNode != null)
{
oNode.DataItem = Builder.GetNodeData(oNode.Value);
oNode.RaiseNodeDataBind(false);
}
e.Row.Owner.DataItem = Builder.GetNodeData(e.Row.Owner.Value);
e.Row.Owner.RaiseNodeDataBind(false);
}
if (e.CommandName == "Update")
{
e.Row.Owner.Editing = false;
string newValue = ((TextBox)e.Row.FindControl("TxtSixth")).Text;
e.Row.Owner.DataItem = Builder.GetNodeData(e.Row.Owner.Value);
((Foo)e.Row.Owner.DataItem).C6 = newValue;
e.Row.Owner.RaiseNodeDataBind(false);
}
if (e.CommandName == "Cancel")
{
e.Row.Owner.Editing = false;
e.Row.Owner.DataItem = Builder.GetNodeData(e.Row.Owner.Value);
e.Row.Owner.RaiseNodeDataBind(false);
}
if (e.CommandName == "Remove")
{
if (e.Row.Owner.Parent != null)
{
e.Row.Owner.Parent.ChildNodes.Remove(e.Row.Owner);
}
else
{
this.TreeGrid.Nodes.Remove(e.Row.Owner);
}
}
}
protected void TreeGrid_NodePopulate
(object sender, TB.Web.UI.WebControls.TreeGridNodeEventArgs e)
{
TreeGridNode node = new TreeGridNode("new1");
node.DataItem = Builder.GetNodeData("-1");
e.Node.ChildNodes.Add(node);
node.PopulateOnDemand = true;
node.RaiseNodeDataBind(false);
node = new TreeGridNode("new2");
node.DataItem = Builder.GetNodeData("-1");
e.Node.ChildNodes.Add(node);
node.PopulateOnDemand = true;
node.RaiseNodeDataBind(false);
}
protected void TreeGrid_NodeDataBound(object sender, TreeGridRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow)
{
if (e.Row.RowState == DataControlRowState.Edit)
{
((LinkButton)e.Row.FindControl("LbtnUpdate")).Visible = true;
((LinkButton)e.Row.FindControl("LbtnCancle")).Visible = true;
}
if (e.Row.RowState == DataControlRowState.Normal)
{
((LinkButton)e.Row.FindControl("LbtnUpdate")).Visible = false;
((LinkButton)e.Row.FindControl("LbtnCancle")).Visible = false;
}
}
}
Above is a simple introduction, I will update it shortly.
You can download the demo.zip and run it. The project is a Visual Studio 2008 project.
History
- 29th September, 2008: Initial post
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.