Introduction
Through this tip, you will learn in a step by step manner to easily send email using System.Net.Mail
.
Background
This tip may be useful for intermediate developers who have some basics in C#, ASP.NET MVC, JQuery, JavaScript.
Using the Code
A) Operating Process
The first thing you need to know is how you can get the parameters of SMTP Server. The following links will help you to find them:
Examples of SMTP configuration:
- Office365: Address: smtp.office365.com, Port: 587, SSL: true
- Gmail: Address: smtp.gmail.com, Port: 587, SSL: true
- Yahoo: Address: smtp.mail.yahoo.com, Port: 587, SSL: true
B) Source Code
1) Server Code
* ConfigurationSMTP class
public class ConfigurationSMTP
{
public static string smtpAdress = "smtp.mail.yahoo.com";
public static int portNumber = 587;
public static bool enableSSL = true;
public static string from = "sender email";
public static string password = "password of the above email";
}
* SendNewMessage method
This method contains the main code that sends a new message to a specific e-mail address.
How it works:
- Build a message (using
MailMessage()
class) thanks to data received from HTTP request such as: 'Destination Email', 'Subject', 'Message Body'. - Create and configure the SMTP client using
SmtpClient()
class. - Attach a credentials parameter when trying the creation of SMTP connection (using
smtp.Credentials
method). - Send a message.
[HttpPost]
public ActionResult SendNewMessage()
{
try
{
Response.StatusCode = 200;
string smtpAddress = ConfigurationSMTP.smtpAdress;
int portNumber = ConfigurationSMTP.portNumber;
bool enableSSL = ConfigurationSMTP.enableSSL;
string emailTo = Request.Params["to"];
string subject = Request.Params["subject"];
StringBuilder body = new StringBuilder();
body.Append("<html><head> </head><body>");
body.Append("<div style=' font-family: Arial;
font-size: 14px; color: black;'>Hi,<br><br>");
body.Append(Request.Params["message"]);
body.Append("</div><br>");
body.Append(string.Format("<span style='font-size:11px;font-family:
Arial;color:#40411E;'>{0} - {1} {2}</span><br>",
MessageModel.adress, MessageModel.zip, MessageModel.city));
body.Append(string.Format("<span style='font-size:11px;font-family:
Arial;color:#40411E;'>Mail: <a href=\"mailto:{0}\">{0}</a>
</span><br>", ConfigurationSMTP.from));
body.Append(string.Format("<span style='font-size:11px;font-family:
Arial;color:#40411E;'>Tel: {0}</span><br>",
MessageModel.phone));
body.Append(string.Format("<span style='font-size:11px;font-family:
Arial;'><a href=\"web site\">{0}</a>
</span><br><br>", MessageModel.link));
body.Append(string.Format("<span style='font-size:11px; font-family:
Arial;color:#40411E;'>{0}</span><br>", MessageModel.details));
body.Append( "</body></html>");
using (MailMessage mail = new MailMessage())
{
mail.From = new MailAddress(ConfigurationSMTP.from);
mail.To.Add(emailTo);
mail.Subject = subject;
mail.Body = body.ToString();
mail.IsBodyHtml = true;
string localFileName = "~/Content/TestAttachement.txt";
mail.Attachments.Add(new Attachment
(Server.MapPath(localFileName), "application/pdf"));
using (SmtpClient smtp = new SmtpClient(smtpAddress, portNumber))
{
smtp.Credentials = new NetworkCredential
(ConfigurationSMTP.from, ConfigurationSMTP.password);
smtp.EnableSsl = enableSSL;
smtp.Send(mail);
}
}
}
catch (Exception ex)
{
Response.StatusCode = 400;
}
return null;
}
2) Client Code
* Index.cshtml
This file contains two sections:
- HTML and CSS code: used to create and design form controls
- JavaScript code: used to capture the
onsubmit
event of contact form and build an HTTP request that invokes SendNewMessage
method
<script type="text/javascript">
$(document).ready(function () {
$("#idFormContact").on("submit", function (e) {
e.preventDefault();
var url = "/Home/SendNewMessage";
var formdata = (window.FormData) ? new FormData(this) : null;
var fdata = (formdata !== null) ? formdata : $form.serialize();
$("#idSubmitMvt").attr("disabled", true);
$("#idNotifSuccess").hide();
$("#idNotifError").hide();
$.ajax({
type: "POST",
url: url,
data: fdata,
processData: false,
contentType: false,
success: function (data) {
$("#idNotifSuccess").show();
},
error: function (xhr, ajaxOptions, thrownError) {
console.log("Error");
$("#idNotifError").show();
}
});
});
});
</script>
<div class="row">
<h2>Contact Form</h2>
<form class="col col-xs-6" id="idFormContact" >
<div class="form-group">
<label>Destination</label>
<input type="email" class="form-control"
name="to" value="" placeholder="Destination Email">
</div>
<div class="form-group">
<label>Subject</label>
<input type="text" class="form-control"
value="Test subject"
name="subject" placeholder="Subject">
</div>
<div class="form-group">
<label>Body</label>
<textarea class="form-control"
name="message">Test Message</textarea>
</div>
<button type="submit"
class="btn btn-primary">Submit</button>
<br>
<br>
<div id="idNotifError" style="display:none"
class="alert alert-danger">Fail to send a message</div>
<div id="idNotifSuccess" style="display:none"
class="alert alert-success">Message is sent</div>
</form>
</div>
C) Result
* enter data in the form, and submit.
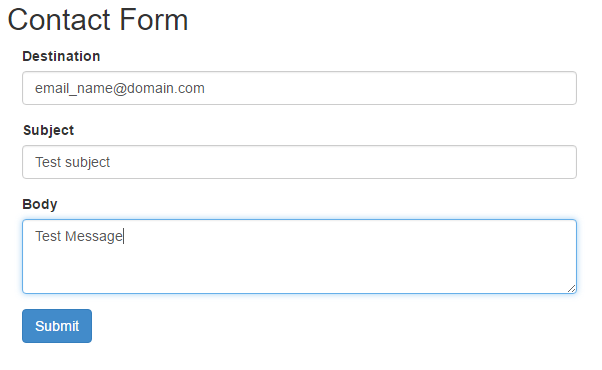
* the details of received message

In Closing
I hope that you appreciated this post. Try to download the source code. Please send me your questions and comments.
History
- v1 19/10/2016: Initial version
Microsoft certified professional in C# ,HTML 5 & CSS3 and JavaScript, Asp.net, and Microsoft Azure.