Introduction
In my previous post, I explained how to implement a basic REST based Web API service with multiple GET
methods and its consumption using JQuery client. In this post, I am going to explain how to consume a REST based Web API service from AngularJS client.
Note: This is only a sample intended to demonstrate multiple Web API GET methods in the same service class and its consumption using AngularJS. For security reasons, web pages cannot make calls to services (APIs) on a domain other than the one where the page originated. Here in this demo article, I won’t be looking into Cross-origin resource sharing (CORS) - sharing /accessing resources between domains other than the originating one. There is CORS NuGet package available targeting Web API 2.0 or later. Please refer to the MSDN documentation for details on this. Also, I won’t concentrate on SQL injection and input validation as it’s a demo version.
I am using Visual Studio Express 2013 for Web as my development environment targeting .NET Framework 4.5.
Here, I will be using the Math’s API Services project from my previous post, and adding a HTML page as client to consume the services via AngularJS.
Step 1
Please open Math’s API Services project (from my previous post) and add AngularJS library under scripts folder.
Note: You have options like adding using NuGet or download and add to the scripts folder or refer the content delivery network (CDN) server path as a best practice.
In this demo, I will be adding AngularJS.js file to the scripts folder and later referring it from the client page.
Step 2
Next add a HTML page under ‘Web’ folder and name it ‘ajsclient.html’. Now our solution explorer looks as below:
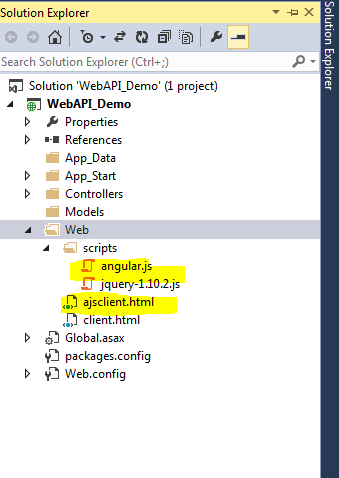
Step 3
Before we create our HTML client, we need to code our AngularJS Controller script file. Add a folder under \scripts\ and name the newly added folder as app to store our application specific Angular script files.
Create a javaScript file under \scripts\app folder to store client specific AngularJS code. Name the file as ‘calcclient.js’ and add the below code to define an AngularJS application and a controller to control the application.
var app = angular.module('CalcApp', []);
app.controller("CalcCtrl", function ($scope, $http) {
$scope.apiresult = 'Results from API call';
$scope.invokeapi = function (method) {
$scope.apiresult = "Calculating...";
var urlString = "/api/math/";
var arg = "value1=" + $scope.inputvalue1 + "&value2=" + $scope.inputvalue2;
urlString = urlString + method + "/?" + arg;
$http.get(urlString)
.success(function (data, status, header, config) {
var myjson = JSON.parse(data);
$scope.apiresult = JSON.parse(myjson);
})
.error(function (ex) {
$scope.apiresult = "error:" + ex.message;
})
}
});
In AngularJS - $scope
is an application level object that owns functions and variables. Inside the controller, we have three 3 $scope
level variables and a function defined.
$scope.apiresult
– Bind to our client output $scope.inputvalue1
- Bind with client input parameter $scope.inputvalue2
- Bind with client input parameter $scope.invokeapi
- Defined as a function to be invoked from client
Step 4
Add the below code to the ‘ajsclient.html’.
<!--
<!DOCTYPE html>
<html ng-app="CalcApp"> <!--
<head>
<title>Calculator Client- AngularJS</title>
<!--
<script src="scripts/angular.js"></script>
<script src="scripts/app/calcclient.js"></script>
</head>
<body>
<!--
<div style="text-align:center" ng-controller="CalcCtrl">
<h2>Calculator client - AngularJS</h2>
<!--
<!--
Please enter Value1 : <input type="number"
id="txtValue1" min="1" max="100"
ng-model="inputvalue1"><br><br>
Please enter Value2 : <input type="number"
id="txtValue2" min="1" max="100"
ng-model="inputvalue2"><br /><br>
<!--
Result from Math API Service : <input type="text"
id="txtResult" ng-disabled="true"
ng-model="apiresult" /><br /><br>
<!--
<input type="button" value="Add"
id="btnAdd" ng-click="invokeapi('ADD')">
<input type="button" value="Substract"
id="btnMinus" ng-click="invokeapi('SUBSTRACT')">
<input type="button" value="Multiply"
id="btnMultiply" ng-click="invokeapi('MULTIPLY')">
<input type="button" value="Divide"
id="btnDivide" ng-click="invokeapi('DIVIDE')">
</div>
</body>
</html>
Now our client interface is ready to consume the web API services. Our client for Math Web API services looks as below. We have two input fields and buttons to invoke the API services. Once the buttons are clicked, using AngularJS we invoke the respective API call and output the result.

To test the client, hit F5 (or whatever you have configured your debug key in Visual Studio) and our API services are ready to be consumed. Input some numeric values to the input text boxes. Then click on the respective button to invoke the API services. The output will be displayed in the output text area.
Note: Here in this demo version, Web API services and client page are in the same project. We can create a separate project for client and consume it. Just make sure to run the WEB API services before client consumption.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.