Introduction
If you’ve renewed your driver’s license any time in the last few years, you have likely noticed an increase in its visual complexity. Many governing bodies have made significant strides towards making forgery more difficult. The unfortunate side-effect from that positive change is that traditional recognition algorithms are more difficult with the many colors, backgrounds, holograms, and layouts.
Thankfully, new driver’s licenses also have PDF417 barcodes on them, and in North America, follow specifications laid out by the American Association of Motor Vehicle Administrators (AAMVA). These barcodes are small, error resistant, and can encode a lot of information.
This tutorial will introduce you to the classes and methods for both reading and writing AAMVA-compliant barcodes from Driver’s Licenses.
Reading AAMVA Barcodes
Since Driver’s Licenses are so broadly accepted as forms of identification, there are a wealth of opportunities for reading the information from them. We covered those concepts in an earlier white paper on Capturing Customer Information from Driver's Licenses, so we won’t repeat them here. That paper also discussed using the PDF417 barcodes before they were regulated by AAMVA. Since then, LEADTOOLS has added a new AAMVAID
class which does all the field parsing for you.
What’s the big deal? Take a look at the raw data for an AAMVA barcode:
@\n\u001e\rANSI 636004080002DL00410266ZN03070017DLDAQ123456789123\nDCSDOE\nDDEN\nDACJANE\nDDFN\nDAD\nDDGN\nDCAC\nDCBNONE\nDCDNONE\nDBD08152015\nDBB08151987\nDBA08152020\nDBC2\nDAU070 in\nDAYBRO\nDAG1100 NEW BERN AVENUE\nDAIRALEIGH\nDAJNC\nDAK276970001\nDCF0123456789\nDCGUSA\nDAZBRO\nDCLU \nDCK000012345678NCSVTL01\nDDB10242014\nDDK1\nDDL1\nZNZNADUP\nZNB\nZNC0\n
Where’s the name? Or the birthdate? Do you need to calculate if this person is over 21 and legally allowed to make a purchase? With LEADTOOLS, you can get that kind of information in beautiful, strongly typed properties:
using (AAMVAID id = BarcodeData.ParseAAMVAData(data.GetData(), false))
{
Console.WriteLine("Issuer Identification Number: " + id.IssuerIdentificationNumber);
Console.WriteLine("First Name: " + id.FirstName.Value);
Console.WriteLine("Last Name: " + id.LastName.Value);
Console.WriteLine("Over 21?: " + id.Over21);
}
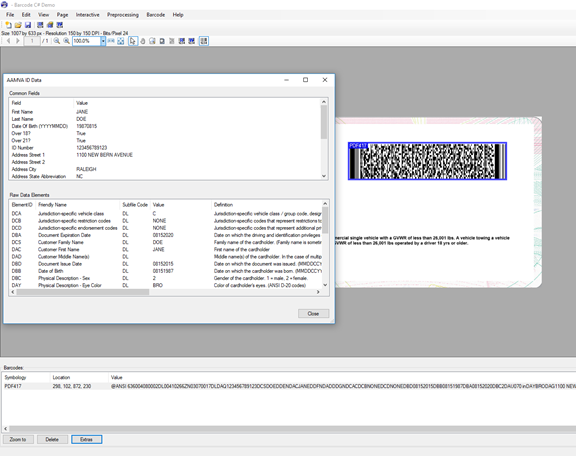
Writing AAMVA Barcodes
There may be fewer reasons for generating AAMVA barcodes, but every motor vehicle agency in every state or province in the United States, Canada, and Mexico needs the ability to print them on the licenses they issue. Naturally, you can’t just print any old barcode onto licenses and IDs. As with most specifications, they are hard to follow and can cause a significant development roadblock due to the R&D requirements. LEADTOOLS simplifies this in two ways: generating the AAMVA string and creating the PDF417 barcode.
First, you need to convert your customer data into a valid AAMVA string value. To aid in this process, LEADTOOLS provides the AAMVAIDBuilder
class. The builder will help organize the data and output it conforming to the 2016 AAMVA CDS. Here we initialize the AAMVAIDBuilder
, add some information and then use the Build
function to generate the AAMVAID
object:
AAMVAIDBuilder builder = new AAMVAIDBuilder();
builder.SetJurisdiction(AAMVAJurisdiction.NorthCarolina,
AAMVAID.LookupIssuerIdentificationNumber(AAMVAJurisdiction.NorthCarolina));
builder.SetVersion(AAMVAVersion.Version2016);
builder.SetJurisdictionVersion("00");
builder.SetNumberOfEntries(1);
builder.SetSubfileType(0, AAMVASubfileType.DL, "DL");
builder.AddDataElementToSubfile(0, "DAC", "John");
builder.AddDataElementToSubfile(0, "DCS", "Doe");
byte[] data;
using (AAMVAID id = builder.Build())
{
data = id.GetBytes();
}
Now that our customer’s information is correctly encoded for AAMVA, we will create the PDF417 barcode. Thanks to LEAD’s nearly three decades of experience in with raster and document imaging technologies, this process is even simpler than writing the AAMVA string despite the barcode’s greater complexity. Here we generate the BarcodeEngine
, set a few options to help the proportions make AAMVA happy, and LEADTOOLS takes care of the rest. In this simple case, we simply created a blank image using the calculated bounds of the barcode:
BarcodeEngine engine = new BarcodeEngine();
PDF417BarcodeWriteOptions writeOptions =
(PDF417BarcodeWriteOptions)engine.Writer.GetDefaultOptions(BarcodeSymbology.PDF417);
writeOptions.XModule = 15;
writeOptions.XModuleAspectRatio = 3;
writeOptions.ECCLevel = PDF417BarcodeECCLevel.Level5;
writeOptions.SymbolWidthAspectRatio = 4;
PDF417BarcodeData barcodeData =
(PDF417BarcodeData)BarcodeData.CreateDefaultBarcodeData(BarcodeSymbology.PDF417);
barcodeData.SetData(data);
const int resolution = 96;
engine.Writer.CalculateBarcodeDataBounds(LeadRect.Empty,
resolution,
resolution,
barcodeData,
writeOptions);
using (RasterImage image = RasterImage.Create(barcodeData.Bounds.Width,
barcodeData.Bounds.Height, 24,
resolution,
RasterColor.FromKnownColor(RasterKnownColor.White)))
{
engine.Writer.WriteBarcode(image, barcodeData, writeOptions);
using (RasterCodecs codecs = new RasterCodecs())
{
codecs.Save(image, imageFilePath, RasterImageFormat.Png, image.BitsPerPixel);
}
}
As a check, we’ll load the image back into the Main Barcode demo like before and read the data.

Conclusion
LEADTOOLS has a history of staying on the forefront of document imaging and recognition technologies like OCR, Forms, Barcode, and PDF. Driver’s licenses and IDs have become far more complex, yet better regulated with the adoption of AAMVA barcodes. LEADTOOLS will stay a viable option for developers wanting to quickly and easily create software to read and write identification data stored in AAMVA barcodes.
Download the Full AAMVA Barcode Example
You can download the fully functional demo which includes the features discussed above. To run this example you will need the following:
- LEADTOOLS free evaluation
- Visual Studio 2010 or later
- Browse to the LEADTOOLS Examples folder (e.g. C:\LEADTOOLS 20\Examples\) where you can find example projects for this and many more technologies in LEADTOOLS.
Support
Need help getting this sample up and going? Contact our support team for free technical support! For pricing or licensing questions, you can contact our sales team (sales@leadtools.com) or call us at 704-332-5532.
Since 1990, LEAD has established itself as the world's leading provider of software development toolkits for document, medical, multimedia, raster and vector imaging. LEAD's flagship product, LEADTOOLS, holds the top position in every major country throughout the world and boasts a healthy, diverse customer base and strong list of corporate partners including some of the largest and most influential organizations from around the globe. For more information, contact sales@leadtools.com or support@leadtools.com.