Collapsing Multiple Progress Bars






4.38/5 (13 votes)
An article on how to collapse the progress feedback given by multiple routines in a single progress bar
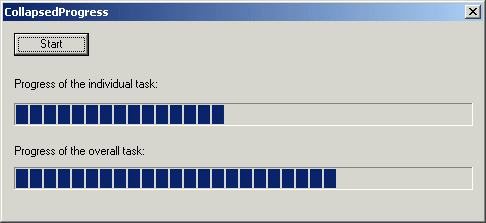
Introduction
Everybody knows the frustration caused by a new progress bar popping up and starting from 0% just after the previous one reached 100%. This annoyance is caused by several consecutive subroutines providing independent progress feedback. Collapsing these isolated progress bars into one bar running from 0 to 100% can be a nasty problem. In this article, an object-oriented approach is presented that provides an elegant and transparent solution to this problem.
Background
In complex software packages, there are often multiple lengthy tasks that provide progress feedback. A single user action could lead to a number of such tasks being executed, typically resulting in several consecutive instances of progress running from 0 to 100%. It is desirable to collapse the isolated instances of progress feedback into one overall instance of feedback running from 0 to 100%. This willl give the user a good notion of how much of the overall task has been finished and how much is left.
It is possible to solve this problem by hard-coding the progress range into every function or by passing the current progress as parameters to each function. However, the first solution is not very flexible (consider calling the same subroutines in a different order or different context) and the latter poses constraints to your code. Therefore this article presents a transparent and flexible solution to the above-described problem.
We introduce a CProgress
class that subscribes itself to a linked list containing all active progress feedback in the application. This subscription mechanism, however, is completely hidden from a programmer using the CProgress
class, which makes it very easy to use.
Using the Code
Consider a simple traditional class for progress feedback, CTraditionalProgress
, which could be used in the following way:
void A()
{
CTraditionalProgress myProgress;
myProgress.Set(0);
B(); //call function B
myProgress.Set(60);
C(); //call function C
myProgress.Set(100);
}
It is very simple to imagine how the code of CTraditionalProgress
could look. The Set()
function simply sets the progress bar to the number that is passed.
Now suppose that functions B()
and C()
have their own progress bars and suppose that we also want to capture that progress in the progress bar of function A()
. In other words, we want to collapse the progress feedback of functions B()
and C()
into the progress bar of function A()
. Well, that is exactly what the CProgress
class does for us. The beauty of it is that we hardly need to change any code. Most especially, we do not need to pass any additional parameters to the B()
and C()
subroutines.
One small adjustment to our code concerns replacing the Set()
member function with the SetRange()
function. Rather than indicating what percentage of the progress has already finished, this function indicates the fraction of the total time that the next block of code is expected to demand. The example code now looks like this:
void A()
{
CProgress myProgress;
myProgress.SetRange(0.60);
B(); //call function B
myProgress.SetRange(0.40);
C(); //call function C
}
void B()
{
CProgress myProgress;
myProgress.SetRange(0.20);
// Do something, estimated duration 20% of time for function B()
for (int n=0; n < 80; n++) {
myProgress.SetRange(0.01);
// Do something, estimated duration 1%
}
}
The progress feedback of function B()
is automatically mapped on the range of 0.60, which was specified in function A()
. If function B()
calls any routines that provide feedback regarding their progress, then that is again collapsed to the range that was specified in function B()
. This process of collapsing is illustrated in the figure below.

Points of Interest
If you are interested in the inner workings of the collapsing mechanism, have a look at the sample code and at the PDF article that is attached in the ZIP file. The main trick is the fact that each CProgress
instance registers itself to a chained list. Since this chained list does not have an explicit owner or parent, it was decided to call it an "orphaned chained list." It is not hard to understand the CProgress
source code. The class consists of less than 50 lines of code. That degree of efficiency could be reached because the traversal of the parent progress nodes is done by simple recursive calls.
History
- 7 November, 2007 -- Version 1.0
- 15 November, 2007 -- Downloads updated