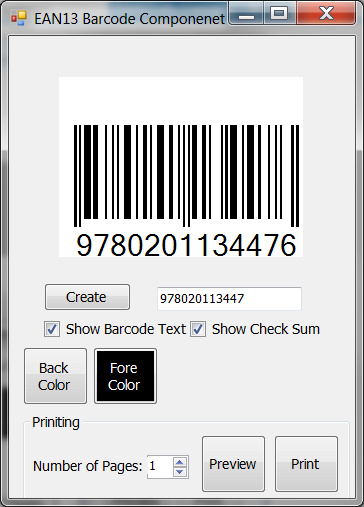
Introduction
In 2005, most of my work was barcode related programs, because I was writing programs for hand held devices which came with an integrated barcode reader. It was exciting to know about the barcode technology and how third party tools drew these bars. So I started looking for some resources to understand the algorithm used to generate barcodes. I discovered a lot of barcode types and each type had its own algorithm. My goal was to create my own barcode controls.
In the beginning of 2006, I started with this EAN13 barcode. After that, I paused because I found other exciting technologies. I know there are many articles in CodeProject that talk about the EAN 13 barcode, but I would like to share this with you.
Using the Code
This function initializes the barcode table which we will use to draw the bars:
Public Sub InitEAN13Tables()
Tables(0).TableA = "0001101"
Tables(0).TableB = "0100111"
Tables(0).TableC = "1110010"
Tables(1).TableA = "0011001"
Tables(1).TableB = "0110011"
Tables(1).TableC = "1100110"
Tables(2).TableA = "0010011"
Tables(2).TableB = "0011011"
Tables(2).TableC = "1101100"
Tables(3).TableA = "0111101"
Tables(3).TableB = "0100001"
Tables(3).TableC = "1000010"
Tables(4).TableA = "0100011"
Tables(4).TableB = "0011101"
Tables(4).TableC = "1011100"
Tables(5).TableA = "0110001"
Tables(5).TableB = "0111001"
Tables(5).TableC = "1001110"
Tables(6).TableA = "0101111"
Tables(6).TableB = "0000101"
Tables(6).TableC = "1010000"
Tables(7).TableA = "0111011"
Tables(7).TableB = "0010001"
Tables(7).TableC = "1000100"
Tables(8).TableA = "0110111"
Tables(8).TableB = "0001001"
Tables(8).TableC = "1001000"
Tables(9).TableA = "0001011"
Tables(9).TableB = "0010111"
Tables(9).TableC = "1110100"
End Sub
The function CalculateCheckSum
calculates the checksum code for the barcode:
Private Function CalculateCheckSum() As Boolean
Dim X As Integer = 0
Dim Y As Integer = 0
Dim j As Integer = 11
Try
For i As Integer = 1 To 12
If i Mod 2 = 0 Then
X += Val(m_BarcodeText(j))
Else
Y += Val(m_BarcodeText(j))
End If
j -= 1
Next
Dim Z As Integer = X + (3 * Y)
m_CheckSum = ((10 - (Z Mod 10)) Mod 10)
Return True
Catch ex As Exception
MessageBox.Show(ex.Message)
Return False
End Try
End Function
CalculateValue
is the main function which calculates the barcode values depending on the barcode table:
Private Function CalculateValue() As Boolean
BarcodeValue = New StringBuilder(95)
Try
BarcodeValue.Append(StartMark)
Select Case m_BarcodeText(0)
Case "0"
For i As Integer = 1 To 6
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableA)
Next
Case "1"
For i As Integer = 1 To 6
If (i = 1) Or (i = 2) Or (i = 4) Then
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableA)
Else
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableB)
End If
Next
Case "2"
For i As Integer = 1 To 6
If (i = 1) Or (i = 2) Or (i = 5) Then
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableA)
Else
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableB)
End If
Next
Case "3"
For i As Integer = 1 To 6
If (i = 1) Or (i = 2) Or (i = 6) Then
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableA)
Else
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableB)
End If
Next
Case "4"
For i As Integer = 1 To 6
If (i = 1) Or (i = 3) Or (i = 4) Then
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableA)
Else
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableB)
End If
Next
Case "5"
For i As Integer = 1 To 6
If (i = 1) Or (i = 4) Or (i = 5) Then
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableA)
Else
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableB)
End If
Next
Case "6"
For i As Integer = 1 To 6
If (i = 1) Or (i = 5) Or (i = 6) Then
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableA)
Else
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableB)
End If
Next
Case "7"
For i As Integer = 1 To 6
If (i = 1) Or (i = 3) Or (i = 5) Then
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableA)
Else
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableB)
End If
Next
Case "8"
For i As Integer = 1 To 6
If (i = 1) Or (i = 3) Or (i = 6) Then
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableA)
Else
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableB)
End If
Next
Case "9"
For i As Integer = 1 To 6
If (i = 1) Or (i = 4) Or (i = 6) Then
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableA)
Else
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableB)
End If
Next
End Select
BarcodeValue.Append(SplittingMark)
For i As Integer = 7 To (m_BarcodeText.Length - 1)
BarcodeValue.Append(Tables(Val(m_BarcodeText(i))).TableC)
Next
BarcodeValue.Append(Tables(CheckSum).TableC)
BarcodeValue.Append(EndMark)
Catch ex As Exception
MessageBox.Show(ex.Message)
End Try
End Function
Printing
In real life you need to print barcode lables to use them on the products and item, I had updated the control to support printing , also you can show/hide bracode value under the barcode bars.

Points of Interest
This barcode control was created just for fun and is not a professional control. We can add many properties to this. I think the bar sizes need some extra handling to enable printing in deferent sizes. Maybe if I have some time, I will write controls for other barcode types like pdf417.
Enjoy :)
Changelog
Version 0.1 :
Version 0.2 :
- Added Show barcode number under barcode bars.
- Added Barcode printing support.
010011000110100101101011011001010010000001000011011011110110010001101001011011100110011100100001