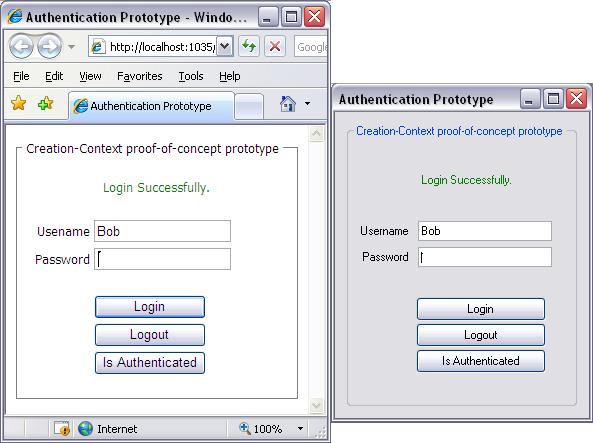
Objective
This article introduces a method for developing Object/Aspect-Oriented applications with concern about the location aspect of an object (known here as Object-Creation Context), and shows how it can reduce development time, cost, and resources while improving the desired quality of the product.
Introduction
The proof-of-concept prototype in this article simply authenticates a user against data in a persistence media (in an XML file). User can submit his/her Username and Password to the AuthenticationBiz
object in the Business Layer, and it checks the current logged in user authentication status. The AuthenticationBiz
object is being instantiated in the HttpSession context of the page in order to maintain user credentials on each and every post back by using the Web Context Provider defined in Framework.Core.Web.UI.dll. Before jumping into details, let me suggest you to download the sample proof-of-concept prototype mentioned above, execute it in Visual Studio .NET environment, and have a glimpse at the code written in various layers (specifically the Business Objects that are being used nested and in the User-Interface layer).
Background
It is useful to have a basic knowledge of web-application development using Application Server Objects such as HttpSession
and HttpApplication
in order to understand the proof-of-concept prototype sample.
Object-Creation Context Attribute Concept
Any object is located in a context that can be considered as an attribute of that object, and can be described differently depending on the subject. For example: if we want to describe a swimmer (Object) in an Olympic competition (Subject), then a specific swimming pool in the host country identifies his or her location (Attribute). If you change the subject to holiday in the above example, then a specific beach in the host country can identify his or her location (Attribute).
Consider a simple word processing application (Object) in an Operating System (Subject); the computer RAM or Hard-Disk can identify as the application location (Attribute). One might ask; where is the object being created, in the RAM or in the Hard-Disk? Which one is the context attribute of that object? It can be either one or both! In fact, the context attribute identifies the default location of the object at the time of its instantiation.
Notepad (Object) for example, in MS-Windows Operating System (Subject), is being created in the Virtual Memory allocated by the Operating System (the default attribute for almost all Windows applications). In software development, objects in the Business Layer of an application can have various Creation-Context attributes depending on the subject. For example: an authentication business object in a user access check (Subject) can hold the credentials for the current user session (Attribute), and an Authorization business object can hold a table of role access rights to the resources for all the sessions of the users (throughout the application).
Object-Creation Context Attribute Usage
In object-oriented programming languages, we are not usually giving our attention to an object location in the system, or even if we do, then probably that object is not located in the heap or stack. That is perfectly fine, and is the default Creation-Context attributes of objects, but if we want to have an object to be created in a different context (such as HttpSession, or Singleton in Remoting), then we need to write a bunch of code and apply various settings. Using the Object-Creation context attribute, an object can have its default Creation-Context identified with an attribute. For example: an object (such as an Authentication business object) with the ContextCreation.Session
attribute will be instantiated in the HttpSession of a page using CreationFactory
and the proper Context Creation Provider.
The proof-of-concept sample included in this article introduces a simple yet practical method that leaves programmers out of State Management troubles of web-application development. Object-Creation Context can be used to implement the business logic of a framework to create an application that supports both Windows and Web-based user interfaces with maximum code reuse and minimum effort.
The Business objects in the framework of the proof-of-concept sample application uses this method to represent the efficiency of using object Creation-Context in developing Web-applications. Business classes (such as: Order Management, User Authentication) are being located in a separate library (.NET assembly) and can be used in both Windows and Web-based applications. The Context Attribute of Business classes identifies where they can be instantiated. In the example (like all multi-layer applications), each Horizontal layer depends on its underlying layers and provides services to its above layers. Meaning that, horizontal application layers cannot (and should not) be used without the existence of the layer(s) below, but can provide services to zero or more upper layer consumers.
The proof-of-concept sample uses the prevalent 3-tier application architecture with focus on Business Layer: Business Layer (BL) provides services to the User Interface Layer (UI) and of course, can listen for upcoming events from the UI layer. BL also consumes the Data Access Layer (DAL) services and can raise events for the underlying layers.
Maintaining the state of an object in a web application is a challenging task that requires a lot of manual work using server objects and/or cookies. In order to simplify web-application development and relieve developers to concentrate on their application business needs as opposed to state management issues, the business objects in the Business Layer can be created and maintained in server objects (such as HttpSession, HttpApplication) so that it is available in each post-back as long as the Session is valid. The sample maintains Business Objects in a server object of the page context.
Using the Code
The provided samples in this article are developed to clarify Object Creation-Context theory and its usage. The proof-of-concept prototype demo has an authentication mechanism to persist user credentials in a Session, and a series of core framework libraries that implement Object-Creation Context for Web and Windows applications. The core framework consists of the following libraries:
- Framework.Core.Business.dll: Business Base classes, Business Exceptions, Business Factory, and Business Events.
- Framework.Core.Common.dll: A common exception that is the base for Business and UI Exceptions.
- Framework.Core.Web.UI.dll: Context provider classes for Session and Application server objects.
- Framework.Core.Win.UI.dll: Context provider that behaves similar for both Session and Application Creation context (
CreationContextAttribute
).
Any program that uses the core framework should include the Core libraries in its corresponding layers. For example: the Security demo consists of Business, Common, and UI layers, and its Business layer should include the Core.Business and Core.Common libraries. Common should have Core.Common and the Web UI should have Core.Web.UI in it as well as the other two Core libraries.

The common layer can consist of any entity type. In the Security demo, I used a database (SecurityDataSet.xsd).

In the Business layer, each Business object can have its own creation context depending on its subject. In the Security demo:
- An instance of the
UsersBiz
class (Object) is created in the server application memory (Creation Context) and remains in the server memory for every page post-back of all the users (Subject). So, all user credentials (SecurityDataSet_securityDataSet
) will be in the server memory as opposed to being fetched from the database for each and every authentication process.
[CreationContext(CreationContextLocation.Application)]
public class UsersBiz : BizBase
{
private const string UsersDataFile = "Users.xml";
private SecurityDataSet _securityDataSet = null;
public EnumerableRowCollection<SecurityDataSet.SYS_tblUserRow> Users…
}
An instance of AuthenticationBiz
class (Object) is created in the server session memory (Creation Context) and remains in the server memory for every page post-back of the current user (Subject). So, the user credential information (SYS_tblUserRow_credentials
) will be available on every post-back of a session. Note that the _usersBiz
object is created using the CreateBusiness
method as opposed to using the new
language keyword.
[CreationContext(CreationContextLocation.Session)]
public class AuthenticationBiz : BizBase
{
private SecurityDataSet.SYS_tblUserRow _credentials;
private UsersBiz _usersBiz;
public AuthenticationBiz(IBizContextProvider<BizBase> sessionBizCtx,
IBizContextProvider<BizBase> applicationBizCtx)
: base(sessionBizCtx, applicationBizCtx)
{
_usersBiz = CreateBusiness<UsersBiz>();
}
public bool Login(string username, string password)…
public bool Logout()…
public bool IsAuthenticated…
}
User interface (either Web or Windows) can create an instance of a Business object using the CreateBusiness<BizBase>
method similar to creating Business objects inside another Business object in the Business Layer. Depending on the Creation-Context attribute, the object can be created in the HttpSession or HttpApplication memory. Following is a code-behind for the web-based application. The class should inherit from the PageBase
class for the Web based user interfaces:
publicpartial class FrmLogin : PageBase
{
private AuthenticationBiz _authentication;
protected void Page_Load(object sender, EventArgs e)
{
_authentication = CreateBusiness<AuthenticationBiz>();
}
protected void btnLogin_Click(object sender, EventArgs e)
{
try
{
if (_authentication.Login(txtUserName.Text, txtPassword.Text))
ShowMessage("Login Successfully.", Color.Green);
}
catch (BizException bizException)
{
ShowMessage(bizException.Message, Color.Red);
}
}
protected void btnLogout_Click(object sender, EventArgs e)
{
try
{
if (_authentication.Logout())
ShowMessage("Logout Successfully.", Color.Blue);
}
catch (BizException bizException)
{
ShowMessage(bizException.Message, Color.Red);
}
}
protected void btnAuthenticated_Click(object sender, EventArgs e)
{
if (_authentication.IsAuthenticated)
ShowMessage("User Is Authenticated.", Color.Green);
else
ShowMessage("User Is Not Authenticated.", Color.Red);
}
}
The class should inherit from the FormBase
class for Windows Forms user interfaces:
publicpartialclassFrmLogin : FormBase
{
private AuthenticationBiz _authentication;
public FrmLogin()
{
InitializeComponent();
_authentication = CreateBusiness<AuthenticationBiz>();
}
private void btnLogin_Click(object sender, EventArgs e)
{
try
{
if (_authentication.Login(txtUserName.Text, txtPassword.Text))
ShowMessage("Login Successfully.", Color.Green);
}
catch (BizException bizException)
{
ShowMessage(bizException.Message, Color.Red);
}
}
private void btnLogout_Click(object sender, EventArgs e)
{
try
{
if (_authentication.Logout())
ShowMessage("Logout Successfully.", Color.Blue);
}
catch (BizException bizException)
{
ShowMessage(bizException.Message, Color.Red);
}
}
private void btnIsAuthenticated_Click(object sender, EventArgs e)
{
if (_authentication.IsAuthenticated)
ShowMessage("User Is Authenticated.", Color.Green);
else
ShowMessage("User Is Not Authenticated.", Color.Red);
}
}
As you can see, the business object instantiation and usage are the same for both Windows and Web user interfaces.
Conclusion
Using the Object Creation-Context attribute in an application reduces the amount of code a developer should write to identify the object location in the system. The object location can be identified once at design time and be used many times while developing the application. That means, spending less time developing applications, and improving quality by extracting the complexity involved in placing objects in their context location.
Points of Interest
Object-Creation Context can be used in Service Oriented Architecture (SOA) applications as a base to distribute processing across different locations. That means, an object instantiation request can be sent to the Enterprise-Service Bus (ESB), and the ESB can provide an instance of the requested object in a location that is identified by the Creation-Context attribute of the object.
History
Version 1.0.0.0.