Over the past decade, Dynamsoft has been dedicated to the document scanning and management markets. We have been continuously improving and polishing Dynamic Web TWAIN — the enterprise-class web document scanning and imaging SDK. In the latest version of Dynamic Web TWAIN, Dynamsoft brought a set of new APIs based on JSON data interchange, which aims to simplify the programming difficulty.
In this post, we discuss how to control your scanners and acquired images easily and efficiently using JSON objects. The article is also a guide for beginners to get started with Dynamic Web TWAIN programming.
What is Dynamic Web TWAIN?
Dynamic Web TWAIN is a browser-based document scanning SDK specifically designed for web applications. It is a cross-platform SDK which supports Windows, Linux, and macOS.
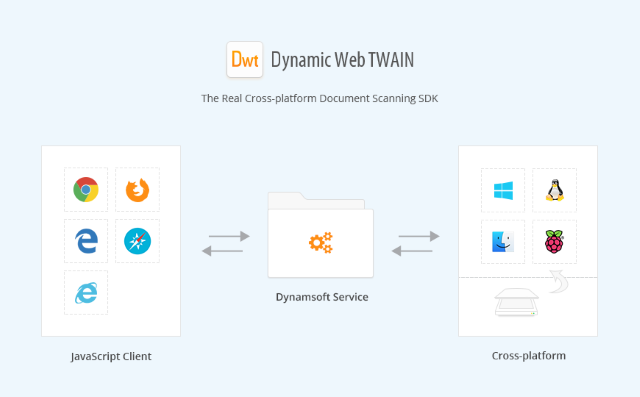
The SDK was originally designed for TWAIN-compatible devices. It is why the product name contains "TWAIN". Nowadays, the supported scanning protocols of Dynamic Web TWAIN also include SANE and ICA, which make scanners accessible and operable in Linux and macOS.
With just a few lines of JavaScript code, developers can easily integrate robust document scanning into web applications. In addition to document scan, Dynamic Web TWAIN features image editing, saving, uploading and etc.
Dynamic Web TWAIN SDK
License
Get a FREE 30-day trial license.
Developer’s Guide
https://www.dynamsoft.com/docs/dwt/index.html
API Documentation
https://www.dynamsoft.com/docs/dwt/API/API-Index.html
Code Gallery
https://www.dynamsoft.com/Downloads/WebTWAIN-Sample-Download.aspx
Build a "Hello World" Web-scanning Program
Let’s try the full-fledged Dynamic Web TWAIN Windows Edition.
First, download Dynamic Web TWAIN SDK and follow the prompts to complete the installation process
While installing the SDK, you can also include some add-ons such as Barcode Reader, OCR Basic, OCR Pro, PDF Rasterizer, Mobile Camera Capture, and Webcam Capture, to further empower your applications.

The Windows installer has already bundled the scanning services for all platforms. You can find .deb, .msi, .pkg and .rpm installers under <Dynamic Web TWAIN>\Resources\dist directory:

With only one copy of the codebase, your web document scanning application can work in Windows, Linux, and macOS as long as the corresponding Dynamsoft service is installed for the particular platform.
There are many useful samples located in the <Dynamic Web TWAIN>\Samples directory that you can modify and deploy:

You can try running a simple document scanning app by double-clicking Getting Started\HelloWorld.html. If you don’t have a scanner connected, there is a virtual scanner available for download.
HelloWorld.html:

Here is the source code:
<!DOCTYPE html>
<html>
<head>
<title>Hello World</title>
<script type="text/javascript" src="Resources/dynamsoft.webtwain.initiate.js"></script>
<script type="text/javascript" src="Resources/dynamsoft.webtwain.config.js"></script>
</head>
<body>
<div id="dwtcontrolContainer"></div>
<input type="button" value="Scan" onclick="AcquireImage();" />
<script type="text/javascript">
function AcquireImage() {
var DWObject = Dynamsoft.WebTwainEnv.GetWebTwain('dwtcontrolContainer');
if (DWObject) {
DWObject.SelectSource(function () {
var OnAcquireImageSuccess, OnAcquireImageFailure;
OnAcquireImageSuccess = OnAcquireImageFailure = function () {
DWObject.CloseSource();
};
DWObject.OpenSource();
DWObject.IfShowUI = false;
DWObject.Resolution = 300;
DWObject.PixelType = EnumDWT_PixelType.TWPT_GRAY;
DWObject.AcquireImage(OnAcquireImageSuccess, OnAcquireImageFailure);
}, function () {
console.log('SelectSource failed!');
});
}
}
</script>
</body>
</html>
To implement a simple document scanning web application, follow the basic steps below:
- Instantiate the Dynamic Web TWAIN object
- Select a scanning device
- Open the scanner source
- Acquire images from the scanner
Replacing JavaScript Code with JSON Object
Developers do not need to write much JavaScript code when using the latest Dynamic Web TWAIN SDK. Instead, a new way to communicate between Dynamsoft service and the web client is to package scanning operations and commands into a JSON object.
Convert the JavaScript code above into a JSON object as follows:
<!DOCTYPE html>
<html>
<head>
<title>Hello World</title>
<script type="text/javascript" src="Resources/dynamsoft.webtwain.initiate.js"></script>
<script type="text/javascript" src="Resources/dynamsoft.webtwain.config.js"></script>
</head>
<body>
<div id="dwtcontrolContainer"></div>
<input type="button" value="Scan" onclick="AcquireImage();" />
<script type="text/javascript">
function AcquireImage() {
var DWObject = Dynamsoft.WebTwainEnv.GetWebTwain('dwtcontrolContainer');
if (DWObject) {
DWObject.startScan({
exception: "fail",
ui: { bShowUI: false },
settings: {
Resolution: 300,
IfDisableSourceAfterAcquire: true,
pixelType: EnumDWT_PixelType.TWPT_GRAY
}
}).then(function(settings){
}).catch(function(err){
alert(JSON.stringify(err));
});
}
}
</script>
</body>
</html>
For debugging purposes, let’s try to trigger an exception, change the pixel type to 17 (an unsupported value):
settings: {
Resolution: 300,
IfDisableSourceAfterAcquire: true,
pixelType: 17
}

For more information about the JSON object properties, please reference <Dynamic Web TWAIN >\ Documents\Dynamic Web TWAIN Developer's Guide.pdf.
What is the Advantage of using a JSON Object?
Since Dynamsoft service supports JSON data interchange, JavaScript is not the only programming language for building document scanning app anymore. The new communication model allows developers to send scanning requests and receive scanned documents encoded as base64 strings. In the near future, you can use Java, Python or other high-level programming languages to create document scanning and management applications in any operating systems. Subscribe to our newsletter and be notified as soon as it becomes available.

Technical Support
If you have any questions about Dynamic Web TWAIN SDK, please feel free to contact support@dynamsoft.com.
New:
- [ActiveX] Added method
GetImagePartURL
to work with the Barcode Reader - [HTML5] Added a few global settings which may speed up the initialization process of the SDK. The settings are
IfCheckDCP
, IfCheckDWT
, IfDisableDefaultSettings
and IsLicensePromptFriendl
Improved:
- [HTML5 | Windows] Improved the TIFF compression algorithm when it's set to JPEG so that the resulting file size is significantly reduced
- [ActiveX & HTML5 | Windows] Included the Webcam and Barcode reader libraries in the service installer for easier distribution
- [HTML5] Improved the built-in image viewer to be more responsive when navigating through images