Introduction
We all know Databases is heart of any application, and some time its too complex to manage right? Yes. That’s why Microsoft come up with the Entity Framework : which reduce complexity and make easy for developers to write great program without having much more idea of Database. Thats why now-a-days most of Microsoft .Net developers use the .Net Entity Framework for database operation.
What is the Entity framework?
The Entity framework is an object-relation mapper, means - it takes the structure of the database and turns it into objects that the .Net framework can understand. Developers use those object to interact with the database instead of interacting with database directly. It’s possible to perform the full set of Create, Read, Update, and Delete (CRUD) operations using the Entity Framework features.
It has three work flows
-
Code – first,
-
Model-first and
-
database first.
Choosing and using the right approach can save developers a lot of time and effort, especially when interacting with complex database design.
In this Article we will work through the Code-first work flow with simple Demo Application.
Cover part:
- Understanding the Code First Work-flow
- Simple Demonstration on code-frist approach with MVC Application.
Understanding the Code First Work-flow
The code first approach is introduced in Entity Framework 4.1, and is a latest workflow Microsoft has introduced. It lets us transform our coded classes into a database application, which means code first lets us to define our domain model using POCO (plan old CLR object) class rather than using an xml - based EDMX files, which has no dependency with entity framework. Our model classes becomes the domain model, there for we most have to be very conscious in designing our model classes. And the rest work will be done by entity-frame work. This is the beauty of the code first approach where our model classes are become the data models on which Entity framework relies.
Simple Demonstration on code-frist approach with Asp.Net MVC Application
1) Create a class library project Named as “CodeFirstModel” and solution Named as “CodeFirstDemoApplication”. Open VS -> File ->New Project -> Class library project. Refer screen shot below.
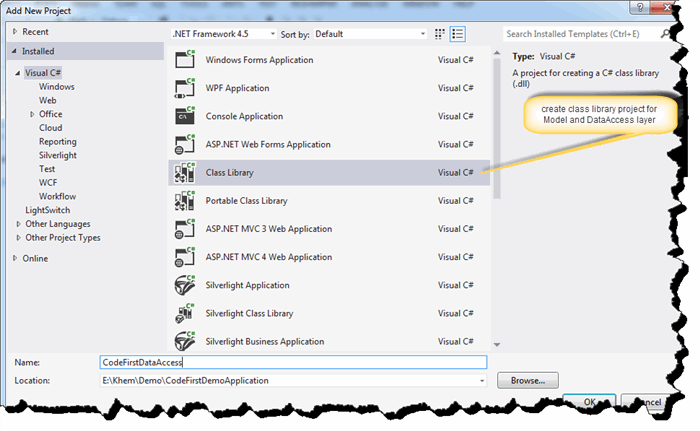
And new class named as “Employee.cs”. Project name->right click-> Add-> class.
public class Employee
{
public int EmployeeId { get; set; }
public string Name { get; set; }
public string Description { get; set; }
public bool IsEmployeeRetired { get; set; }
public string Country { get; set; }
public string Company { get; set; }
}
Code-first will use this model class to define data model on which entity framework relies. It has convention (we will discuss more in next article) that if it finds a property named Id or a property with the combined name of type name and Id (i.e EmployeeId) that property will be automatically configured as the Primary key and not null and also this key marked as auto-increment identity in the table. If it cannot find any property that matches this convention, it will throw an exception at run-time telling there is no key (I.e EntityType “class name” has no key defied. Define the key for this entity type).
2) Create another Class library project and named as “CodeFirstDataAccess” : Right click project solution -> Add -> new Project -> Class library project and Install Entity framework form NuGet Package manager. Select Project -> right click -> Manage NuGet Packages -> Search Entity FrameWork -> Install. refer below screen shot.

i) Add the reference of entity framework dll and “CodeFirstModel” to the current project "i.e CodeFirstDataAccess".
ii) Add a class named as “DemoEntityContext.cs” - Then implement DBContext and DBSet to interact with database and its operations.
public class DemoEntityContext : DbContext
{
public DemoEntityContext() : base("name=DbConnectionString") { }
public DbSet<Employee> Employees { get; set; }
}
This simple class represents the whole data layer for our application. ”DBConnectionString” is the connection string name defined in web.config file(discuss later).
DBContext – In simple this class is responsible to interact with database, and also to manage the entity objects during run time, which includes populating objects with data from a database, change tracking, and persisting data to the database.
DBSet - This class represents an entity set that is used for the create, read, update, and delete operations.
3) Add an Empty MVC web application named as “CodeFirstWebApp” which will use the above datalayer for db operation. Right click on project solutions -> Add -> new Project -> ASP.NET MVC4 Web application. refer screen shot

i) Add HomeController.cs controller, Index.cshtml (razor view) view, and then add a _partial view (Check partial detail ) to display employee records “_EmployeeDetailView.cshtml” and a model class named as “EmployeeModel.cs” and include jquery file too.
a) Content in Index.cshtml
@{
ViewBag.Title = "Index";
}
<!DOCTYPE html>
<meta name="viewport" content="width=device- <title>Index</title>
<body>
<input type="button" value="InsertRecord" id="btnInsertRecord"/>
<input type="button" value="DisplayRecords" id="btnDisplay"/>
<div id="divPartial">
</div>
</body>
<script src="~/Content/jquery-1.7.1.min.js"></script>
<script type="text/javascript">
$(function () {
$('#btnInsertRecord').click(function (data) {
$.post("@Url.Action("InsertEmployeeDetail", "Home")", function (data) {
if (data) {
alert('Successfuly inserted!!');
} else {
alert('An error occured!');
}
});
});
$('#btnDisplay').click(function (data) {
$.post("@Url.Action("GetEmployeeDetails", "Home")", function (data) {
if (data) {
$('#divPartial').append(data);
} else {
alert('An error occured!');
}
});
});
});
</script>
</html>
Two button to insert and display info, which trigger the action method present in the HomeController.cs class.
b) Content in HomeController.cs
public ActionResult Index()
{
try
{
Database.SetInitializer(new DropCreateDatabaseIfModelChanges<DemoEntityContext>());
return View();
}
catch (Exception)
{
throw;
}
}
DropCreateDatabaseIfModelChanges : If any model class is changed and we want to update that, then we need to implment this class. This will delete, recreate the database. You can remove this once changes is done.
DropCreateDatabaseAlways : which will delete and recreate database every time when context is initialize.
public ActionResult InsertEmployeeDetail()
{
try
{
for (int counter = 0; counter < 5; counter++)
{
var emp = new Employee()
{
Name = "Eemployee " + counter,
Company = "Mindfire Solutions " + counter,
Description = "Software Engineer",
IsEmployeeRetired = false,
Country = "India",
};
using (var context = new DemoEntityContext())
{
context.Employees.Add(emp);
context.SaveChanges();
}
}
}
catch (Exception)
{
throw;
}
return Json(true);
}
Above action code will insert some demo record to Employee Table
public ActionResult GetEmployeeDetails()
{
var model = new List<EmployeeModel>();
try
{
using (var context = new DemoEntityContext())
{
var value = context.Employees.ToList();
foreach (var employee in value)
{
var empModel = new EmployeeModel();
empModel.EmployeeName = employee.Name;
empModel.Company = employee.Company;
empModel.Country = employee.Country;
empModel.Description = employee.Description;
empModel.IsEmployeeRetired = employee.IsEmployeeRetired? "Yes": "No";
model.Add(empModel);
}
}
}
catch (Exception)
{
throw;
}
return PartialView("_EmployeeDetailView", model);
}
this method will simply get the employee details and pass to partial view
c) Partial view “_EmployeeDetailView.cshtml”
@model List<CodeFirstWebApp.Models.EmployeeModel>
@{
Layout = null;
}
<h1>Employee Informations</h1>
@if (Model != null)
{
<div>
<table cellspacing="0"width="50%" border="1">
<thead>
<tr>
<th>
Employee Name
</th>
<th>
Company Name
</th>
<th>
Department
</th>
<th>
Employee Retired
</th>
<th>
Country
</th>
</tr>
</thead>
<tbody>
@foreach (var employee in Model)
{
<tr>
<td align="center">
@employee.EmployeeName
</td>
<td align="center">
@employee.Company
</td>
<td align="center">
@employee.Description
</td>
<td align="center">
@employee.IsEmployeeRetired
</td>
<td align="center">
@employee.Country
</td>
</tr>
}
</tbody>
</table>
</div>
}
d) EmployeeModel.cs : - model class to represent employee detail
public class EmployeeModel
{
public string EmployeeName { get; set; }
public string Description { get; set; }
public string Country { get; set; }
public string IsEmployeeRetired { get; set; }
public string Company { get; set; }
}
II) Add reference of entity framework, model class projects “CodeFirstModel” and “CodeFirstDataAccess”.
Now we are almost done with our demo project. Refer below screen shot for final structure of the solution.

III) And set the project as “set as startup project”.
But the Question is, where is our database? code-first default convention (means if no any config is set) which looks in the local SQL Server Express instance (localhost\SQLEXPRESS) for a database matching the fully qualified name of the context class (ProjectName.COntextClassName) i.e ( CodeFirstDataAccess.DemoEntityContext ). Not finding one, Code First creates the database and then uses the model it discovered by convention to build the tables and columns of the database. Refer screen shot below- Image itself describe how database initialization done in code-first approach.

In our demo we will locate the database location using configuration file – because this approach is quite useful when we want to deploy our application in different environment. In this case Code First convention looks exactly which database to use for a particular context, using the DbContext constructors or your application configuration file.
Web.config
<connectionStrings>
<add name="DbConnectionString" providerName="System.Data.SqlClient"
connectionString="Server=.; Database=EmployeeDB; Trusted_Connection=true" />
</connectionStrings>
DbConnectionString - Connection string name we used above. Using this Code-first will creat “EmployeeDB” as database name in the local sql server.
Wow Now we are ready to build our first code-first demo application.
Hit F5 to run the application: observation
1) An index page will display, then press button "InsertRecord". Now check local db you will find database is created with name “EmployeeDB” along with Table named as “Employee” and some record.

2) Press button “DisplayRecord”, hmm this is our final result.
