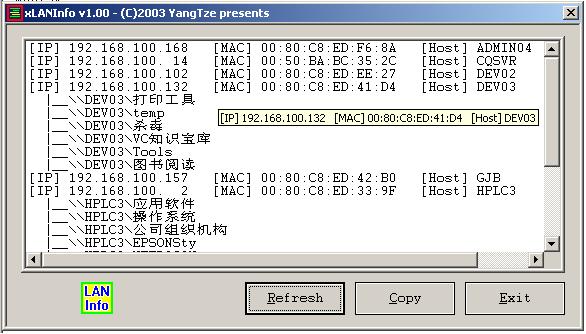
Introduction
xLANInfo is a useful tool for getting some information about LAN, such as remote-machine's IP/MAC/Host name/Shared resources.
Key code fragment
#include <Winsock2.h>
#include < Iphlpapi.h >
#pragma comment( lib,"Ws2_32.lib" )
#pragma comment( lib,"Mpr.lib" )
#pragma comment( lib,"iphlpapi.lib" )
void CXLANInfoDlg::OnGetLanInfo()
{
BOOL bCopyList = FALSE;
m_list.ResetContent();
CWaitCursor wait;
CString strTemp = _T("");
CString strTemp1 = _T("");
CString strSubResource = _T("");
struct hostent *host;
struct in_addr *ptr;
DWORD dwScope = RESOURCE_CONTEXT;
NETRESOURCE *NetResource = NULL;
HANDLE hEnum;
WSADATA wsaData;
WSAStartup( MAKEWORD( 1,1 ),&wsaData );
WNetOpenEnum( dwScope,NULL,NULL,NULL,&hEnum );
if( hEnum ) {
DWORD Count = 0xFFFFFFFF;
DWORD BufferSize = 2048;
LPVOID Buffer = new char[ 2048 ];
WNetEnumResource( hEnum,&Count,Buffer,&BufferSize );
NetResource = ( NETRESOURCE * )Buffer;
for( unsigned int i = 0; i < Count; i++, NetResource++ ) {
m_list.UpdateWindow();
if( NetResource->dwUsage == RESOURCEUSAGE_CONTAINER &&
NetResource->dwType == RESOURCETYPE_ANY ) {
if( NetResource->lpRemoteName ) {
{
strSubResource.Empty();
BOOL ret = GetSubResource( NetResource,
strSubResource );
}
CString strFullName = NetResource->lpRemoteName;
if( 0 == strFullName.Left( 2 ).Compare( "\\\\" ) )
strFullName = strFullName.Right(
strFullName.GetLength() - 2 );
host = gethostbyname( strFullName );
if( host == NULL ) continue;
ptr = ( struct in_addr * )host->h_addr_list[ 0 ];
int a = ptr->S_un.S_un_b.s_b1;
int b = ptr->S_un.S_un_b.s_b2;
int c = ptr->S_un.S_un_b.s_b3;
int d = ptr->S_un.S_un_b.s_b4;
strTemp.Format( _T("%d.%d.%d.%d"),a,b,c,d );
GetRemoteMAC( strTemp,strTemp1 );
if( strTemp1 != _T("Hello,YangTze!") ) {
strTemp.Format(
_T("[IP] %3d.%3d.%3d.%3d [MAC] %s [Host] %s"),
a,b,c,d,strTemp1,strFullName );
}
else {
strTemp.Format(
_T("[IP] %3d.%3d.%3d.%3d [Host] %s"),
a,b,c,d,strFullName );
}
int index = m_list.AddStringEx( strTemp );
m_list.SetCurSel( index );
if( !strSubResource.IsEmpty() ) {
int at = 0;
while( 1 ) {
m_list.UpdateWindow();
at = strSubResource.Find( '?',0 );
if( at == -1 ) break;
strTemp = " |__";
strTemp += strSubResource.Left( at );
int index = m_list.AddStringEx( strTemp );
m_list.SetCurSel( index );
strSubResource = strSubResource.Right(
strSubResource.GetLength()-at-1 );
}
}
bCopyList = TRUE;
}
}
}
delete Buffer;
WNetCloseEnum( hEnum );
}
WSACleanup();
CButton *pCopyListBtn = ( CButton * )GetDlgItem( IDC_COPY_LANINFO );
pCopyListBtn->EnableWindow( bCopyList );
}
void CXLANInfoDlg::GetRemoteMAC(CString &IP, CString &strMAC)
{
HRESULT hr;
IPAddr ipAddr;
ULONG pulMac[ 2 ];
ULONG ulLen;
CString tmp0,tmp1;
strMAC = _T("Hello,YangTze!");
ipAddr = inet_addr( ( char * )( LPCTSTR )IP );
memset( pulMac,0xff,sizeof( pulMac ) );
ulLen = 6;
hr = SendARP( ipAddr,0,pulMac,&ulLen );
if( NO_ERROR != hr || ulLen == 0 ) return;
ULONG i;
char * szMac = new char[ ulLen * 3 ];
PBYTE pbHexMac = ( PBYTE )pulMac;
for( i = 0; i < ulLen - 1; ++ i ) {
tmp0.Format( "%02X:",pbHexMac[ i ] );
tmp1 += tmp0;
}
tmp0.Format( "%02X",pbHexMac[ i ] );
tmp1 += tmp0;
delete [] szMac;
strMAC = tmp1;
}
BOOL CXLANInfoDlg::GetSubResource(NETRESOURCE *pContainer, CString &strRet)
{
NETRESOURCE NetResource;
NETRESOURCE *pSubNetResource = NULL;
HANDLE hEnum = NULL;
DWORD ret;
CopyMemory( ( PVOID )&NetResource,( PVOID )pContainer,
sizeof( NETRESOURCE ) );
NetResource.dwScope = RESOURCE_GLOBALNET;
NetResource.dwType = RESOURCETYPE_ANY;
NetResource.dwUsage = RESOURCEUSAGE_CONTAINER;
ret = WNetOpenEnum( RESOURCE_GLOBALNET,
RESOURCETYPE_ANY,0,&NetResource,&hEnum );
if( NO_ERROR != ret ) return FALSE;
if( hEnum ) {
DWORD Count = 0xFFFFFFFF;
DWORD BufferSize = 2048;
LPVOID Buffer = new char[ 2048 ];
ret = WNetEnumResource( hEnum,&Count,Buffer,&BufferSize );
pSubNetResource = ( NETRESOURCE * )Buffer;
if( NO_ERROR == ret ) {
CString tmp;
for( unsigned int i = 0; i < Count; i++, pSubNetResource++ ) {
m_list.UpdateWindow();
if( pSubNetResource->lpRemoteName ) {
tmp.Format( "%s?",pSubNetResource->lpRemoteName );
strRet += tmp;
}
}
}
delete Buffer;
WNetCloseEnum( hEnum );
}
return TRUE;
}
Thanks
Thanks for your review!
History
- First written - By YangTze, 11/26/2K3