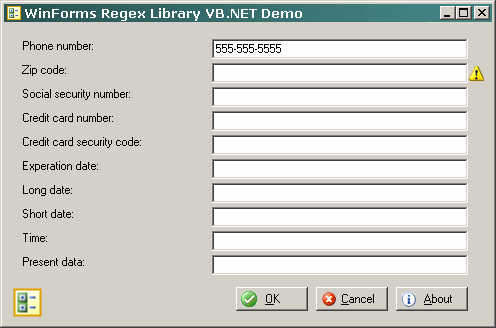
Introduction
WinFormsRegexLibrary is a regular expression validation library for Windows Forms. It provides validation methods for a number of different data, such as, phone numbers, Zip codes, email addresses, URLs, IP addresses, credit card numbers, date's, times, etc. The library is written in C#, and the demo is written in VB.NET. I will be posting a version of the library written in VB.NET, and a demo written in C#, soon. The concepts of using the library are the same, in either language.
Using the code
To use the library, the first thing you need to do is to copy the WinFormsRegexLibrary.xml file to your application's bin directory, in-order for the XML documentation comments to show up. Then add a reference to the WinFormsRegexLibrary.dll assembly. Then add an ErrorProvider
control to your form, where you need validation. Then to use it in your code, you type the class name; RegexValidator
, followed by the dot operator (.
), and the name of the method you wish to use for validation.
Each method accepts two parameters, the first one being the control to validate, and the second one being the ErrorProvider
to use for displaying an error message when incorrect data is entered. Optionally, some methods, such as the IsPresent
method, also accepts a string for the name of the field to validate. This is used in the error message so the user knows which control is required, etc.
using WinFormsRegexLibrary;
public class MainForm : System.Windows.Form
{
public MainForm()
{
}
public static void Main()
{
Application.Run(new MainForm());
}
private void textBox1_Validating(object sender, CancelEventArgs e)
{
if (RegexValidator.IsUSPhoneNumber(textBox1, errorProvider1)
{
e.Cancel = false;
}
else
{
e.Cancel = true;
}
}
private void textBox1_TextChanged(object sender, EventArgs e)
{
if (RegexValidator.IsUSPhoneNumber(textBox1, errorProvider1);
{
return true;
}
else
{
return false;
}
}
}
Imports WinFormsRegexLibrary
Public Class MainForm
Private Sub TextBox1_Validating(ByVal sender As Object, _
ByVal e As System.ComponentModel.CanelEventArgs)
If RegexValidator.IsUsPhonenUmber(TextBox1, ErrorProvider1) Then
e.Canel = False
Else
e.Cancel = True
End If
End Sub
Private Sub TextBox1_TextChanged(ByVal sender As Object, _
ByVal e As System.EventArgs)
If RegexValidator.IsUSPhoneNumber(TextBox1, ErrorProvider1) Then
Return True
Else
Return False
End If
End Sub
End Class
Points of Interest
This library has a lot of validation methods, and I will keep adding to it. If you know of some common uses of regular expression validation that you would like to see in this library, please email me at codeintellects@msn.com.
History
- Version 1.1 - No bugs, all expressions fully tested and working.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.