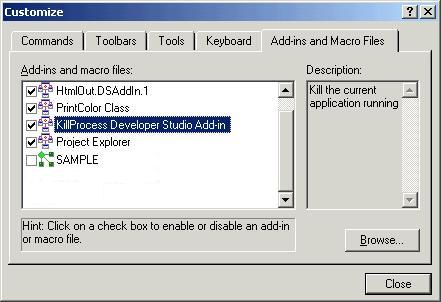
Introduction
How many times have you been in the situation, during development, you had to kill the current project process
for any reason (infinite loops, crazy threads, a wrong return value and so on) ? It happens
continuously, I guess.
What to do then? I always have the Task Manager minimized to the System Tray and when I need to kill the project
process I click it up, scrolling the tasks list, found mine end kill it. Too much time wasted! ... :)
So it came to my mind to simplify the killing procedure, making it as fastest as possible: Why not having a button in the IDE
to do the job for us? An Add-In would help. So here it is.
Background
To understand the source code, it is just needed to read any other Add-in article in this CodeProject section.
The code
The DevStudio Add-Ins Wizard does the main job for us to crate an Add-In, in fact the code you have to implement is
simply the one relative to what the Add-In you're creating stands for: in this case killing the active project running process.
The method to implement is ICommand::KillProcessCommandMethod()
STDMETHODIMP CCommands::KillProcessCommandMethod()
{
AFX_MANAGE_STATE(AfxGetStaticModuleState());
USES_CONVERSION;
CComPtr<IDispatch> iDisp=NULL;
m_pApplication->get_ActiveProject(&iDisp);
CComQIPtr<IGenericProject, &__uuidof(IGenericProject)> pProject(iDisp);
if(pProject == NULL)
return S_OK;
CComBSTR bstrName=NULL;
pProject->get_Name(&bstrName);
bstrName+=".exe";
LPCTSTR stringa = OLE2T(bstrName);
LPSTR str = (LPSTR)stringa;
int len = strlen(str);
if(len > 15)
{
for(int i = 15; i < len; i++)
if(*(str + i)!=NULL)
*(str + i) = NULL;
}
HANDLE hSnapShot=CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS,0);
PROCESSENTRY32* processInfo=new PROCESSENTRY32;
processInfo->dwSize=sizeof(PROCESSENTRY32);
DWORD processID = 0;
BOOL bFound = FALSE;
while(Process32Next(hSnapShot,processInfo)!=FALSE)
{
processID = processInfo->th32ProcessID;
if(strcmp(processInfo->szExeFile,str) == 0)
{
bFound = TRUE;
break;
}
}
CloseHandle(hSnapShot);
if(bFound)
{
HANDLE hProcess = OpenProcess(PROCESS_ALL_ACCESS,TRUE,processID);
if(hProcess != NULL)
TerminateProcess(hProcess,0);
}
return S_OK;
}
Hints
Remember to include in StdAfx.h the following header files:
#include <comdef.h>
#include <winbase.h>
#include <tlhelp32.h>
Copy the KillProcess.dll
in the <Visual Studio Install Dir>\Common\MSDev98\Addins folder
Limitations
As stated in the code comments, we forced the name of the executable to be the same as the project one, plus an '.exe' extension.
this is not very portable. I tried to find through the
IConfiguration
interface or
IBuildProject
interface to obtain the name of the
executable to be produced but maybe I followed the wrong way. If you have any hint or comment are always welcome. Thanks. Fabio
History
Worked as a Java developer targeting J2EE and J2SE for half a decade.
Now he's employed for Avanade as a Senior Associate Consultant on Microsoft technologies.
His hobbies are Win32 programming using SDK, MFC, ATL and COM; playing guitar and listening to music.