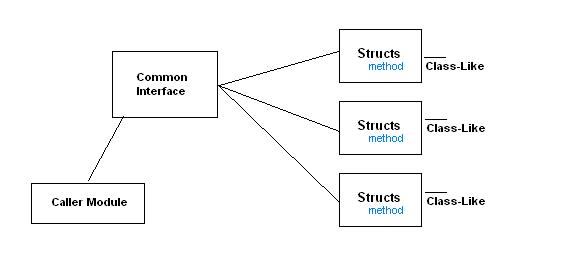
Introduction
With the change of solution methods of real-life problems and designed architectures,
software projects started to be developed using object oriented programming instead of
structural programming. Powerful structural languages such as C has become less popular
in large scale projects due to difficulties of adapting structural solutions for
maturity factors(reusability, extendibility etc...) .We discuss adaptation of an
OOP concept polymorphism to structural languages.
Background
Due to OOP is completely a different approach according to Structural programming,
it comes with many programming technics different than Structural programming. One of
these technics is polymorphism. Polymorphism simply means "common interface, specific
implementation", deeply means each class has its own implementation while they share a
common interface. This concept brings benefits to object oriented programming such as
combining all objects behaviours in a standard interface and applying it with all classes.
As an OOP concept, can this feature be implemented in a structural language such as C?
C provides powerful programming abilities like pointers and structures. And by using these
elements, polymorphism model can be partially implemented.
Using the code
This code is only a sample for what you can do in C, in polymorphism manner. So code is
small and to explain how it works, lots of comments has been added. Also other tips are
given how you can make structural concept similar to object oriented.
First we should have a structure that acts like class and related functions
#ifndef _POLY_STRUC_H
#define _POLY_STRUC_H
struct POLY_STRUCT
{
void (*poly)( ); };
struct POLY_STRUCT *InitPoly( void (*poly)() );
void DestroyPoly( struct POLY_STRUCT *poly );
void Run_Poly_Method( struct POLY_STRUCT *poly );
#endif
In this part, I implemented typical functions that initializes, destroys
struct and calls typical polymorphic method. In other scenarios, this
methods can change or be extended.
#include <stdlib.h>
#include "POLY_STRUC.H"
struct POLY_STRUCT *InitPoly( void (*poly)() )
{
struct POLY_STRUCT *result = (struct POLY_STRUCT*)
malloc( sizeof( struct POLY_STRUCT ) );
if(result != NULL)
result->poly = poly;
return result;
}
void DestroyPoly( struct POLY_STRUCT *poly )
{
if(poly != NULL) free(poly);
}
void Run_Poly_Method( struct POLY_STRUCT *poly_s )
{
(* ( poly_s->poly ) ) ();
}
</stdlib.h>
And the sample main class to see how it works:
#include <stdio.h>
#include <stdlib.h>
#include "POLY_STRUC.H"
struct POLY_STRUCT* example_poly1;
struct POLY_STRUCT* example_poly2;
void poly_example1_method(){printf("EXAMPLE1\n");}
void poly_example2_method(){printf("EXAMPLE2\n");}
int main(int argc, char *argv[])
{
example_poly1 = InitPoly(&poly_example1_method);
example_poly2 = InitPoly(&poly_example2_method);
Run_Poly_Method(example_poly1);
Run_Poly_Method(example_poly2);
DestroyPoly(example_poly1);
DestroyPoly(example_poly2);
return 0;
}
</stdlib.h></stdio.h>
Conclusion
In adapting polymorphism, function pointers
plays
the big role for the design. Also using struct
s is
important for creating class oriented models. But, in the current
circumstances we can not apply encapsulation in C(no private or
protected modifiers).
It's obvious that polymorphism is more effective when it's
used in OOP(with inheritance
or composition
).
In this article, our goal is to use object oriented aspects in a structural
language as much as we can ,to use advantages of it. But lack of dynamic
binding, it's sure static and hard-coded.
What about the advantages and disadvantages? Trade-off between
performance and problem-solution benefits(Using an object oriented
design in projects simplifies solutions also increases reusability
and extendibility) is main design consideration in this article.
Performance impact is obvious. Calling functions by function-pointers
is relatively slow. Also accessing struct members makes execution slower.
But in the other side reusability and extendibility gain is sure obvious.
Adapting polymorphism brings some extra code implementations that makes
relatively small projects more complex and unreadable. It's important to use
polymorphism in big-scale projects(operating systems,hardware implementations etc...)
to use it efficiently. But this disadvantage turns into an advantage when used in large scale projects.
Points of Interest
This was a quite different experience , like using C as C++.I know it's
very important to use object oriented discrete from structural approach, but also
it's also important to see the similarities. For a long time, I developed projects with
object-oriented languages (C++, C#, JAVA) , using C again was quite enjoying. I think
I'll continue enhancing this concept by adding other auxiliary methods.
History
Version 1.0 - Initial Version