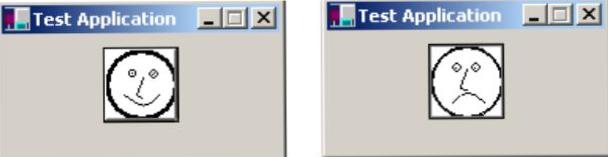
This article is about writing a customized Button
control that displays different images when a user moves the mouse over the button, clicks on the button and leaves the button. This control is exactly like CBitmapButton
control class available in MFC
. The demo project included with this article has a very simple implementation of a derived class from the Button
class.
For every control in Windows, events are fired by the framework for different actions taken by a user in the GUI application. This gives the application a chance to respond to these events and act according to the design and requirements of that control or window. This goes for the Button
control too. The Button
class is derived from the ButtonBase
class. This base class encapsulates most of the typical implementation of event handling. If you do not want to do any customized work on the control, then the base class will handle all of the events for us. Since we want to draw different images on the button for different user actions, the derived class will have to handle the painting of the button window. In the .NET Framework, drawing an image on a button control is as simple as attaching an image file resource as one of the properties of the Button
class. The property is BackgroundImage
. If you do not want to change the image for different actions, then you do not need to derive any special class from base class; in the application’s form, set that property and you are done. For accomplishing drawing of different images, you can make use of another property of the Button
class, ImageList
. Yes, The ImageList
class is very much like the CImageList
class in MFC. You can add different images to this list. You can attach an image list to the Button
control, and then assign the index of the image in the list to the Button
control. The .NET Framework will draw that image on the control. You can make use of this concept to change the image on the button corresponding to different actions. The ButtonBase
class has a bunch of overridable virtual
functions that get called when the mouse moves over the control, a button is clicked or a button goes into the raised position. These methods are PaintDown
, PaintRaised
and PaintOver
. You can supply your own implementation in the derived class to handle these events. Just make sure that you call the base class' method too.
The implementation of derived control is as follows:
public class NKBitmapButton : Button
{
public NKBitmapButton()
{
}
protected override Rectangle OverChangeRectangle
{
get
{
return base.ClientRectangle;
}
}
protected override void PaintDown (PaintEventArgs pevent, int borderWidth)
{
ImageIndex = 1;
base.PaintDown (pevent, borderWidth);
}
protected override void PaintOver (PaintEventArgs pevent)
{
ImageIndex = 0;
base.PaintOver (pevent);
}
protected override void PaintRaised (PaintEventArgs pevent, int borderWidth)
{
ImageIndex = 0;
base.PaintRaised (pevent, borderWidth);
}
}
There is a bug in the .NET framework. The PaintOver
method does not get called when the mouse moves over the button control. It only gets called after you have clicked on the button. Therefore I have set the image index back to the one that gets displayed when the button is in the normal raised position.
How To Use The NKBitmapButton Class
In the windows application, add a button. The Wizard will add an entry into the InitializeComponent
method of the Form
class. Change the variable type from System.WinForms.Button
to NetGUIGoodies.NKBitmapButton
. Add an image list to your form. Create two bitmaps of size 48x48 and add them to the list. The image at index 0 will be drawn when the button is in normal raised position and the image at index 1 will be drawn when you click on the button and it is in the down position. To keep the implementation simple, I have not added properties or methods to the derived button class to specify size and indices of the images.
private NetGUIGoodies.NKBitmapButton PictureButton;
private void InitializeComponent()
{
this.PictureButton = new NetGUIGoodies.NKBitmapButton ();
this.ButtonImageList = new System.WinForms.ImageList ();
.
.
PictureButton.ImageList = this.ButtonImageList;
PictureButton.ImageIndex = 0;
.
.
}
License
This article has no explicit license attached to it, but may contain usage terms in the article text or the download files themselves. If in doubt, please contact the author via the discussion board below. A list of licenses authors might use can be found here.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.