Improved Facade Design Pattern in Automation Testing v.2.0





5.00/5 (3 votes)
Explains in details how to use the Facade Design Pattern in automation tests so that its class to follow the dependency inversion principle.
Introduction
The today’s article is dedicated once again to the Facade Design Pattern. In my previous article on the matter, I showed you how you can utilize the pattern to build more readable tests. Here I am going to present you how to improve further the usage of the facade design pattern. The new version is going to follow the Dependency Inversion Principle. This way you can bring even more abstraction to your test framework and make your tests even more maintainable.

Definition Recall
A facade is an object that provides a simplified interface to a larger body of code, such as a class library. It makes a software library easier to use and understand, is more readable, and reduces dependencies on external or other code.
How not to Use Facade Design Pattern
A couple of years ago when I started to use the facade design pattern, I didn’t apply it correctly. The main problem that my teammates and I tried to solve back then was the lack of code reuse in our tests. We succeeded in our quest, and our code started to follow one of the main superb programming principles- DRY Don’t Repeat Yourself.
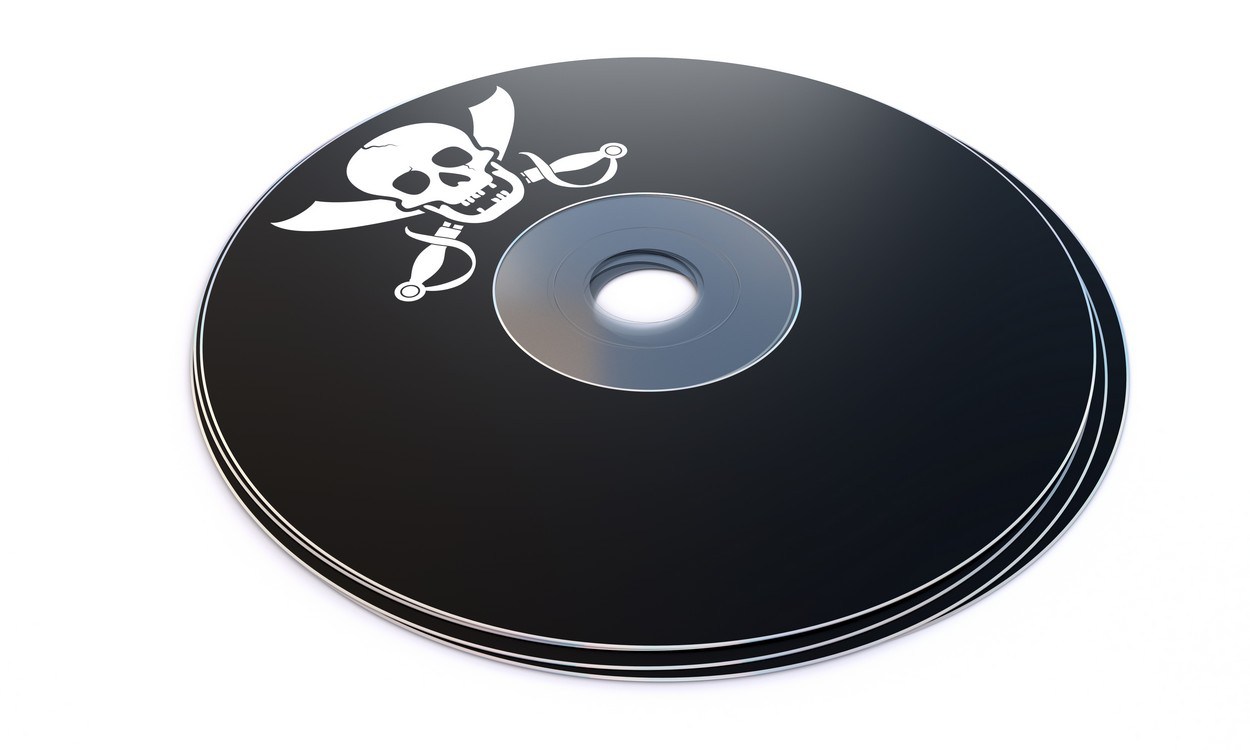
Don’t Repeat Yourself Principle (DRY)
The developer who learns to recognize duplication, and understands how to eliminate it through appropriate practice and proper abstraction, can produce much cleaner code than one who continuously infects the application with unnecessary repetition. Duplication results in increased probability of bugs and adds complexity to the system. Moreover, duplication makes the system more difficult to understand and maintain.
However, there was one significant problem with the initial version of our facades. Back then we were still not using the Page Object Pattern. As a result, the size of our facades’ files got enormous, like thousands of lines of code.

In the presented example over 700 lines. This happened because all pages’ elements and framework’s stuff were hidden there.
Because of the large files we had to use a special language feature to solve this. This feature creates regions in the file that you can collapse or expand in the editor.
Initial Version Facade Design Pattern
Below you can find the previously presented example regarding the correct usage of the facade design pattern. The tests’ logic/workflow is encapsulated in the PurchaseFacade class.
In case the workflow of the test case is changed, it can be quickly updated only in a single place. Or if you want to add additional assertions, they can be added to the PurchaseItem method.
Improved Version Facade Design Pattern
The only issue of the previously presented code is that it doesn’t follow the Dependency Inversion Principle.

Dependency Inversion Principle
It suggests that our high-level components (the facades) should not depend on our low-level components (the pages); rather, they should both depend on abstractions.
Find below, the code of the improved version of the facade that holds the logic related to the creation of purchases.
Through the usage of the pages’ interfaces, the façade follows the Dependency Inversion Principle. You can replace the version of some of the pages without changing even a single line of code in the façades.
The facade combines the different pages’ methods to complete the wizard of the order. If there is a change in the order of the executed actions you can edit it only here. It will apply to tests that are using the facade. The different test cases are accomplished through the various parameters passed to the facade’s methods. You can read how to create these types of pages in my article Page Objects That Make Code More Maintainable
These types of facades contain a much less code because most of the logic is held by the pages instead of the facade itself.
Finally, I want to allude briefly to the name of the facade. It doesn’t contain the word Facade in its name because it should not suggest that it is hiding a complex logic.
So Far in the "Design Patterns in Automated Testing" Series
- Page Object Pattern
- Advanced Page Object Pattern
- Facade Design Pattern
- Singleton Design Pattern
- Fluent Page Object Pattern
- IoC Container and Page Objects
- Strategy Design Pattern
- Advanced Strategy Design Pattern
- Observer Design Pattern
- Observer Design Pattern via Events and Delegates
- Observer Design Pattern via IObservable and IObserver
- Decorator Design Pattern- Mixing Strategies
- Page Objects That Make Code More Maintainable
- Improved Facade Design Pattern in Automation Testing v.2.0
- Rules Design Pattern
- Specification Design Pattern
- Advanced Specification Design Pattern
If you enjoy my publications, feel free to SUBSCRIBE
Also, hit these share buttons. Thank you!
Source Code
References:
- SOLID Principles
- An Absolute Beginner’s Tutorial on Dependency Inversion Principle, Inversion of Control and Dependency Injection
- The Don’t-Repeat-Yourself (DRY) design principle in .NET
- Page Objects That Make Code More Maintainable
The post Improved Facade Design Pattern in Automation Testing v.2.0 appeared first on Automate The Planet.
All images are purchased from DepositPhotos.com and cannot be downloaded and used for free.
License Agreement