As a developer, you might have applications that use NoSQL document data. You can use a SQL API account in Azure Cosmos DB to store and access this document data. This tutorial shows you how to build a Node.js console application to create Azure Cosmos DB resources and query them.
In this tutorial, you will:
- Create and connect to an Azure Cosmos DB account.
- Set up your application.
- Create a database.
- Create a container.
- Add items to the container.
- Perform basic operations on the items, container, and database.
Prerequisites
Make sure you have the following resources:
Create Azure Cosmos DB account
Let's create an Azure Cosmos DB account. If you already have an account you want to use, you can skip ahead to Set up your Node.js application. If you are using the Azure Cosmos DB Emulator, follow the steps at Azure Cosmos DB Emulator to set up the emulator and skip ahead to Set up your Node.js application.
- In a new browser window, sign in to the Azure portal.
-
Select Create a resource > Databases > Azure Cosmos DB.
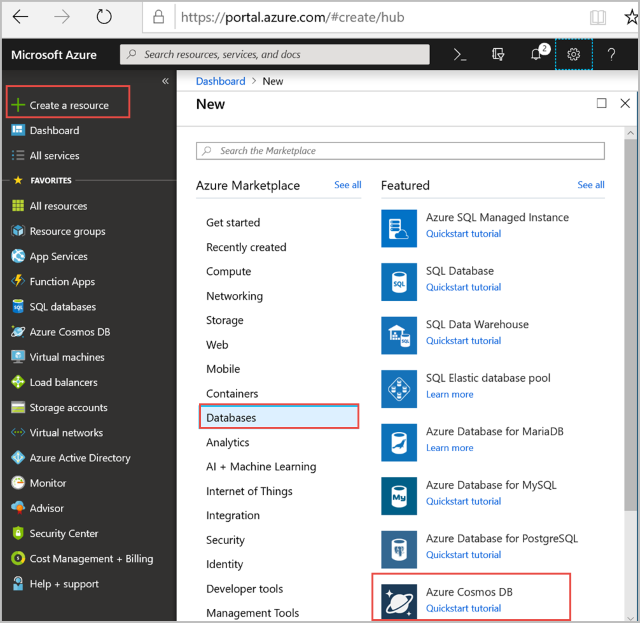
-
On the Create Azure Cosmos DB Account page, enter the basic settings for the new Azure Cosmos DB account.
Setting | Value | Description |
Subscription | Your subscription | Select the Azure subscription that you want to use for this Azure Cosmos DB account. |
Resource Group | Create new
Then enter the same unique name as provided in ID | Select Create new. Then enter a new resource-group name for your account. For simplicity, use the same name as your ID. |
Account Name | Enter a unique name | Enter a unique name to identify your Azure Cosmos DB account. Because documents.azure.com is appended to the ID that you provide to create your URI, use a unique ID.
The ID can only contain lowercase letters, numbers, and the hyphen (-) character. It must be between 3 and 31 characters in length. |
API | Core(SQL) | The API determines the type of account to create. Azure Cosmos DB provides five APIs: Core(SQL) for document databases, Gremlin for graph databases, MongoDB for document databases, Azure Table, and Cassandra. Currently, you must create a separate account for each API.
Select Core(SQL) because in this article you create a document database and query by using SQL syntax.
Learn more about the SQL API. |
Location | Select the region closest to your users | Select a geographic location to host your Azure Cosmos DB account. Use the location that's closest to your users to give them the fastest access to the data. |
Select Review+Create. You can skip the Network and Tags section.

-
The account creation takes a few minutes. Wait for the portal to display the Congratulations! Your Azure Cosmos DB account was created page.

Set up your Node.js application
Before you start writing code to build the application, you can build the framework for your app. Run the following steps to set up your Node.js application that has the framework code:
- Open your favorite terminal.
- Locate the folder or directory where you'd like to save your Node.js application.
-
Create two empty JavaScript files with the following commands:
-
Windows:
fsutil file createnew app.js 0
fsutil file createnew config.js 0
-
Linux/OS X:
touch app.js
touch config.js
-
Install the @azure/cosmos module via npm. Use the following command:
npm install @azure/cosmos --save
Set your app's configurations
Now that your app exists, you need to make sure it can talk to Azure Cosmos DB. By updating a few configuration settings, as shown in the following steps, you can set your app to talk to Azure Cosmos DB:
-
Open config.js in your favorite text editor.
-
Copy and paste the code snippet below and set properties config.endpoint
and config.primaryKey
to your Azure Cosmos DB endpoint URI and primary key. Both these configurations can be found in the Azure portal.

var config = {}
config.endpoint = "~your Azure Cosmos DB endpoint uri here~";
config.primaryKey = "~your primary key here~";
-
Copy and paste the database
, container
, and items
data to your config
object below where you set your config.endpoint
and config.primaryKey
properties. If you already have data you'd like to store in your database, you can use the Data Migration tool in Azure Cosmos DB rather than defining the data here.
var config = {}
config.endpoint = "~your Azure Cosmos DB account endpoint uri here~";
config.primaryKey = "~your primary key here~";
config.database = {
"id": "FamilyDatabase"
};
config.container = {
"id": "FamilyContainer"
};
config.items = {
"Andersen": {
"id": "Anderson.1",
"lastName": "Andersen",
"parents": [{
"firstName": "Thomas"
}, {
"firstName": "Mary Kay"
}],
"children": [{
"firstName": "Henriette Thaulow",
"gender": "female",
"grade": 5,
"pets": [{
"givenName": "Fluffy"
}]
}],
"address": {
"state": "WA",
"county": "King",
"city": "Seattle"
}
},
"Wakefield": {
"id": "Wakefield.7",
"parents": [{
"familyName": "Wakefield",
"firstName": "Robin"
}, {
"familyName": "Miller",
"firstName": "Ben"
}],
"children": [{
"familyName": "Merriam",
"firstName": "Jesse",
"gender": "female",
"grade": 8,
"pets": [{
"givenName": "Goofy"
}, {
"givenName": "Shadow"
}]
}, {
"familyName": "Miller",
"firstName": "Lisa",
"gender": "female",
"grade": 1
}],
"address": {
"state": "NY",
"county": "Manhattan",
"city": "NY"
},
"isRegistered": false
}
};
JavaScript SDK uses the generic terms container and item. A container can be a collection, graph, or table. An item can be a document, edge/vertex, or row, and is the content inside a container.
-
Finally, export your config
object, so that you can reference it within the app.js file.
},
"isRegistered": false
}
};
module.exports = config;
Connect to an Azure Cosmos DB account
-
Open your empty app.js file in the text editor. Copy and paste the code below to import the @azure/cosmos
module and your newly created config
module.
const CosmosClient = require('@azure/cosmos').CosmosClient;
const config = require('./config');
const url = require('url');
-
Copy and paste the code to use the previously saved config.endpoint
and config.primaryKey
to create a new CosmosClient
.
const url = require('url');
const endpoint = config.endpoint;
const masterKey = config.primaryKey;
const client = new CosmosClient({ endpoint: endpoint, auth: { masterKey: masterKey } });
Now that you have the code to initialize the Azure Cosmos DB client, let's take a look at how to work with Azure Cosmos DB resources.
Create a database
-
Copy and paste the code below to set the database ID, and the container ID. These IDs are how the Azure Cosmos DB client will find the right database and container.
const client = new CosmosClient({ endpoint: endpoint, auth: { masterKey: masterKey } });
const HttpStatusCodes = { NOTFOUND: 404 };
const databaseId = config.database.id;
const containerId = config.container.id;
A database can be created by using either the createIfNotExists
or create function of the Databases class. A database is the logical container of items partitioned across containers.
-
Copy and paste the createDatabase
and readDatabase
methods into the app.js file under the databaseId
and containerId
definition. The createDatabase
function will create a new database with id FamilyDatabase
, specified from the config
object if it does not already exist. The readDatabase
function will read the database's definition to ensure that the database exists.
async function createDatabase() {
const { database } = await client.databases.createIfNotExists({ id: databaseId });
console.log(`Created database:\n${database.id}\n`);
}
async function readDatabase() {
const { body: databaseDefinition } = await client.database(databaseId).read();
console.log(`Reading database:\n${databaseDefinition.id}\n`);
}
-
Copy and paste the code below where you set the createDatabase
and readDatabase
functions to add the helper function exit
that will print the exit message.
function exit(message) {
console.log(message);
console.log('Press any key to exit');
process.stdin.setRawMode(true);
process.stdin.resume();
process.stdin.on('data', process.exit.bind(process, 0));
};
-
Copy and paste the code below where you set the exit
function to call the createDatabase
and readDatabase
functions.
createDatabase()
.then(() => readDatabase())
.then(() => { exit(`Completed successfully`); })
.catch((error) => { exit(`Completed with error \${JSON.stringify(error)}`) });
At this point, your code in app.js should now look as following code:
const CosmosClient = require('@azure/cosmos').CosmosClient;
const config = require('./config');
const url = require('url');
const endpoint = config.endpoint;
const masterKey = config.primaryKey;
const client = new CosmosClient({ endpoint: endpoint, auth: { masterKey: masterKey } });
const HttpStatusCodes = { NOTFOUND: 404 };
const databaseId = config.database.id;
const containerId = config.container.id;
async function createDatabase() {
const { database } = await client.databases.createIfNotExists({ id: databaseId });
console.log(`Created database:\n${database.id}\n`);
}
async function readDatabase() {
const { body: databaseDefinition } = await client.database(databaseId).read();
console.log(`Reading database:\n${databaseDefinition.id}\n`);
}
function exit(message) {
console.log(message);
console.log('Press any key to exit');
process.stdin.setRawMode(true);
process.stdin.resume();
process.stdin.on('data', process.exit.bind(process, 0));
}
createDatabase()
.then(() => readDatabase())
.then(() => { exit(`Completed successfully`); })
.catch((error) => { exit(`Completed with error ${JSON.stringify(error) }`) });
-
In your terminal, locate your app.js file and run the command:
node app.js
Create a container
Next create a container within the Azure Cosmos DB account, so that you can store and query the data.
Warning
Creating a container has pricing implications. Visit our pricing page so you know what to expect.
A container can be created by using either the createIfNotExists
or create function from the Containers
class. A container consists of items (which in the case of the SQL API is JSON documents) and associated JavaScript application logic.
-
Copy and paste the createContainer
and readContainer
function underneath the readDatabase
function in the app.js file. The createContainer
function will create a new container with the containerId
specified from the config
object if it does not already exist. The readContainer
function will read the container definition to verify the container exists.
async function createContainer() {
const { container } = await client.database(databaseId).containers.createIfNotExists({ id: containerId });
console.log(`Created container:\n${config.container.id}\n`);
}
async function readContainer() {
const { body: containerDefinition } = await client.database(databaseId).container(containerId).read();
console.log(`Reading container:\n${containerDefinition.id}\n`);
}
-
Copy and paste the code underneath the call to readDatabase
to execute the createContainer
and readContainer
functions.
createDatabase()
.then(() => readDatabase())
.then(() => createContainer())
.then(() => readContainer())
.then(() => { exit(`Completed successfully`); })
.catch((error) => { exit(`Completed with error ${JSON.stringify(error)}`) });
At this point, your code in app.js should now look like this:
const CosmosClient = require('@azure/cosmos').CosmosClient;
const config = require('./config');
const url = require('url');
const endpoint = config.endpoint;
const masterKey = config.primaryKey;
const client = new CosmosClient({ endpoint: endpoint, auth: { masterKey: masterKey } });
const HttpStatusCodes = { NOTFOUND: 404 };
const databaseId = config.database.id;
const containerId = config.container.id;
async function createDatabase() {
const { database } = await client.databases.createIfNotExists({ id: databaseId });
console.log(`Created database:\n${database.id}\n`);
}
async function readDatabase() {
const { body: databaseDefinition } = await client.database(databaseId).read();
console.log(`Reading database:\n${databaseDefinition.id}\n`);
}
async function createContainer() {
const { container } = await client.database(databaseId).containers.createIfNotExists({ id: containerId });
console.log(`Created container:\n${config.container.id}\n`);
}
async function readContainer() {
const { body: containerDefinition } = await client.database(databaseId).container(containerId).read();
console.log(`Reading container:\n${containerDefinition.id}\n`);
}
function exit(message) {
console.log(message);
console.log('Press any key to exit');
process.stdin.setRawMode(true);
process.stdin.resume();
process.stdin.on('data', process.exit.bind(process, 0));
}
createDatabase()
.then(() => readDatabase())
.then(() => createContainer())
.then(() => readContainer())
.then(() => { exit(`Completed successfully`); })
.catch((error) => { exit(`Completed with error ${JSON.stringify(error)}`) });
-
In your terminal, locate your app.js file and run the command:
node app.js
Create an item
An item can be created by using the create function of the Items
class. When you're using the SQL API, items are projected as documents, which are user-defined (arbitrary) JSON content. You can now insert an item into Azure Cosmos DB.
-
Copy and paste the createFamilyItem
function underneath the readContainer
function. The createFamilyItem
function creates the items containing the JSON data saved in the config
object. We'll check to make sure an item with the same id does not already exist before creating it.
async function createFamilyItem(itemBody) {
try {
const { item } = await client.database(databaseId).container(containerId).item(itemBody.id).read();
console.log(`Item with family id ${itemBody.id} already exists\n`);
}
catch (error) {
if (error.code === HttpStatusCodes.NOTFOUND) {
const { item } = await client.database(databaseId).container(containerId).items.create(itemBody);
console.log(`Created family item with id:\n${itemBody.id}\n`);
} else {
throw error;
}
}
};
-
Copy and paste the code below the call to readContainer
to execute the createFamilyItem
function.
createDatabase()
.then(() => readDatabase())
.then(() => createContainer())
.then(() => readContainer())
.then(() => createFamilyItem(config.items.Andersen))
.then(() => createFamilyItem(config.items.Wakefield))
.then(() => { exit(`Completed successfully`); })
.catch((error) => { exit(`Completed with error ${JSON.stringify(error)}`) });
-
In your terminal, locate your app.js file and run the command:
node app.js
Query Azure Cosmos DB resources
Azure Cosmos DB supports rich queries against JSON documents stored in each container. The following sample code shows a query that you can run against the documents in your container.
-
Copy and paste the queryContainer
function below the createFamilyItem
function in the app.js file. Azure Cosmos DB supports SQL-like queries as shown below.
async function queryContainer() {
console.log(`Querying container:\n${config.container.id}`);
const querySpec = {
query: "SELECT VALUE r.children FROM root r WHERE r.lastName = @lastName",
parameters: [
{
name: "@lastName",
value: "Andersen"
}
]
};
const { result: results } = await client.database(databaseId).container(containerId).items.query(querySpec).toArray();
for (var queryResult of results) {
let resultString = JSON.stringify(queryResult);
console.log(`\tQuery returned ${resultString}\n`);
}
};
-
Copy and paste the code below the calls to createFamilyItem
to execute the queryContainer
function.
createDatabase()
.then(() => readDatabase())
.then(() => createContainer())
.then(() => readContainer())
.then(() => createFamilyItem(config.items.Andersen))
.then(() => createFamilyItem(config.items.Wakefield))
.then(() => queryContainer())
.then(() => { exit(`Completed successfully`); })
.catch((error) => { exit(`Completed with error ${JSON.stringify(error)}`) });
-
In your terminal, locate your app.js
file and run the command:
node app.js
Replace an item
Azure Cosmos DB supports replacing the content of items.
-
Copy and paste the replaceFamilyItem
function below the queryContainer
function in the app.js file. Note we've changed the property 'grade' of a child to 6 from the previous value of 5.
async function replaceFamilyItem(itemBody) {
console.log(`Replacing item:\n${itemBody.id}\n`);
itemBody.children[0].grade = 6;
const { item } = await client.database(databaseId).container(containerId).item(itemBody.id).replace(itemBody);
};
-
Copy and paste the code below the call to queryContainer
to execute the replaceFamilyItem
function. Also, add the code to call queryContainer
again to verify that item has successfully changed.
createDatabase()
.then(() => readDatabase())
.then(() => createContainer())
.then(() => readContainer())
.then(() => createFamilyItem(config.items.Andersen))
.then(() => createFamilyItem(config.items.Wakefield))
.then(() => queryContainer())
.then(() => replaceFamilyItem(config.items.Andersen))
.then(() => queryContainer())
.then(() => { exit(`Completed successfully`); })
.catch((error) => { exit(`Completed with error ${JSON.stringify(error)}`) });
-
In your terminal, locate your app.js
file and run the command:
node app.js
Delete an item
Azure Cosmos DB supports deleting JSON items.
-
Copy and paste the deleteFamilyItem
function underneath the replaceFamilyItem
function.
async function deleteFamilyItem(itemBody) {
await client.database(databaseId).container(containerId).item(itemBody.id).delete(itemBody);
console.log(`Deleted item:\n${itemBody.id}\n`);
};
-
Copy and paste the code below the call to the second queryContainer
to execute the deleteFamilyItem
function.
createDatabase()
.then(() => readDatabase())
.then(() => createContainer())
.then(() => readContainer())
.then(() => createFamilyItem(config.items.Andersen))
.then(() => createFamilyItem(config.items.Wakefield))
.then(() => queryContainer
())
.then(() => replaceFamilyItem(config.items.Andersen))
.then(() => queryContainer())
.then(() => deleteFamilyItem(config.items.Andersen))
.then(() => { exit(`Completed successfully`); })
.catch((error) => { exit(`Completed with error ${JSON.stringify(error)}`) });
-
In your terminal, locate your app.js file and run the command:
node app.js
Delete the database
Deleting the created database will remove the database and all children resources (containers, items, etc.).
-
Copy and paste the cleanup
function underneath the deleteFamilyItem
function to remove the database and all its children resources.
async function cleanup() {
await client.database(databaseId).delete();
}
-
Copy and paste the code below the call to deleteFamilyItem
to execute the cleanup
function.
createDatabase()
.then(() => readDatabase())
.then(() => createContainer())
.then(() => readContainer())
.then(() => createFamilyItem(config.items.Andersen))
.then(() => createFamilyItem(config.items.Wakefield))
.then(() => queryContainer())
.then(() => replaceFamilyItem(config.items.Andersen))
.then(() => queryContainer())
.then(() => deleteFamilyItem(config.items.Andersen))
.then(() => cleanup())
.then(() => { exit(`Completed successfully`); })
.catch((error) => { exit(`Completed with error ${JSON.stringify(error)}`) });
Run your Node.js application
Altogether, your code should look like this:
const CosmosClient = require('@azure/cosmos').CosmosClient;
const config = require('./config');
const url = require('url');
const endpoint = config.endpoint;
const masterKey = config.primaryKey;
const HttpStatusCodes = { NOTFOUND: 404 };
const databaseId = config.database.id;
const containerId = config.container.id;
const client = new CosmosClient({ endpoint: endpoint, auth: { masterKey: masterKey } });
async function createDatabase() {
const { database } = await client.databases.createIfNotExists({ id: databaseId });
console.log(`Created database:\n${database.id}\n`);
}
async function readDatabase() {
const { body: databaseDefinition } = await client.database(databaseId).read();
console.log(`Reading database:\n${databaseDefinition.id}\n`);
}
async function createContainer() {
const { container } = await client.database(databaseId).containers.createIfNotExists({ id: containerId });
console.log(`Created container:\n${config.container.id}\n`);
}
async function readContainer() {
const { body: containerDefinition } = await client.database(databaseId).container(containerId).read();
console.log(`Reading container:\n${containerDefinition.id}\n`);
}
async function createFamilyItem(itemBody) {
try {
const { item } = await client.database(databaseId).container(containerId).item(itemBody.id).read();
console.log(`Item with family id ${itemBody.id} already exists\n`);
}
catch (error) {
if (error.code === HttpStatusCodes.NOTFOUND) {
const { item } = await client.database(databaseId).container(containerId).items.create(itemBody);
console.log(`Created family item with id:\n${itemBody.id}\n`);
} else {
throw error;
}
}
};
async function queryContainer() {
console.log(`Querying container:\n${config.container.id}`);
const querySpec = {
query: "SELECT VALUE r.children FROM root r WHERE r.lastName = @lastName",
parameters: [
{
name: "@lastName",
value: "Andersen"
}
]
};
const { result: results } = await client.database(databaseId).container(containerId).items.query(querySpec).toArray();
for (var queryResult of results) {
let resultString = JSON.stringify(queryResult);
console.log(`\tQuery returned ${resultString}\n`);
}
};
async function replaceFamilyItem(itemBody) {
console.log(`Replacing item:\n${itemBody.id}\n`);
itemBody.children[0].grade = 6;
const { item } = await client.database(databaseId).container(containerId).item(itemBody.id).replace(itemBody);
};
async function deleteFamilyItem(itemBody) {
await client.database(databaseId).container(containerId).item(itemBody.id).delete(itemBody);
console.log(`Deleted item:\n${itemBody.id}\n`);
};
async function cleanup() {
await client.database(databaseId).delete();
}
function exit(message) {
console.log(message);
console.log('Press any key to exit');
process.stdin.setRawMode(true);
process.stdin.resume();
process.stdin.on('data', process.exit.bind(process, 0));
}
createDatabase()
.then(() => readDatabase())
.then(() => createContainer())
.then(() => readContainer())
.then(() => createFamilyItem(config.items.Andersen))
.then(() => createFamilyItem(config.items.Wakefield))
.then(() => queryContainer())
.then(() => replaceFamilyItem(config.items.Andersen))
.then(() => queryContainer())
.then(() => deleteFamilyItem(config.items.Andersen))
.then(() => cleanup())
.then(() => { exit(`Completed successfully`); })
.catch((error) => { exit(`Completed with error ${JSON.stringify(error)}`) });
In your terminal, locate your app.js file and run the command:
node app.js
You should see the output of your get started app. The output should match the example text below.
Created database:
FamilyDatabase
Reading database:
FamilyDatabase
Created container:
FamilyContainer
Reading container:
FamilyContainer
Created family item with id:
Anderson.1
Created family item with id:
Wakefield.7
Querying container:
FamilyContainer
Query returned [{"firstName":"Henriette Thaulow","gender":"female","grade":5,"pets":[{"givenName":"Fluffy"}]}]
Replacing item:
Anderson.1
Querying container:
FamilyContainer
Query returned [{"firstName":"Henriette Thaulow","gender":"female","grade":6,"pets":[{"givenName":"Fluffy"}]}]
Deleted item:
Anderson.1
Completed successfully
Press any key to exit
Get the complete Node.js tutorial solution
If you didn't have time to complete the steps in this tutorial, or just want to download the code, you can get it from GitHub.
To run the getting started solution that contains all the code in this article, you will need:
Install the @azure/cosmos module via npm. Use the following command:
npm install @azure/cosmos --save
Next, in the config.js file, update the config.endpoint and config.primaryKey
values as described in Step 3: Set your app's configurations.
Then in your terminal, locate your app.js
file and run the command:
node app.js
Clean up resources
When these resources are no longer needed, you can delete the resource group, Azure Cosmos DB account, and all the related resources. To do so, select the resource group that you used for the Azure Cosmos DB account, select Delete, and then confirm the name of the resource group to delete.
Founded in 1975, Microsoft (Nasdaq “MSFT”) is the worldwide leader in software, services, devices and solutions that help people and businesses realize their full potential.