XP Logon Control






4.48/5 (11 votes)
An XPLogonControl that mimics the Windows XP Logon Interface
- Download source code and project (September 3, 2006) - 933.95
- Download source code and project - 933.02
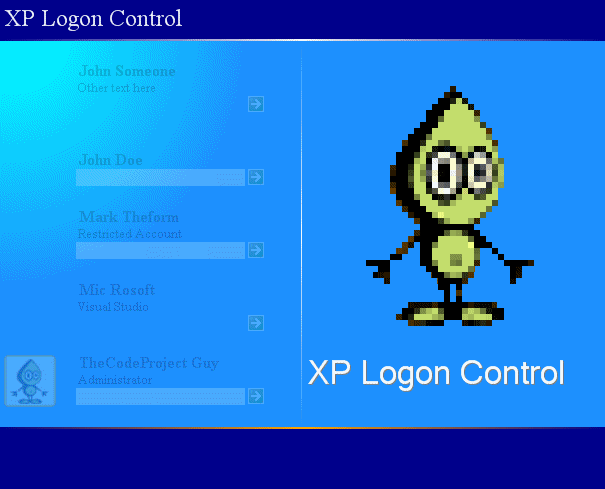
Introduction
This is a control that I first saw in The XP Common Controls (XPCC). The XPCC control however lacked some of the features that I would have liked, one being a transparency effect. This control has the same basic principle as the XPCC version and that is to mimic the Microsoft Windows XP login screen. The controls will mimic that login affect of the login screen when a user clicks on the login button. Some features that I have included that were not included in the XPCC control include: menu account transparency, bitmap background support, welcome screen capabilities, and a choice of how the login control works. This article will go over various drawing routines and control principles used in the XP Logon Control.
Painting
There are two areas of the control that are painted: XPLogonControl
and XPLogonAccount
. For the purpose of this article, we will start from the lowest to highest in what is painted. This will lead to the explanation of the XPLogonControl
first.
XPLogonControl
The XPLogonControl
is the basis for the entire control and gives the control the XP logon appearance. The XPLogonControl
is comprised of three rectangles: centRect
, headRect
, and footRect
, each representing the header, footer, and center of the XPLogonControl
respectively. To calculate the regions for the rectangles, I have created a method called ComputRects
which will calculate the location and size of each rectangle based on properties set for the control. This method is called whenever the paint routine is called so that the painting is correct. To prevent any lines or rectangles interfering with each other, the order in which the parts of the control are painted are specific and are as follows:
centRect
is painted using aLinearGradientBrush
with aPathGradientBrush
overlay. This gives that control the little circle in the upper left hand corner. Or if theShowBitmapBackground
is set totrue
aTextureBrush
is used to paint the background.Midline
is painted behind the header and footer so that the line will not mess with either header or footer painting. The midline is drawn with aLinearGradientBrush
withColorBlending
.Header
is drawn using aLinearGradientBrush
.Header
line is also drawn using aLinearGrad
andientBrush
ColorBlend
routine.- Since no other paint routines will be close to the header, the
headerText
will be painted on the header. Footer
is drawn using aLinearGradientBrush
.Footer
line is also drawn using aLinearGradientBrush
andColorBlend
routine.- All other adornments are added depending on whether the
ControlImage
orControlText
properties contain any data.
XPLogonAccount
The XPLogonAccount
will be the key menu system for this control, and whenever you add a LogonAccount
in the XPLogonControl
, an XPLogonAccount
will be shown with the LogonAccount
data, hence the reason why a LogonAccount
is a parameter for the XPLogonAccount
initialization. The painting for this control adds an interesting twist to how you normally would paint to a UserControl
. For this control, I created a bitmap object the size of this control and all painting that I do is painted to this bitmap. The painting includes various values from the LogonAccount
class that were sent through the parameter. The items painted to the bitmap are: Account_Name
, Account_Image
, Account_Subtext
, logon button, and a white area where the completely transparent RichTextBox
is located (text is painted to this white area whenever the RichTextBox
's text property changes, this is to make so that the text that the RichTextbox
contains is partially transparent as well). Once everything is painted to the image, I then paint the image partially transparent to the control. I paint the image to the control using a ColorMatrix
and an ImageAttribute
which will allow the partial transparency. An example of how I do this is in the following code snippet. currTrans
is the current transparency setting for the control, and b
is the bitmap that everything is being drawn to. In the ColorMatrix
you can see the fourth float array is the array in which you can set the transparency for the ColorMatrix
, in this case full transparency is 255 and currTrans
is a value between 50 and 225, so to get the proper transparency for the control we divide the values.
ImageAttributes ia=new ImageAttributes();
ColorMatrix cm=new ColorMatrix(new float[][]
{new float[]{1,0,0,0,0},
new float[]{0,1,0,0,0},
new float[]{0,0,1,0,0},
new float[]{0,0,0,(float)currTrans/255,0},
new float[]{0,0,0,0,1}});
ia.SetColorMatrix(cm);
b.MakeTransparent();
e.Graphics.DrawImage(b,new Rectangle(0,0,b.Width,b.Height) ,
0, 0, b.Width, b.Height,
GraphicsUnit.Pixel,ia); b.Dispose();
Account Management
Accounts for the control are handled in a roundabout way. You add a LogonAccount
class to accounts collection of the XPLogonControl
. Then when the LogonAccount
is added, the control will invalidate. When the control is invalidated, a set of events is undertaken and are as follows:
- Paint routines are called
- All controls on the
XPLogonControl
are cleared ScrollableAccountPanel
s are added depending on theLogonStyle
of the control.ScrollableAccountPanel
s areScrollableControl
s that will collect and organize all controls that are put into it. AllXPLogonAccount
s that are added to theScrollableAccountPanel
will be organized and if all of them will not fit into the space provided, scrollbars will appear so that you can maneuver through the accounts.XPLogonAccount
s are added to theScrollableAccountPanel
s.
These steps will repeat whenever there is a change in the accounts collection.
Other Notes
A few features have been added just for fun:
- A
SetTheme
property and method are located in theXPLogonControl
and whenever a value is changed with the method or property, then the colors of theXPLogonControl
will change to the specified theme. Themes included include:XPBlue
,XPGreen
,XPSilver
,Darkness
,Desert
, andTheCodeProject
:) .
Conclusion
Whether you understood half of what this article has gone over :), I hope that this control will be useful to all programmers.
Final Note: All code is distributed under GPL.
History
- September 3, 2006
- Password masking added
- April 27, 2006
- Initial version