Two Line Text Combobox
CComBoxEx is a simple MFC control derived from CWnd, it can display two line data
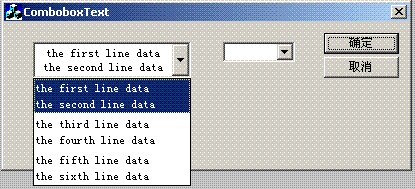
Introduction
CComBoxEx
is a simple MFC control derived from CWnd
. It can display two line text in combobox
control.
Background
A few days ago, in one of my projects, I need one combobox
control that could display two line text. Because the project time was tight, firstly I hoped that I could get some derived combobox
controls from The Code Project website, but I got nothing. Finally, I decided to make this control by myself. Now I completed this combobox
control, and it is not so bad for a VC++ beginner, so I decided to share this control with all CodeProject workmates. I did not have a lot of time to work on this control, so I had to code it in a simple manner, but it had to satisfy my requirements. It has some features as follows:
- It is simple, and easy to use.
- It is similar to the main functions of MFC
combobox
control. - It can display two line text.
Now the combobox
control is here. Enjoy and please help me with your invaluable notes, bugs reports, ideas, etc. that you think might improve the quality of this code.
Using the Code
Creating the Combobox Control
First, we need to create a two line text combobox
control.
- Add ComBoxEx.h and ComBoxEx.cpp to your project.
- Add
#include " ComBoxEx.h"
to the top of header file of class in which you want to add this two line text control. - Add a member variable of type
CComBoEx
. - In your cpp file, use the
Create
method of the member variable to create a two line textcombobox
control.// create combobox control // to get combobox control position CStatic *pStatic = (CStatic *)GetDlgItem(IDC_COMBOBOX_STATIC); CRect rcStatic; pStatic->GetWindowRect(rcStatic); ScreenToClient(rcStatic); // to create combobox control m_ComboxEx.Create(rcStatic, this, 2000); // add data to combobox control CString str1, str2; str1 = "the first line data"; str2 = "the second line data"; m_ComboxEx.AddString(str1, str2); str1 = "the third line data"; str2 = "the fourth line data"; m_ComboxEx.AddString(str1, str2); str1 = "the fifth line data"; str2 = "the sixth line data"; m_ComboxEx.AddString(str1, str2); // set combobox current selected item m_ComboxEx.SetCurSel(1); // Get Combobox Control Current Selected Item m_ComboxEx.GetCurSel();
Combobox
control notify messageWhen
combobox
control selects one item,combobox
control's parent window would receivecombobox
control's notify message, you can do it like this.// first declare msg response function in parent window header, under //}}AFX_MSG and up DECLARE_MESSAGE_MAP(), just like this. afx_msg LRESULT OnComboboxNotify(WPARAM wParam, LPARAM lParam); // second map combobox msg, between // BEGIN_MESSAGE_MAP and END_MESSAGE_MAP(), just like this. BEGIN_MESSAGE_MAP(CComboboxTextDlg, CDialog) //{{AFX_MSG_MAP(CComboboxTextDlg) ON_WM_SYSCOMMAND() ON_WM_PAINT() ON_WM_QUERYDRAGICON() ON_WM_CTLCOLOR() //}}AFX_MSG_MAP ON_MESSAGE(COMBOBOXEX_NOTIFYMSG, OnComboboxNotify) END_MESSAGE_MAP() // finally, code combobox msg process function LRESULT CComboboxTextDlg::OnComboboxNotify(WPARAM wParam, LPARAM lParam) { TRACE("Combobox CtrlID = %d, CurSelNo = %d\n", wParam, lParam); return 1; }
Next to Update
This Combobox
control is simple, and there are many functions that need update.
- Support font setting
Now this
combobox
control uses the default font, and I didn't implement font setting functions. Combobox
auto sizeCombobox
control can't auto size, when you use it, you must set control height and width to fit content, next I will implement it.Comboxlist
support scroll barCombobox
control calculatescomboxlist
size according tocombobox
control' size. Whencombobox
control items are very large,comboxlist
sizes are larger than screen size, it can't display all items on screen.Combobox
support item dataYou know MFC
combobox
control support information for every item, next to update thiscombobox
control and support this function.- Support dropdown style
Now this
combobox
control does not support this style, next add to it.
History
- 2011/11/22 Create
combobox
control