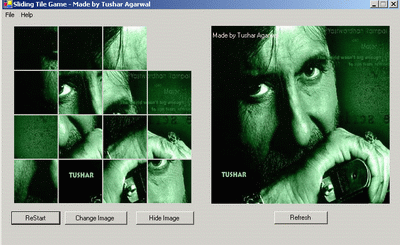
Introduction
In this article, you can learn how to edit images without using Windows API or other functions. You will also learn how to dynamically move and edit the controls.
While making this program, I needed some functions which could crop, resize, clip the image. But .NET framework doesn't provide us with any of such tools. So I wrote all the functions on my own because I did not wanted to use any of the APIs.
Objective and How to play the Game
This game has three modes:
- Number Mode (I haven't programmed it yet, but it's very easy to do).
- Picture Mode.
- Number and Picture Mode.
In this game, there are 15 tiles or buttons which you have to arrange numerically, or if you are playing in the picture mode then you have to arrange the titles in order to get the image as shown on the right side.
In this game, there is one empty tile where other tiles can come. Only the adjoining tiles can take its place. Whenever you click a tile (button), it shifts towards the empty block if there is a way. Like this way, you have to arrange the tiles so as to make the image as shown in the figure on the right side.
Using the code
First of all, I created a Panel
. I added 15 buttons to it. I stored all the buttons in an ArrayList
alAllButtons
. Each button's size is 80x80 pixel.
Now I load the default image and store it in a variable MainBitmap
.
Now I have to make 15 pieces of this image and that to be of size 80x80 pixels. Here is the code to do that:
ArrayList ilTemp = new ArrayList ();
int h,v;
h=v=0;
for(int k = 0; k <15; k++)
{
Bitmap b = new Bitmap(x,y);
for(int i =0; i< x; i++)
for(int j = 0; j< y; j++)
b.SetPixel (i,j,ToBeCropped.GetPixel ((i+h),(j+v)));
ilTemp.Add(b);
h+=80;
if (h == 320)
{h=0; v+=80;}
}
return ilTemp;
Now add Image
s to the Button
s. This is achieved with the help of the following code:
public void AddImagesToButtons()
{
ilSmallImages = ReturnCroppedList(MainBitmap,80,80);
button1.Image = (Image)ilSmallImages[0];
button2.Image = (Image)ilSmallImages[1];
button3.Image = (Image)ilSmallImages[2];
button4.Image = (Image)ilSmallImages[3];
button5.Image = (Image)ilSmallImages[4];
button6.Image = (Image)ilSmallImages[5];
button7.Image = (Image)ilSmallImages[6];
button8.Image = (Image)ilSmallImages[7];
button9.Image = (Image)ilSmallImages[8];
button10.Image = (Image)ilSmallImages[9];
button11.Image = (Image)ilSmallImages[10];
button12.Image = (Image)ilSmallImages[11];
button13.Image = (Image)ilSmallImages[12];
button14.Image = (Image)ilSmallImages[13];
button15.Image = (Image)ilSmallImages[14];
}
Now I have to jumble all the buttons so that they all mix up and the user can play the game.
private void Randomize ()
{
Random r = new Random ();
Button tempBtn = new Button();
for (int i =0; i < 100; i++)
{
tempBtn = (Button) alAllButtons[r.Next(alAllButtons.Count )];
MoveButton(tempBtn);
}
}
Now, whenever a button is clicked, it has to be moved to the empty point. A button is only moved when it is either horizontally or vertically inline with the empty point. This is checked with the help of the following code:
EmptyPoint.X == ClickedButton.Location.X || EmptyPoint.Y ==
ClickedButton.Location.Y
If this condition is satisfied then the button(s) is moved accordingly:
private void MoveButton(Button ClickedButton )
{
if(EmptyPoint.X == ClickedButton.Location.X
|| EmptyPoint.Y == ClickedButton.Location.Y)
{
Point tempEmptyPoint = new Point ();
tempEmptyPoint = ClickedButton.Location;
int d = Direction (ClickedButton.Location ,EmptyPoint);
if(EmptyPoint.X == ClickedButton.Location.X )
{
foreach(Button bx in panel1.Controls )
{
if (((bx.Location.Y >= ClickedButton.Location.Y)
&& (bx.Location.Y < EmptyPoint.Y )
&&(bx.Location.X == EmptyPoint.X ))
|| ((bx.Location.Y <= ClickedButton.Location.Y)
&& (bx.Location.Y > EmptyPoint.Y )
&&(bx.Location.X == EmptyPoint.X )))
{
switch (d)
{
case 1: Functions.MoveUp(bx);
break;
case 3: Functions.MoveDown(bx);
break;
}
}
}
}
if(EmptyPoint.Y == ClickedButton.Location.Y )
{
foreach(Button bx in panel1.Controls )
{
if (((bx.Location.X >= ClickedButton.Location.X)
&& (bx.Location.X < EmptyPoint.X )
&& (bx.Location.Y == EmptyPoint.Y )) ||
((bx.Location.X <= ClickedButton.Location.X)
&& (bx.Location.X >EmptyPoint.X )
&& (bx.Location.Y == EmptyPoint.Y )))
{
switch (d)
{
case 0 : Functions.MoveRight(bx);
break;
case 2: Functions.MoveLeft(bx);
break;
}
}
}
}
EmptyPoint = tempEmptyPoint;
}
}
A ChangeImage
function loads a random image from the database:
Bitmap b = new Bitmap (320,320);
Random r = new Random ();
string sql = "Select * from Bitmaps";
string strConn = @"Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" +
Application.StartupPath +"\\game.mdb";
OleDbConnection Conn = new OleDbConnection (strConn);
try
{
Conn.Open();
System.Data.OleDb.OleDbDataAdapter da =
new OleDbDataAdapter (sql,Conn);
DataSet ds = new DataSet ();
da.Fill (ds,"Bitmaps");
DataTable dt = ds.Tables["Bitmaps"];
DataRow dr;
do
{
dr= dt.Rows [r.Next (dt.Rows.Count )];
b=(Bitmap) Bitmap.FromFile (Application.StartupPath
+ "\\Images\\"+dr["Picture"].ToString ());
}while(dr["Type"].ToString () != c.ToString ());
}
catch (Exception ex)
{
MessageBox.Show (ex.Message.ToString ());
Conn.Close ();
}
finally
{
Conn.Close ();
}
return b;
}
The rest of the code is self explanatory. For any help or information, please feel free to contact me.
History
First version: 18 March 2004 (On my birthday).
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.