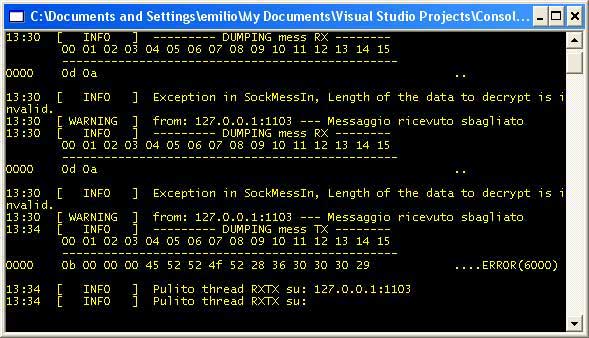
Introduction
In an environment of complex logging and catching exceptions, it's useful to dump byte arrays. To do this, any programmer will write his methods every time he needs them.
CodeProject is a placeholder for useful common code every programmer needs, so I add this small method that can dump easily internal data stored in the classes. I thought to publish this method which can easily dump byte arrays.
public class Settings
{
public string fmt_2digits ="{0:d2}";
public string fmt_4digits ="{0:d4}";
public string fmt_2dhex ="{0:x2}";
}
private Settings _settings = new Settings();
private void TraceDump(byte []ms, string what)
{
byte [] arr = ms;
int len = arr.Length;
if (len == 0)
return;
System.Text.StringBuilder sb = new System.Text.StringBuilder();
int rows = (int) (arr.Length / 16);
int cols = 16;
sb.Append("--------- DUMPING ");
sb.Append(what);
sb.Append(" --------\n\r");
sb.Append(" \t");
for(int j = 0; j < cols; j++)
sb.AppendFormat(_settings.fmt_2digits+" ",j);
sb.Append("\n\r \t");
for(int j = 0; j < cols; j++)
sb.Append("---");
sb.Append("\n\r");
string asciistr = "";
for (int i = 0; i < rows+1; i++)
{
int offset = cols*i;
sb.AppendFormat(_settings.fmt_4digits+"\t",offset);
for (int j = 0; j < cols; j++)
{
if ((offset+j) < len)
{
asciistr +=
(
(arr[offset+j] > 31 )
&&
(arr[offset+j] < 127 )
)
? Encoding.ASCII.GetString(arr,offset+j,1) : ".";
sb.AppendFormat(_settings.fmt_2hex+" ", arr[offset+j]);
}
else
sb.Append(" ");
}
sb.AppendFormat("\t{0}", asciistr);
sb.Append("\n\r");
asciistr = "";
}
_loggerwrapper.Log(sb);
}
Naturally, this method is in the lowest mode and the dump is possible in a context of serialization such as:
private void TraceDump(MemoryStream ms, string what)
{
ms.Position = 0;
TraceDump(ms.ToArray(),what);
}
Output
-------- DUMPING mess RX --------
00 01 02 03 04 05 06 07 08 09 10 11 12 13 14 15
------------------------------------------------
0000 0d 0a ..
Like you can see, the output is hex editor like and simplifies runtime data reading.
Namespaces
For this job, you must declare the use of some namespaces like these:
using System;
using System.Text;
Usage
The usage is very simple:
MemoryStream stream = new MemoryStream()
MyObject myobject = new MyObject()
...
... do something
...
BinaryFormatter formatter = new BinaryFormatter();
formatter.Serialize(stream, myobject);
Console.Write(TraceDump(_myArrayofBytes, "my object examined" );
I don't want to write a tutorial on formatting the classes, maybe in the future, but visualize what and how data it is stored in a stream is the scope of this tip.
You can dump in the Trace of Visual Studio 200x with the well-known method Trace.Write
:
System.Diagnostics.Trace.Write(TraceDump(_myArrayofBytes, "my object examined" );
else
Console.Write(TraceDump(_myStream, "my object examined" );
Point of interest
Is an object serializable? Oh! All objects can do it by implementing the ISerializable
interface; then people can serialize objects in the MemoryStream
, and dump it! There is no particular point of interest instead of the usability you can do!
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.