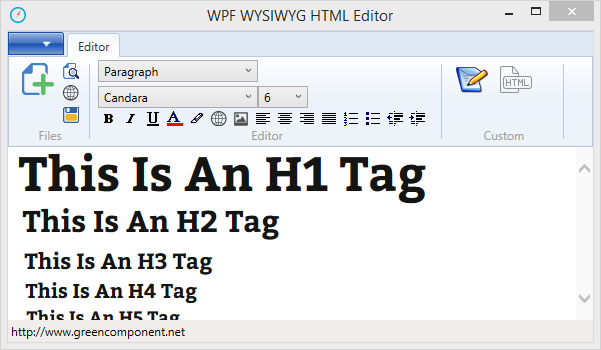
Introduction
In this tip, you will learn the use of WPF webbrowser
control and the use of the library mhtml for editing. This simple example will also help you understand how the toolbar works in WPF.
Background
In the development of a WPF application, I needed to edit HTML documents. After extensive research on already existing solutions in WPF without finding my happiness, I finally decided to write my own HTML editor.
After analyzing Winform solutions, I found that Microsoft made significant changes in the WPF Webbrowser
.
It is always possible to use WPF WindowsFormsHost
, but you lose the benefits of WPF.
In the WPF version of the webbrowser
, there is no more IsWebBrowserContextMenuEnabled
, ActiveXInstance
.
Ownership document has also been changed, the Winform version contains a document of type System.Windows.Forms.HtmlDocument
with lots of interesting methods such as PointToClient
and GetElementFromPoint
.
In WPF webrowser
, the document is a document
object and you need to cast it to mshtml.HtmlDocument
type.
The mshtml
library is a very powerful library that offers great possibilities for editing and analyzing an HTML document.
The official documentation is very well stocked.
https://msdn.microsoft.com/en-us/library/aa753630%28v=vs.85%29.aspx
WPF Interface
The benefits of WPF technology are numerous and has the potential to create many advanced interfaces.
A future article will be devoted to Ribbon Toolbar in WPF.
Editing the HTML
To edit a document using Microsoft.mshtml
library, it’s necessary to reference it in the project.
using mshtml;
public void newDocument()
{
webBrowser.NavigateToString(Properties.Resources.New);
doc = webBrowser.Document as HTMLDocument;
doc.designMode = "On";
}
Formatting the HTML
To change the heading of a selection, there are several ways to proceed.
This method uses FormatBlock webbrowser
control.
public static void RibbonComboboxFormat(ComboBox RibbonComboboxFormat)
{
string ID = ((Items)(RibbonComboboxFormat.SelectedItem)).Value;
webBrowser.doc = webBrowser.webBrowser.Document as HTMLDocument;
if (webBrowser.doc != null)
{
webBrowser.doc.execCommand("FormatBlock", false, ID);
}
}
Another solution is to use the library mshtml.
mshtml.IHTMLDocument2 doc;
doc = webBrowser1.Document.DomDocument as mshtml.IHTMLDocument2;
mshtml.IHTMLTxtRange r = doc.selection.createRange() as mshtml.IHTMLTxtRange;
mshtml.IHTMLElement parent = r.parentElement();
mshtml.IHTMLElement heading = doc.createElement("<h2>");
r.pasteHTML(heading.outerHTML);
With the Appendchild webbrowser
method, you can add elements such as tables or any other HTML too.
HtmlElement tableElem = webBrowser1.Document.CreateElement("TABLE");
webBrowser1.Document.Body.AppendChild(tableElem);
To format the text, you can proceed in several different ways, either by using the webbrowser
or the mshtml library.
In this example, I chose to use commands in the webbrowser
.
public static void InsertOrderedList(HTMLDocument doc)
{
if (doc != null)
{
doc.execCommand("InsertOrderedList", false, null);
}
}
Color Dialogbox in WPF
The dialog box Winform is incompatible with WPF.
WPF uses System.Windows.Media.Color and System.Windows.Media.Brush
while Winform uses System.Drawing.Color
.
The workaround is to made color transfer from the four channels individually.

public static System.Windows.Media.Color Pick()
{
System.Windows.Media.Color col = new System.Windows.Media.Color();
using (System.Windows.Forms.ColorDialog colorDialog = new System.Windows.Forms.ColorDialog())
{
colorDialog.AllowFullOpen = true;
colorDialog.FullOpen = true;
System.Windows.Forms.DialogResult result = colorDialog.ShowDialog();
if (result == System.Windows.Forms.DialogResult.OK)
{
col.A = colorDialog.Color.A;
col.B = colorDialog.Color.B;
col.G = colorDialog.Color.G;
col.R = colorDialog.Color.R;
}
}
return col;
}
Supress the Script Error Message
For pages containing scripts, the webbrowser
may generate errors, in the Winform version of the webbrowser
, there is a ScriptErrorsSuppressed
property that has unfortunately disappeared in the WPF webbrowser
.
webBrowser.ScriptErrorsSuppressed = true;
It is necessary to implement this functionality in WPF webbrowser
.
using System.Reflection;
public static void HideScriptErrors(WebBrowser wb, bool Hide)
{
FieldInfo FieldInfoComWebBrowser = typeof(WebBrowser).GetField
("_axIWebBrowser2", BindingFlags.Instance | BindingFlags.NonPublic);
if (FieldInfoComWebBrowser == null)
{
return;
}
object ComWebBrowser = FieldInfoComWebBrowser.GetValue(wb);
if (ComWebBrowser == null)
{
return;
}
ComWebBrowser.GetType().InvokeMember("Silent",
BindingFlags.SetProperty, null, ComWebBrowser, new object[] { Hide });
}
Conclusion
Thanks for viewing this tip. I expect feedback from you.
License
This tip, along with any associated source code and files, is licensed under The Code Project Open License (CPOL)