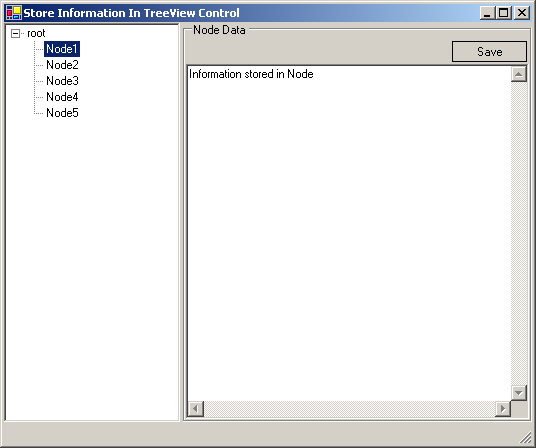
Introduction
This article will show you two things that you can do with the Tag
property. First, I will show you how to use the Tag
property of a control (here a TreeNode
) to store information. Then, I will show you how to link controls together with the Tag
property, which is used in this article to link DataRenderer
to the TreeNode
, which should save the data.
Background
The Tag
property is defined in the System.Windows.Forms.Control
class of the .NET Framework. This is the base class (directly or indirectly) of all the controls in Windows Forms.
The type of the Tag
property is object
. So you can store all kinds of information that you can think of. Object
is the "superclass" of .NET. All other classes are inherited (directly or indirectly) from this class. The primitive data types, like string
or int
, can always convert to or from an object
. This technique is called boxing/unboxing.
Using the Code
When the TreeView
control fires the AfterSelect
event, the TreeNode
information is displayed in a stupid TextBox txtData
. The TextBox
and the other controls (here only the "Save"-button) were created at runtime and docked on the GroupBox gpData
. The "Save" button was added to a Panel actBtnPanel
docked to the top. If a TreeNode
in the TreeView
control tvMain
is selected and this selected node has information in the Tag
property, then show this in txtData
.
For later saving, the TextBox
saves a reference to the TreeNode
object, where the information should be saved. The created Button
btnSave
stores a reference to the TextBox
object in the Tag
property. So it is able to find out the new information that has to be saved.
TextBox txtData = new TextBox();
if(this.tvMain.SelectedNode.Tag != null)
txtData.Text =
this.tvMain.SelectedNode.Tag.ToString();
txtData.Tag = this.tvMain.SelectedNode;
...
Button btnSave = new Button();
btnSave.Text = "Save";
btnSave.Tag = txtData;
btnSave.Click += new EventHandler(BtnSaveClick);
this.gpData.Controls.Add(txtData);
this.gpData.Controls.Add(actBtnPanel);
When the user changes the text in the TextBox
txtData
and clicks the "Save" button, the button instance itself is passed to the assigned event-handler as sender
. Here, the sender
was casted to the right type Button
. The Tag
property of the button has a reference to the Textbox
, which has the new content to be saved in TreeNode
. The Textbox
also has a reference to the TreeNode
(in its Tag
property), where the information is to be stored. And that's it. In the event-handler now we have all the information and references that are necessary to save the data.
private void BtnSaveClick(object sender, System.EventArgs e)
{
Button btnSave = sender as Button;
TextBox txtData = btnSave.Tag as TextBox;
TreeNode tnDesc = txtData.Tag as TreeNode;
if(txtData.Modified)
{
tnDesc.Tag = txtData.Text;
}
}
Points of interest
- In this article, you can see that you don't have to save all the interesting objects in the extra-defined reference variables to work with them. The controls themselves can save the information that they need to work with.
- .NET is great and I had a lot of fun programming in C#.
License
This article has no explicit license attached to it, but may contain usage terms in the article text or the download files themselves. If in doubt, please contact the author via the discussion board below.
A list of licenses authors might use can be found here.