Broadcasting Using Socket-Oriented Approach






3.05/5 (11 votes)
This solution explains a socket approach to send a message (broadcast message ) to clients in the same VLAN
Introduction
This solution explains a socket approach to sending a message (broadcast message) to clients in the same VLAN (Virtual Lan). If broadcasting is needed for the whole LAN (over all VLANs) in the same local area network then you have to use Remoting instead of Sockets.
How To Send Message to Connected LAN Clients Using Broadcasting
The application consists of two programs "Clfrm" and "SERVfrm." Clfrm exists on all LAN computers and SERVfrm exists on the server.
When the time is 12:00 AM the SERVfrm will send a broadCast message to all connected clients (Clfrm). The Clfrm will receive the message and run an alert form to notify the user.
Using the Code
I use the following namespace to do my job:
System.Net
System.Net.Sockets
The time was an important factor, as was having no delay when sending/receiving a message from the server to all clients. So, I used sockets instead Of remoting (remoting may cause a simple delay).
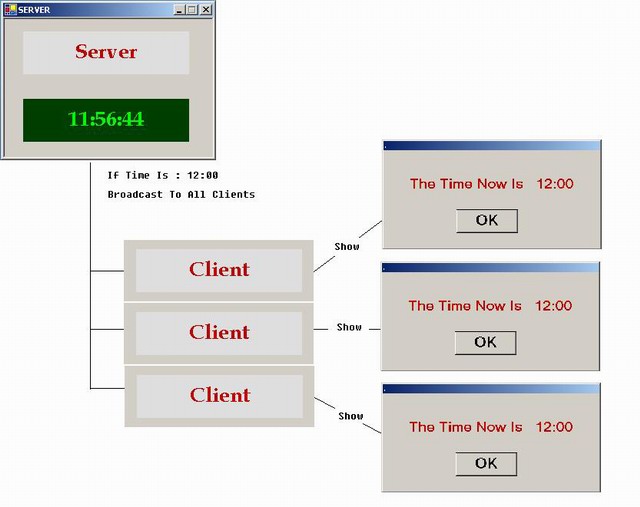
The Server Code
// Timer to Check Server Time
private void timer1_Tick(object sender, System.EventArgs e)
{
// Display Server Time
label1.Text = System.DateTime.Now.ToLongTimeString();
The timer here is to get the server time.
if(System.DateTime.Now.Hour == 11 && System.DateTime.Now.Minute==59 &&
System.DateTime.Now.Second==59)
Check if the time is 11:59:59.
{
/* Define a socket
Dgram Socket : Provides datagrams, which are connectionless messages
of a fixed maximum length.
This type of socket is generally used for short messages, such as a name
server or time server, since the order and reliability of message
delivery is not guaranteed
AddressFamily.InterNetwork indicates that an IP version 4 addresses
is expected when a socket connects to an end point. */
Socket sock = new Socket(AddressFamily.InterNetwork, SocketType.Dgram,
ProtocolType.Udp); // Protocol Type is UDP
//Get the IP Broadcast address , Use The Port 9050
IPEndPoint iep1 = new IPEndPoint(IPAddress.Broadcast, 9050);
//IP Broadcast Range using Port 9050
IPEndPoint iep2 = new IPEndPoint(IPAddress.Parse("192.168.100.255"),9050);
byte[] data = Encoding.ASCII.GetBytes("12"); // The Data To Sent /*
Set Socket Options ---> SocketOptionLevel
Member name Description
IP Socket options apply only to IP sockets.
IPv6 Socket options apply only to IPv6 sockets.
Socket Socket options apply to all sockets.
Tcp Socket options apply only to TCP sockets.
Udp Socket options apply only to UDP sockets.
*/
//SocketOptionName.Broadcast : to enable broadcast
sock.SetSocketOption(SocketOptionLevel.Socket,
SocketOptionName.Broadcast, 1);
sock.SendTo(data, iep1);
sock.SendTo(data, iep2);
sock.Close();
}
//
Note
In the first run of the client application the firewall will show the below message. When The Clfrm runs on the client machine it will not appear in the desktop or task manager Application tab.
I put ShowInaskbar = false
in 'Clfrm' which will hide the 'Clfrm' from the Task Manager Application Tab (it will appear in the process tab), and made Opacity = 2

Press unlock to allow the client to use 9050 port to receive messages from the server.
The Client Code
// public string TI ="0"; string stringData ;
This timer will check the value of stringData
private void timer1_Tick(object sender, System.EventArgs e) { Socket sock = new Socket(AddressFamily.InterNetwork,SocketType.Dgram, ProtocolType.Udp); /* The Socket.Bind method uses the Any field to indicate that a Socket instance must listen for client activity on all network interfaces. The Any field is equivalent to 0.0.0.0 in dotted-quad notation. */ IPEndPoint iep = new IPEndPoint(IPAddress.Any, 9050); //Associates a Socket with a local endpoint EndPoint ep = (EndPoint)iep; sock.Bind(iep); byte[] data = new byte[1024]; int recv = sock.ReceiveFrom(data, ref ep);// Receive Data stringData = Encoding.ASCII.GetString(data, 0, recv); TI=stringData; sock.Close(); } private void timer2_Tick(object sender, System.EventArgs e) { if(TI == "12") { timer2.Interval = 3000; TI = "0"; stringData = "0"; Process.Start(Application.StartupPath+"\\ALER.exe"); // Run ALER.exe } else { timer2.Interval =400; } } //
The sock.Close();
will close the port 9050 for future use.
Why Using Sockets instead of Remoting
- If you need bidirectional communication and if the data is a small fragment use sockets
- If you want the best performance
- When Client is waiting for notifications/events from server
- Remoting is a higher layer on Sockets. When both client and server is under your control you can use Remoting