How to : Using PHP Fusion 7 CAPTCHA






4.85/5 (3 votes)
Shows you how using PHP Fusion 7 CAPTCHA class
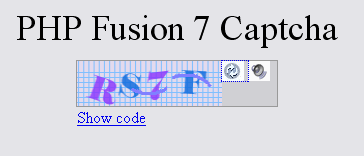
Introduction
PHP Fusion 7 has a greate CAPTCHA class under GPL license, so we can use it in our GPL projects.
This article shows you how we can use it.
Using the code
At First for using the PHP Fusion 7 CAPTCHA class we should create a data base and a table for storing the CAPTCHA codes.I've created them with a SQL like this :
/* Creating the DataBase */
CREATE DATABASE `phpfusion7`;
/* Creating the CAPTCHA table */
DROP TABLE IF EXISTS `phpfusion7`.`fusion_captcha`;
CREATE TABLE `phpfusion7`.`fusion_captcha` (
`captcha_datestamp` int(10) unsigned NOT NULL default '0',
`captcha_ip` varchar(20) NOT NULL,
`captcha_encode` varchar(32) NOT NULL default '',
`captcha_string` varchar(15) NOT NULL default '',
KEY `captcha_datestamp` (`captcha_datestamp`)
) ENGINE=MyISAM DEFAULT CHARSET=latin1;
If you are going to change the name of DB or table you should also change some information in the class's files. ( like config.php
and multisite_include.php
)Then we must create a PHP file that contains database setting. I've used the default file of PHP Fusion
In the CAPTCHA class we'll include it.
config.php
<?php
// database settings
$db_host = "localhost";
$db_user = "root";
$db_pass = "";
$db_name = "phpFusion7";
$db_prefix = "fusion_";
define("DB_PREFIX", "fusion_");
?>
Also I've already copied this file in my project ( multisite_include.php
). This file exist in PHP Fusion project and contains the name of the database's tables
securimage.php
in PHP Fusion 7 contains the CAPTCHA class.Now let's see adjustments variable of this CAPTCHA class.
var $image_width = 140; //Width of CAPTCHA image
var $image_height = 45;//Height of CAPTCHA image
//Type of CAPTCHA image
//SI_IMAGE_PNG for PNG image
//SI_IMAGE_GIF for GIF image
//SI_IMAGE_JPEG for JPG image
var $image_type = SI_IMAGE_PNG;
var $code_length = 4; // length of CAPTCHA code that'll show in image
//All characters that'll use for creating CAPTCHA code
var $charset = 'ABCDEFGHKLMNPRSTUVWYZ23456789';
//If you want, this class uses the characters that store in a file, set this variable
//and set $use_wordlist to true
var $wordlist_file = '';
var $use_wordlist = true;
//For using GD fonts that store in gdfonts folder
var $use_gd_font = false;
//Name of destination GD font
var $gd_font_file = 'gdfonts/bubblebath.gdf';
var $gd_font_size = 20;//Size of GD font
var $ttf_file = "./elephant.ttf";//Path of TTF Font file
var $font_size = 24;//Size of TTF font
//Maximum and minimum angle of CAPTCHA code
//The CAPTCHA code will rotate with these angles
var $text_angle_minimum = -20;
var $text_angle_maximum = 20;
//Start of X posiotion for writing CAPTCHA code
var $text_x_start = 8;
//Maximum and minimum of distance between CAPTCHA words
var $text_minimum_distance = 30;
var $text_maximum_distance = 33;
var $image_bg_color = "#e3daed";//Background of CAPTCHA image
var $text_color = "#ff0000";//Text color of CAPTCHA image
//If you set this one to true the CAPTCHA code will show colorful else will show in red
var $use_multi_text = true;
//Colors for coloring the words in CAPTCHA image
var $multi_text_color = "#0a68dd,#f65c47,#8d32fd";
//For showing the CAPTCHA code with transparency
var $use_transparent_text = true;
var $text_transparency_percentage = 50;//Percent of CAPTCHA code transparency
var $draw_lines = true;//For drawing lines in CAPTCHA image
var $line_color = "#80BFFF"; //Color of lines
var $line_distance = 5; //The distance between lines
var $line_thickness = 1; //Thickness of lines
var $draw_angled_lines = false;//Draw lines with a angle
var $draw_lines_over_text = false;
var $arc_linethrough = true; //Draw two arcs over the words in the CAPTCHA image
var $arc_line_colors = "#8080ff";//Color of arcs
var $audio_path = './audio/';//Path of audio files for
The results of some adjustments : var $text_angle_minimum = -50;
var $text_angle_maximum = 50;
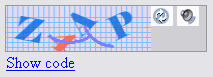
var $text_minimum_distance = 10;
var $text_maximum_distance = 15;
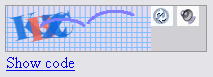
var $use_multi_text = false;
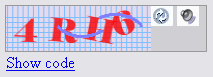
var $use_transparent_text = true;
var $text_transparency_percentage = 80;
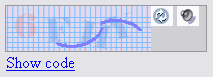
var $draw_lines = false;
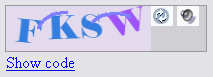
var $draw_lines = true;
var $line_color = "#80BFFF";
var $line_distance = 5;
var $line_thickness = 1;
var $draw_angled_lines = true;
var $draw_lines_over_text = false;
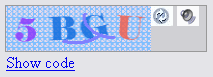
var $draw_lines = true;
var $line_color = "#80BFFF";
var $line_distance = 5;
var $line_thickness = 1;
var $draw_angled_lines = false;
var $draw_lines_over_text = true;
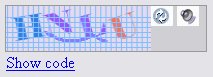
var $arc_linethrough = false;
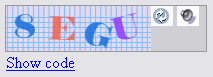
Allright, now we can use the class.
securimage_show.php
generates the CAPTCHA image. Just we should use it correctly.Like this :
<script language="javascript" type="text/javascript">
function RefreshCaptcha()
{
var captchaImage = document.getElementById('captcha');
captchaImage.src = 'securimage/securimage_show.php?sid=' + Math.random();
return false
}
</script>
<img src="securimage/securimage_show.php?sid=' +
Math.random();" alt="Validation Code:"
name="captcha" width="145" height="45" align="left" id="captcha"/>
<a onclick="RefreshCaptcha();" href="#">
<img align="top" alt="" src="securimage/images/refresh.gif"/> </a>
<a href="securimage/securimage_play.php">
<img align="top" style="margin-bottom: 1px;" alt=""
src="securimage/images/audio_icon.gif"/>
</a>
After that we can access to the CAPTCHA code with a mysql query like this PHP code :getCode.php :
<?php
function getCode()
{
require_once("config.php");
require_once("securimage/multisite_include.php");
mysql_connect($db_host, $db_user, $db_pass);
mysql_select_db($db_name);
$result = mysql_query("SELECT MAX(captcha_datestamp),
captcha_string FROM ".DB_CAPTCHA." WHERE captcha_ip='" .
$_SERVER['REMOTE_ADDR'] . "'")
or die(mysql_error());
if (mysql_num_rows($result))
{
$data = mysql_fetch_assoc($result);
return strtoupper($data['captcha_string']);
}
else
{
return "";
}
}
$captchaCode = getCode();
echo $captchaCode;
?>
Other my CAPTCHA articles :
Captcha Image in PHP
How to : Using Phpbb3 captcha
History
First post : 26th of December, 2008