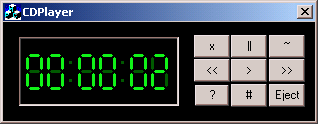
Introduction
This project explores the Microsoft Media Control Interface (MCI). I wrote a wrapper for MCI CD Audio commands. The class wrapper was named CCDAudio
.
Details
The CCDAudio
class declaration is:
class CCDAudio
{
public:
CCDAudio();
virtual ~CCDAudio();
MCIERROR Play();
MCIERROR Play(const int nPos);
MCIERROR Stop();
MCIERROR Pause();
MCIERROR Forward();
MCIERROR Backward();
void EjectCDROM();
int GetCurrentPos();
int GetCurrentTrack();
int GetLenghtAllTracks();
int GetTracksCount();
int GetTrackLength(const int nTrack);
int GetTrackBeginTime( const int nTrack );
bool IsMediaInsert();
bool IsPaused();
bool IsStopped();
bool IsReady();
bool IsPlaying();
protected:
MCIERROR m_nErrorCode;
protected:
inline void MCIError( MCIERROR MCIError );
};
All of the functions are divided into three groups:
- Manage functions.
- Time and position functions.
- Status functions.
Note: The time is represented in seconds.
Use of CCDAudio
- First you should create an instance of this class:
protected:
CCDAudio m_CDAudio;
- For starting to play the tracks:
m_CDAudio.Play();
- To pause playing:
m_CDAudio.Pause();
- To stop playing:
m_CDAudio.Stop();
- Move to the previous track:
m_CDAudio.Backward();
- Move to the next track:
m_CDAudio.Forward();
- Eject/Close CD ROM:
m_CDAudio.EjectCDROM();
- Play from begining of the selected track:
m_CDAudio.Play( m_CDAudio.GetTrackBeginTime( nTrack ) );
That is all. I hope this class will be useful.