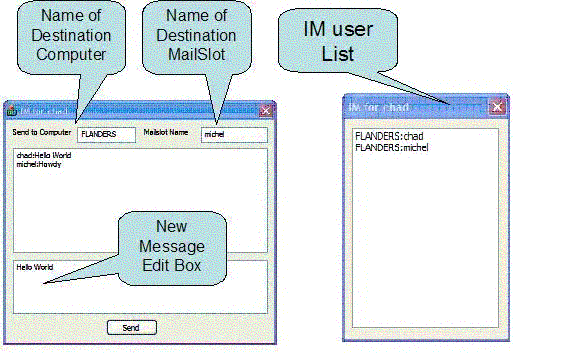
Introduction
Instant messaging is more than just a way for some 18 year old girls to keep
track what they are all going to wear the next day at school. IM is being used
by businesses for internal communication. IM can be a very affective tool for
getting information quickly back from a coworker.
IM can enhance many existing business applications. The real problem is how
to add this support while not writing too much actual code. One solution would
be to use some sort of TCP/IP server, and have a bunch of TCP/IP clients connect
to the server. That type of solution might prove to be somewhat difficult.
Luckily there is a real easy way to send messages from one computer to another,
“mailslots”.
Mailslots are a message passing algorithm for one directional communications.
Using a small amount of code you can get a good start on an IM client, without
ever writing a server. My solutions presented here is a rough prototype for
building a mailslot based chat program. A good amount of work will still need to
go into it to really make an affective tool. But, if you are willing to spend
some time you could easily integrate a mailslot chat program into an already
existing MFC application.
Running the sample
After building the solution you will need to have all users of the software
run it from the same directory. So you will need to create a shared directory
that all users of the mailslot program can access. To start MailSlotApp.exe you
need to pass your username as the only command line parameter. For example,
"MailSlotApp Chad" you start the program with the user "Chad". When the program
starts the user "Chad" would be added to the file "userlist.dat".
When the UserList dialog starts up it will list the contents of the
"userlist.dat" file. As users login there names will be added to the
"userlist.dat" file and a notification will be sent to all active mailslots.
(currently no notification is included for logout).
To send a message to another user just double click on their name in the list
and a MailSlotDlg will appear. From the MailSlotDlg you can enter a new message
and send it of to the other user. When the other user receives the message the
m_mailSlot
object in their MailSlotApp.exe will read the message
and notify the UserList
that a new message has arrived.
After receiving notification of the new MailSlot message their UserList
dialog will create a new non-modal dialog to manage the conversation with that
particular user.
Source Code Organization
- Package.h/cpp
- Serializable CObject. Base for MailSlotMessage.
- MailSlot.h/cpp
- MailSlot and MailSlotMessage classes.
- MailSlotApp.h/cpp
- Test Application.
- MailSlotDlg.h/cpp
- IM dialog.
- UserList.h/cpp
- User selection dialog. Spawns MailSlotDlg's as needed.
The most useful classes to reuse in your own projects will probably be found
in Package.h/cpp and MailSlot.h/cpp. The actual dialogs in UserList and
MailSlotDlg need some work before they could really be useful.
The project contains two dialogs. The first dialog is the UserList dialog.
This is kind of like your buddy list in a normal IM client. Here you should be
presented with a list of all users that are running the program from the same
directory as you.
The second dialog is the MailSlotDlg. This dialog will display your
conversation history with a particular user, and it will allow you to enter new
messages. The MailSlotDlg class never does directly interact with the
MailSlot.
All communication between users occurs through the use of a
MailSlot
object m_mailSlot
within the UserList dialog.
When you send a message from the MailSlotDlg that message will actually get
forwarded to the UserList dialog which will then finish sending the message.
When a new message has been received it is the responsibility of the UserList
dialog to process the message and determine where to forward the message.
Send Message: MailSlotDlg-->UserList-->MailSlot
Receive Message: MailSlot->UserList-->MailSlotDlg
MailSlot
Class
The MailSlot
class can be used to abstract away the details of
actually sending and receiving data from an actual mailslot. To use the
MailSlot
class all you need to do is create an instance of
MailSlot
and call its create method.
m_mailSlot.Create(m_userName, this, MAILSLOT_NOTIFY_MSG);
Sending an message using the mailslot class is real easy. Just call
MailSlot::Send(..)
m_mailSlot.Send(*pMsg);
Reading from a mailslot involves setting up a method to handle the messages
sent to MAILSLOT_NOTIFY_MSG
BEGIN_MESSAGE_MAP(UserList, CDialog)
ON_MESSAGE(MAILSLOT_NOTIFY_MSG, OnMailSlotNotify)
END_MESSAGE_MAP()
LRESULT UserList::OnMailSlotNotify(WPARAM w, LPARAM l)
{
MailSlotMessage msg;
while (m_mailSlot.Read(msg)) {
if (msg.MsgType() == MailSlotMessage::TEXT) {
MailSlotDlg * pWnd = GetDlg(msg.FromComputerName(),
msg.FromUserName());
if (pWnd)
pWnd->AddMessageToHistory(msg);
} else {
if (msg.MsgText() == LOGIN_MSG) {
RefreshList();
}
}
}
return 1;
}
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.