Introduction
Ever tried to integrate a reliable and fast web browser in your app only to jump through hoops trying?
In this tip, you will learn how easy it is to integrate the fantastic CefSharp web browser component (based on Chromium) into your C# app.
You can then use this web browser:
- To give users an integrated browsing experience
- To add embedded UI programmed in HTML/JavaScript
- For web automation
CefSharp is reliable, fast, fully open-source and does not need any extra dependencies to be installed by end users (except VC++ Runtime 2013).
In this guide, we will use a WinForms project but CefSharp works equally well with WPF projects.
Getting Started
Follow these steps to quickly get started with CefSharp.
Project
1. Create a Windows Forms Application (C#) project with .NET 4.5.2
2. Name your project (e.g. "CefTest
")
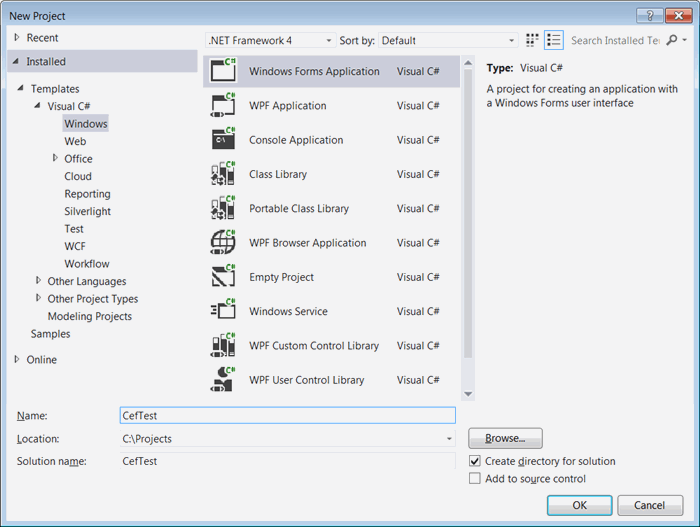
DLLs (NuGet Method)
3A) If you use NuGet, you can just grab CefSharp.WinForms from the NuGet repository, and skip the manual method below.
3B) If you used NuGet, navigate to SOLUTION > Configuration Manager and set your project to x86 or x64 since AnyCPU is not currently supported.

DLLs (Manual Method)
PLEASE SKIP THIS SECTION IF YOU USED NUGET!
3A) Download the CefSharp binaries and unzip them.
3B) Right click your project & click "Add Reference", then "Browse".

3C) Select these DLLs:
- CefSharp.dll
- CefSharp.Core.dll
- CefSharp.WinForms.dll

3D) Select all the files in the ZIP:

3E) Copy those files into your Project's Debug folder (inside Bin):

Code
4. Right click your main form and select "View Code".

5. Add the following code:
Copy this into your import
section:
using CefSharp;
using CefSharp.WinForms;
Copy this into your code section:
public ChromiumWebBrowser browser;
public void InitBrowser(){
Cef.Initialize(new CefSettings());
browser = new ChromiumWebBrowser ("www.google.com");
this.Controls.Add(browser);
browser.Dock = DockStyle.Fill;
}
Call InitBrowser()
just after the call to InitializeComponent()
:
InitBrowser()
Your code should finally look like this:

Run!
6. Press F5 and you will see the Google homepage!

Troubleshooting
An unhandled exception of type 'System.IO.FileNotFoundException' occurred in browser.exe
Additional information: Could not load file or assembly 'CefSharp.Core.dll' or one of its dependencies.
If you get this error, ensure you have Visual C++ 2013 Redistributable installed.
An unhandled exception of type 'System.BadImageFormatException' occurred in browser.exe
Additional information: Could not load file or assembly 'CefSharp.Core, Version=43.0.0.0, Culture=neutral,
If you get this error, it means you are on a 64-bit PC and your app is built in AnyCPU mode. You need to change this to x86 or x64 mode:
- Open the Solution Explorer panel
- Right click your project > Project Properties
- Click on the Build tab
- Change Platform target to x86 or x64
Full Featured Browsers
If you are looking for a full-featured browser project, then check out these open source browsers built with CefSharp in C#.
WPF. Address Bar with Suggestions, Multiple tabs, Downloads, Bookmarks, History.
<screenshot not provided by author>
Windows Forms. Address Bar, Multiple tabs, Downloads.

History
- V1.0 - Published the article on Nov 23rd, 2015
- V1.1 - Added troubleshooting guide on Nov 25th, 2015
- V1.2 - Added NuGet guide and VS project ZIP on Nov 26th, 2015
- V1.3 - Added troubleshooting tip for 64-bit PCs on Aug 6th 2016
- V1.4 - Updated to CefSharp v51, and .NET 4.5.2 on Aug 28th 2016
- V1.6 - Added SharpBrowser and WebExpress projects on Sep 2th 2016
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.