Introduction
In this article I will explain how to start using Twitter api with C# only without any third-party libraries.
This article is intented developers who have basic skills in http/https but have not yet learned REST architecture and OAuth system, or learned already with other services but have problems with Twitter.
Background
For a start, you need:
- Twitter account (requires email confirmation, and can be full locked without incoming sms verification, but not outgoing)
- Any Visual Studio
Authorize your account in twitter and open https://apps.twitter.com/, click create new app button
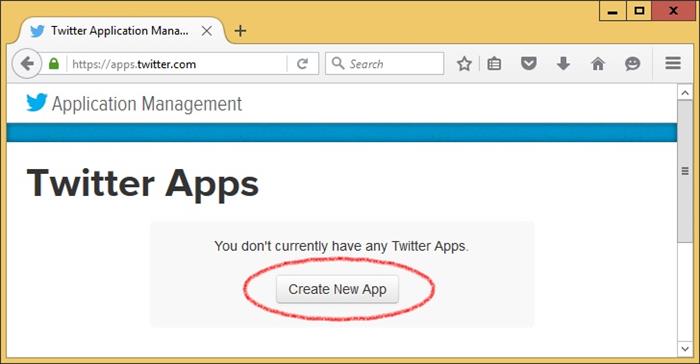
Provide application name should be unique, description and website URL can be fake:

Scroll bottom, accept checkbox and click create your twitter application:

You app created, go keys and access tokens tab:

Copy the api key and api secret to clipboard:

Paste them to notepad in this form:

This string should be base64-encoded I using https://www.base64encode.org/ with UTF-8
Encoded string:

Encoded string is credentials to get bearer access token for application. Access token should be get programmatically on every application run and should not store anywhere
Using The Code
To send any http requests:
using System.Net;
To work with post requests:
using System.IO;
Do not forgot twitter anywhere supports https requests only not http.
To get bearer access token send http post request and pass credentials to Authorization header
string access_token = "";
var post = WebRequest.Create("https://api.twitter.com/oauth2/token") as HttpWebRequest;
post.Method = "POST";
post.ContentType = "application/x-www-form-urlencoded";
post.Headers[HttpRequestHeader.Authorization] = "Basic " + credentials;
var reqbody = Encoding.UTF8.GetBytes("grant_type=client_credentials");
post.ContentLength = reqbody.Length;
using (var req = post.GetRequestStream())
{
req.Write(reqbody, 0, reqbody.Length);
}
try
{
string respbody = null;
using (var resp = post.GetResponse().GetResponseStream())
{
var respR = new StreamReader(resp);
respbody = respR.ReadToEnd();
}
access_token = respbody.Substring(respbody.IndexOf("access_token\":\"") + "access_token\":\"".Length, respbody.IndexOf("\"}") - (respbody.IndexOf("access_token\":\"") + "access_token\":\"".Length));
}
catch
{
}
To exploit any REST api methods send http get or post requests and pass bearer token to Authorization header,
For example you have method https://dev.twitter.com/rest/reference/get/statuses/user_timeline
Your request is
var gettimeline = WebRequest.Create("https://api.twitter.com/1.1/statuses/user_timeline.json?count=3&screen_name=twitterapi") as HttpWebRequest;
gettimeline.Method = "GET";
gettimeline.Headers[HttpRequestHeader.Authorization] = "Bearer " + access_token;
try
{
string respbody = null;
using (var resp = gettimeline.GetResponse().GetResponseStream())
{
var respR = new StreamReader(resp);
respbody = respR.ReadToEnd();
}
MessageBox.Show(respbody);
}
catch
{
}
Results


For more security when app closes send request to invalidate token (to avoid it stolen and used by malware)
var inv = WebRequest.Create("https://api.twitter.com/oauth2/invalidate_token") as HttpWebRequest;
inv.Method = "POST";
inv.ContentType = "application/x-www-form-urlencoded";
inv.Headers[HttpRequestHeader.Authorization] = "Basic " + credentials;
var reqbodyinv = Encoding.UTF8.GetBytes("access_token=" + access_token);
inv.ContentLength = reqbodyinv.Length;
using (var req = inv.GetRequestStream())
{
req.Write(reqbodyinv, 0, reqbodyinv.Length);
}
try
{
post.GetResponse();
}
catch
{
}
History
02/03/2016: Published first time
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.