Matlab 7.1+ and Visual Studio 2005
An article describing how to compile Matlab 7.1-7.4 mexw32 files using Visual Studio 2005
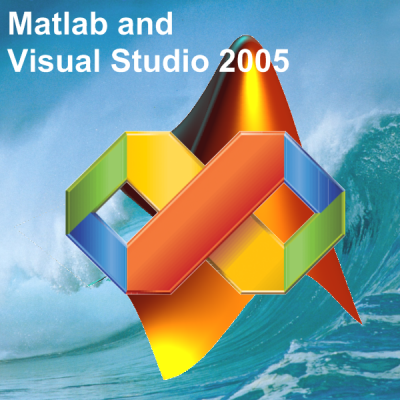
Introduction
This program shows how to compile and link the new Matlab mexw32 files using Visual Studio 2005. Matlab 7.1 changed its mex file format to .mexw32 from .dll. All new versions, MATLAB 7.1 (R14SP3), MATLAB 7.2 (R2006a), MATLAB 7.3 (R2006b), and MATLAB 7.4 (R2007a), compile mexw32 files. Previously compiled mex .dlls must be recompiled. Using Visual Studio 2005 makes this process easy. Furthermore, there are many times where one needs to use outside headers and libraries from other C/C++ functions. Visual Studio works much better than Matlab's own compiler in these situations. Mex files allow one to create very powerful Matlab functions, and are able to use all the functionality of .m Matlab script files.
Background
Why did I learn this in the first place? I needed to control a laser gyroscope from Matlab. I had the C functions to control the laser from the command prompt, so I needed to implement the C code inside of a Matlab mexw32 file. Not only did I need to add the libraries and headers for the mex file to compile, but I also needed to add in the laser libraries. My example code contains function calls to the laser device, however they are commented out since they will not compile without the correct libraries. You can switch your code, for your own specific function calls, for mine. - A. Bryant Nichols Jr.
Using the Code
You can either open my solution file, and modify the properties to link to your matlab directory, or follow these steps:
- Create a new C++ Win32 console application.
- Add mexversion.rc from the MATLAB include directory, matlab\extern\include, to the project.
- Create a .def file with the following text, and add it to the Solution.
Note: ChangemyFileName
to yours.LIBRARY myFileName EXPORTS mexFunction
- Under C/C++ General Additional Include Directories, add matlab\extern\include.
- Under C/C++ Preprocessor properties, add
MATLAB_MEX_FILE
as a preprocessor definition. - Under Linker General Output File, change to .mexw32
- Under Linker General Additional Library Directories, add matlab\extern\lib\win32\microsoft
- Under Linker Input Additional Dependencies, add libmx.lib, libmex.lib, and
libmat.lib
as additional dependencies. - Under Linker Input Module Definition File, add the (.def) file you created.
- Under General Configuration Type, choose .dll.
- Add more links to includes and libraries that you will need for your program. In my case, I also added the \include and \lib dependencies for the laser gyroscope.
The Code
The headers required by Matlab. Note: Include your own headers for more specific functions.
#include "stdafx.h"
#include "Windows.h"
#include "mex.h"
#include "matrix.h"
//#include "AerSys.h"
This is my own function, used to control the laser.
void StartDevice();
This is the main entry point for the mex file. nlhs
is the number of outputs, *plhs
is the output pointer, rlhs
is the number of inputs, and *prhs
is the input pointer.
void mexFunction(int nlhs, mxArray *plhs[], int nrhs, const mxArray *prhs[])
The first variable read from the input is iCase
which is used for the case
statement. See section Sample for all of the possible input/output options.
if (nrhs>0)
{
data1 = mxGetPr(prhs[0]);
iCase = (int)data1[0];
}
if (nrhs>1)
{
data1 = mxGetPr(prhs[1]);
dist1 = data1[0];
if (iCase==4)
{
char buf[128];
mwSize buflen;
// Allocate enough memory to hold the converted string.
buflen = mxGetNumberOfElements(prhs[1]) + 1;
mxGetString(prhs[1], buf, buflen);
sprintf_s(szProgram,buf);
}
}
if (nrhs>2)
{
data1 = mxGetPr(prhs[2]);
dist2 = data1[0];
}
StartDevice();
if (iCase==3)
{
if (nlhs>=2)
{
plhs[0] = mxCreateDoubleMatrix(1, 1, mxREAL);
plhs[1] = mxCreateDoubleMatrix(1, 1, mxREAL);
data1 = mxGetPr(plhs[0]);
data1[0] = dXPos/AZCOUNTS;
data1 = mxGetPr(plhs[1]);
data1[0] = dYPos/ELCOUNTS;
}
}
return;
This is the call to my function, however it will not compile without the correct laser device libraries.
void StartDevice()
Sample Matlab Commands
These are optional commands that could be used in Matlab to run the laser. Note: Even though the filename is MatlabVS.m, if one types MatlabVS(1);
at the Matlab command prompt, the MatlabVS.mexw32 file gets priority and will be run. This is a nice way to comment your mexw32 files so users can understand its usage format.
% function MatlabVS(option, var1, var2)
%
% 0) To Reset...
% MatlabVS(0);
%
% 1) To Initialize...
% MatlabVS(1);
%
% 2) To Absolute Move...
% MatlabVS(2,Xdist,Ydist);
%
% 3) To Return Positions
% [AZ,EL]=MatlabVS(3);
%
% 4) To Run GCode...
% MatlabVS(4,FileName);
% e.g. MatlabVS(4,'Aero.pgm');
%
% 5) To Increment Move...
% MatlabVS(5,Xdist,Ydist);
%
% 6) To Home...
% MatlabVS(6);
Points of Interest
Matlab is a great tool for array and matrix calculations, plotting, analysis, etc. The added use of C/C++ in Matlab mexw32 files allows one to acquire realtime data from external C controlled devices and analyze it right away inside of Matlab. With Matlab 7.1+ and Visual Studio 2005, the sky's the limit!
History
- Version 1.1