Extending the GridView CommandField To Add Delete Confirmation






4.42/5 (11 votes)
Tired of constantly writing the same labourious plumbing to add a confirmation to a delete button in a GridView? I was, so I simplified things.
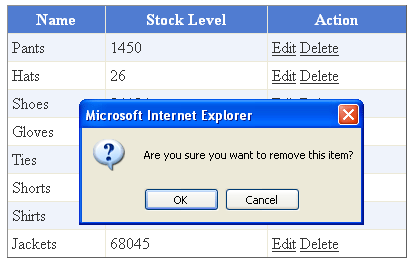
Introduction
We've all added delete confirmations before; they're a really great way of stopping users from doing something they didn't intend with disastrous consequences. Sadly the GridView
's CommandField
doesn't natively support this, requiring lots of boring plumbing to be duplicated in code-behind files. This article describes a simple, clean and reusable method of adding a delete confirmation by extending the CommandField
control.
Background
This article roughly follows on from my previous article on extending the GridView control and illustrates in a similar manner, the advantage of extending existing controls to provide additional functionality.
About the Code
The sample project contains a demo form and the key class ExtendedCommandField
. It simply inherits from the standard CommandField
class and provides a new property, DeleteConfirmationText
, that allows you to set the text that will appear in the confirmation box. If no text is supplied, the field behaves like a standard CommandField
and provides no delete confirmation. When the property is set, the usual basic JavaScript to display the confirmation box is added to the delete button.
This is all done in the InitializeCell
method where you can perform all sorts of tricks to further customize the appearance and behaviour of the field.
/// <summary>
/// An extended <see cref="CommandField"/> that allows deletions
/// to be confirmed by the user.
/// </summary>
public class ExtendedCommandField : CommandField
{
/// <summary>
/// Initialize the cell.
/// </summary>
public override void InitializeCell(DataControlFieldCell cell,
DataControlCellType cellType, DataControlRowState rowState, int rowIndex)
{
base.InitializeCell(cell, cellType, rowState, rowIndex);
if (!string.IsNullOrEmpty(this.DeleteConfirmationText) && this.ShowDeleteButton)
{
foreach (Control control in cell.Controls)
{
IButtonControl button = control as IButtonControl;
if (button != null && button.CommandName == "Delete")
// Add delete confirmation
((WebControl)control).Attributes.Add("onclick", string.Format
("if (!confirm('{0}')) return false;", this.DeleteConfirmationText));
}
}
}
#region DeleteConfirmationText
/// <summary>
/// Delete confirmation text.
/// </summary>
[Category("Behavior")]
[Description("The text shown to the user to confirm the deletion.")]
public string DeleteConfirmationText
{
get { return this.ViewState["DeleteConfirmationText"] as string; }
set { this.ViewState["DeleteConfirmationText"] = value; }
}
#endregion
}
Using the Code
You can then use the ExtendedCommandField
in your GridView
just as you would a normal column.
<asp:GridView ID="grid" runat="server">
<Columns>
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="StockLevel" HeaderText="Stock Level" />
<Alex:ExtendedCommandField DeleteConfirmationText=
"Are you sure you want to remove this item?"
HeaderText="Action" ShowDeleteButton="True" />
</Columns>
</asp:GridView>
Don't forget, of course, that you'll need a @Register
directive at the top of the page so ASP.NET knows where to find the control.
Points of Interest
The InitializeCell
method is a convenient way to customize the behaviour and appearance of GridView
fields. More generally, one could easily extend the DataControlField
to provide completely custom behaviour. One such extension I intend to develop soon is a DropDownListField
which allows users to select values from a DropDownList
within a GridView
cell.
History
- 06-Sep-07: First version