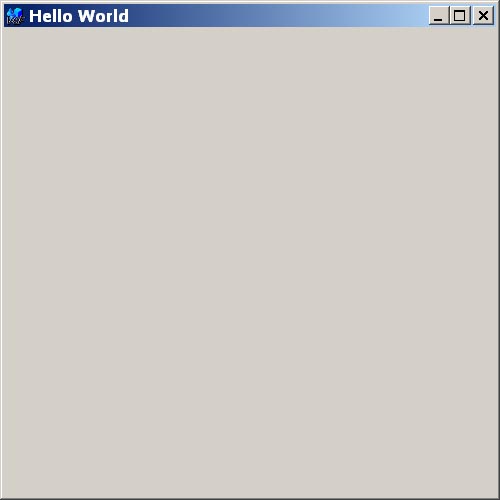
About the Visual Component Framework
The Visual Component Framework was inspired by the ease of use of environments like NeXTStep's Interface Builder, Java IDE's like JBuilder, Visual J++, and Borland's Delphi and C++ Builder. I wanted a generic C++ class framework I could use to build apps quickly and visually (when designing GUIs), as well as having the core of the framework be as cross platform as possible. The Visual Component Framework is an Open Source project, so feel free to grab and use it if you think it might be useful. If you're really adventuresome, you can volunteer to help develop it, making it even better, especially in tying it into the VC++ environment as an add-in. For more information on either the project, helping out, or just browsing the doxygen generated documentation, please go to the VCF project on Source Forge here, or the project website. The code is available from CVS (follow the how-to here for setting up CVS on Windows), or a tar.gz file in files section of the project.
Introduction
This article will explain the very basics of creating a Hello World application with the Visual Component Framework. The Visual Component Framework (VCF) is designed to be a free, open, modern C++ framework, that while currently only runs on Win32, is architected to be relatively easy to port to other platforms, particularly Linux and Mac OSX. It is easy to use, and has an API similar to Java. With that said, let's get the ball rolling!
This simple application will demonstrate creating a VCF::Application
instance and displaying a main window, with "Hello World" in its caption bar.
The simplest full VCF application, in other words, one that uses the Application
class as its starting point, as opposed to just using certain pieces of it, needs only a few lines of code in a standard C main function, like the following:
int main(int argc, char *argv[])
{
Application app;
Application::appMain( argc, argv );
}
Those two lines of code are all that is needed to create your app instance on the stack, and then start the app running by calling the static Application::appMain()
function. If you are using the VCF Application
class features, like we are here, then there can only be one instance of an Application
derived class for the running process, much like the restrictions that apply to MFC's CWinApp
. Once you have this instance, it is necessary to start the app up by calling Application::appMain()
function, passing in the number of arguments and the argument array of strings. This is processed internally and calls the application's initRunningApplication()
and then the run loop, which starts the message pump up. From there on in, you are processing Windows messages.
Well, this by itself is not that interesting, so let's add a window.
int main(int argc, char *argv[])
{
Application app;
Window* mainWindow = new Window();
Application::appMain( argc, argv );
}
This creates our new window on the heap. This is the preferred way of creating most objects in the VCF, with a few exceptions. Next, we need to set the application's main window. In doing this, the application is able to register itself as a listener to the Windows window events (via a call to addWindowListener()
), and will be notified when the window closes itself. At this point, the application will in turn terminate itself cleanly and shut down. Failure to do this will prevent the application of being notified to close correctly, and it will not be able to free up the heap based memory for the Window
object.
int main(int argc, char *argv[])
{
Application app;
Window* mainWindow = new Window();
app.setMainWindow( mainWindow );
Application::appMain( argc, argv );
}
Well, this is still pretty boring, so let's set the window's caption and then display it.
int main(int argc, char *argv[])
{
Application app;
Window* mainWindow = new Window();
app.setMainWindow( mainWindow );
mainWindow->setCaption( "Hello World" );
mainWindow->show();
Application::appMain( argc, argv );
}
And there you have it: a window will magically display itself, with a caption that reads "Hello World". The caption is set via the setCaption()
method, and the window is made visible via the show()
method. Alternatively, we could have made the window visible by calling the setVisible()
with a true bool
value.
Most of this was pretty easy, with the only odd thing the missing WinMain()
. This is circumvented by setting some custom linker settings: in the output section of the project setting's Link tab, you specify mainCRTStartup
as the Entry-point symbol, and make sure your /subsystem
flag is set to /subsystem:windows
, not /subsystem:console
. With this set, you can go about your merry way and still use main()
just like normal.