Partial Rendering Control using JQuery in MVC 2






4.17/5 (8 votes)
This article shows a web custom control that allows partial rendering using JQuery in an MVC 2 web application
Introduction
In this article, I'm going to show web custom control that allows to have partial rendering using async post-back (through JQuery) in an MVC 2 web application.
I'm not going to explain "the core" of that control because it's just an MVC version of the previous control that I already shown in another article. If you want to have more information about the engine, you can find a good explanation on "Partial rendering control using JQuery" (you can find it in my article section). Even if that control is an ASP.NET version, the "core" concept is very close to the one shown here.
Using the Code
The goal of this control is to help the developer to have partial rendering without dealing with JQuery and complex client template. Let's go for an example to better understand how we can use it.
First of all, we have to create an MVC 2 Web application. Just open Visual Studio 2010 --> new project --> MVC 2 application.
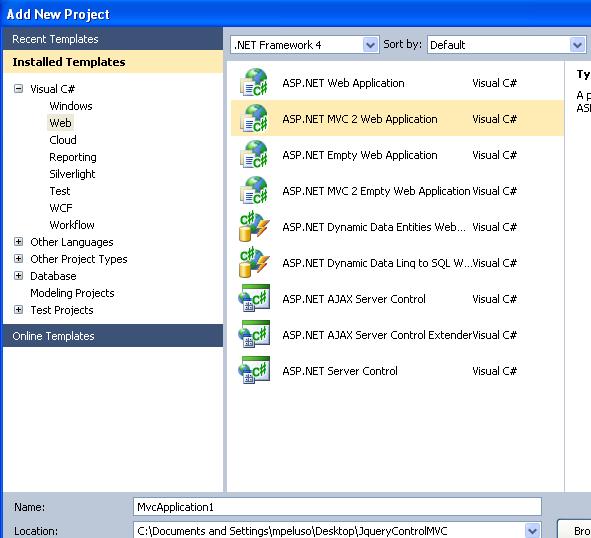
Now we have to add the JqueryControl
to the toolbox clicking on it and selecting "choose item".
Click on Browse and select the "JqueryController.dll" from JQueryControlMVC2.zip\JqueryController\bin\Debug.
Now open index.aspx under view, then drag and drop the JqueryControl
on the page. This is going to be the container of the portion of the page we want to refresh (partial rendering).
At this point, we have to create a template that represents that portion of the page. To commit that, just add a UserViewControl
to the page by clicking on add new item and selecting ViewUserControl
MVC 2:
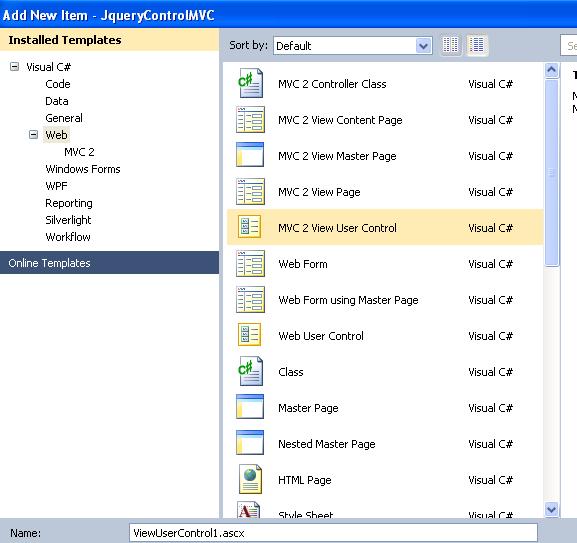
This is going to be the portion of the page we want to refresh. In this example, we are going to show the current time. When the user clicks on a button we want refresh the time using partial rendering. This is just an example to focus on the JQuery control functionality but you can use this approach in a more complex context. The last task we have to do is to create the model that is going to pull back the information we want to show on the view. In this example, the model is just a class with a property that returns the current time.
public class TimeModel
{
public string time
{
get { return DateTime.Now.ToShortDateString(); }
}
}
}
At this point, we have to add that user control into the JqueryController
. This is going to be the portion of the page we want to refresh. It doesn't matter how complex the interface we have is. We can use exactly the same approach. The last thing we need is to add the web control that is going to raise the postback. Add an HTML button to the page.
At this point, the page source should look like the below:
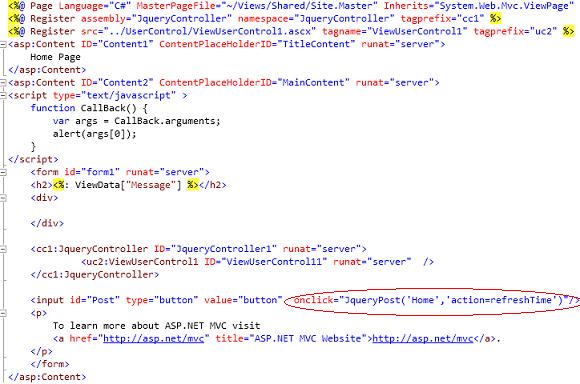
The last thing we need is to add some JavaScript code to the click event of the button. As you can see, we are going to call a "special" JavaScript function called "JqueryPost
". This function must have as first parameter the action we want to call. We can also pass any parameters we want to have on the controller side. Now let's focus on the controller just to see how easy it is to have partial rendering using this web control. To make the web control work, the controller has to inherit from MvcControllerBase
. When the user clicks on the button, the homeController
is going to be called. The controller inherits a property called "PostParameter
" from MVCControllerBase
that contains all the parameters we send along with the request and "IsPartialRendering
" that is a Boolean property to understand if the post back has been raised by the JqueryPost
function. At this point on the controller we are able to understand what we need to do, checking the parameter that has been sent with the request. In this example, we just check if the parameter "action=refreshTime
". In this case, we create an instance of the model then we just need to call "RefreshJQueryPanel
" to refresh the portion of the page on the client side.
[HandleError]
public class HomeController : MvcControllerBase
{
private const string UserControlPath="~/Views/UserControl/ViewUserControl1.ascx";
public ActionResult Index()
{
ViewData["Message"] = "Welcome to ASP.NET MVC!";
return View();
}
[HttpPost]
public ActionResult Index(string x)
{
if (this.IsPartialRendering && this.PostParameter.ContainsKey("action"))
{
switch (this.PostParameter["action"])
{
case "refreshTime":
TimeModel t = new TimeModel();
return this.RefreshJQueryPanel("JqueryController1",
UserControlPath, t, "CallBack", "parameterToReturn");
break;
//any other action
}
}
return View();
}
As you can see, "RefreshJQueryPanel
" is a generic function that again the controller inherits from MVCControllerBase
. It gets in input the ID of the JqueryControl
, the path of the userViewControl
and the model to pass to the view user control. The function is generic to also get the type of the model to easier bind it to the userViewControl
(using a strong-typed model). In this case, we use an overload of that method that also accepts a name of a JavaScript callback function we want to call on the client and any parameter to pass to that function. In this example, after the client refreshes it calls the JavaScript function "Call Back" on the client passing the string parameter "parameterToReturn
". In this example, this function just shows a message box but in a real context we could have some parameters back to initialize again the interface for example or for any reason we could need them.
Points of Interest
The scope of this control is just to help the developer to have JQuery partial rendering without dealing with JQuery and using a web control approach to make this task easier to commit. Most of the articles show an approach where JQuery is used to do a call and get JSON back. After that, usually there is a kind of binding between that JSON and the interface. That binding can be implemented using a different way like pure JavaScript, JQuery or any third-part library to makes this task easier. With the approach, I have shown that it easier because the user control used on the page is the same used as template for the partial rendering so that we don't need to spend time to replicate the binding logic on the client side.