A purist hello world app: an IPhone project template without .XIB
A step-by-step Guide to getting a pure code application template.
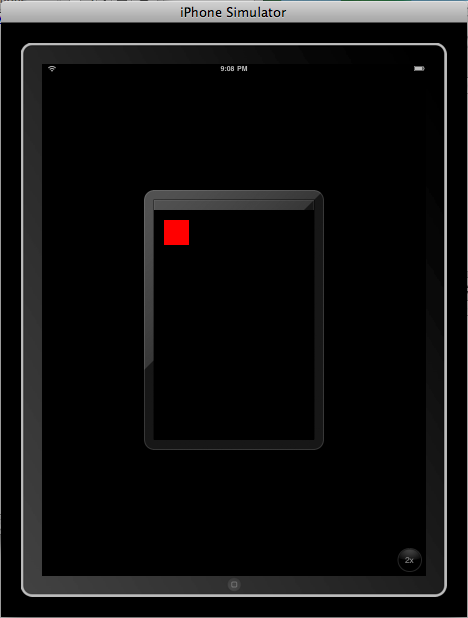
I have gone through quite a few hello world tutorials for the iPhone, and they always reach the point of saying ' and now some magic occurs. You don't need to worry about this... the .XIB takes care of it '
I don't like this. what if I have a project where I am not going to be using IB? it don't want a large invisible object doing unknown things to my code. that makes me feel uneasy.
So, here is a step-by-step Guide to getting a pure code application template. I am using Xcode 3 -- it should be pretty similar for other versions.
- create new project -> view based application "NoXIB"
- delete both .xib files
- open Info.plist, locate the key: "Main nib file base name:" and delete "MainWindow"
- fiddle main.m
- can remove the IBOutlet from here:
- create the window and the view controller here:
- create a view; groups and files pane, right click on NoXIB -> 'classes', add new file, "MyView".
- make sure it is a subclass of UIView.
- override its drawRect so that it draws a red rectangle:
- make the view controller load this view
// // main.m // noXIB // // Created by pi on 13/08/2010. // #import <UIKit/UIKit.h> int main(int argc, char *argv[]) { NSAutoreleasePool * pool = [[NSAutoreleasePool alloc] init]; int retVal = UIApplicationMain(argc, argv, nil, @"noXIBAppDelegate"); [pool release]; return retVal; }
// // noXIBAppDelegate.h // noXIB // // Created by pi on 13/08/2010. // #import <UIKit/UIKit.h> @class noXIBViewController; @interface noXIBAppDelegate : NSObject <UIApplicationDelegate> { UIWindow *window; noXIBViewController *viewController; } @property (nonatomic, retain) /*IBOutlet*/ UIWindow *window; @property (nonatomic, retain) /*IBOutlet*/ noXIBViewController *viewController; @end
// // noXIBAppDelegate.m // noXIB // // Created by pi on 13/08/2010. // #import "noXIBAppDelegate.h" #import "noXIBViewController.h" @implementation noXIBAppDelegate @synthesize window; @synthesize viewController; - (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions { self.window = [[[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]] autorelease];; self.viewController = [[[noXIBViewController alloc] init] autorelease]; // Override point for customization after app launch [window addSubview:viewController.view]; [window makeKeyAndVisible]; return YES; }
// // MyView.m // noXIB // // Created by pi on 13/08/2010. // #import "MyView.h" @implementation MyView : // Only override drawRect: if you perform custom drawing. // An empty implementation adversely affects performance during animation. - (void)drawRect:(CGRect)rect { // Drawing code // Get the graphics context and clear it CGContextRef ctx = UIGraphicsGetCurrentContext(); CGContextClearRect(ctx, rect); // draw a red rectangle CGContextSetRGBFillColor(ctx, 255, 0, 0, 1); CGContextFillRect(ctx, CGRectMake(10, 10, 50, 50)); }
// // noXIBViewController.m // noXIB // // Created by pi on 13/08/2010. // #import "noXIBViewController.h" #import "MyView.h" @implementation noXIBViewController // Implement loadView to create a view hierarchy programmatically, without using a nib. - (void)loadView { [super loadView]; CGRect frame = CGRectMake(10, 10, 300, 300); // 320 x 460 MyView* myV = [ [ [MyView alloc] initWithFrame:frame] autorelease]; myV.clipsToBounds = YES; [[self view] addSubview: myV]; } : :
Bingo! No XIB!