Lambda Expression in C# 3.0






4.23/5 (15 votes)
Lambda Expression is one of the best features of C# 3.0
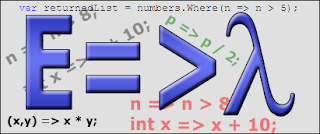
Lambda Expression is one of the best features of C# 3.0. Lambda expression is the same as anonymous method introduced in C# 2.0. Lambda Expression is a concise syntax to achieve the same goal as anonymous method. Now we can summarize Lambda expression in one line.
“Lambda expression is simply a Method.”
Syntax of Lambda Expression
Input Parameters => Expression/Statement Block;
Left hand side of expression contains zero or more parameters followed by Lambda operator ‘=>’ which is read as “goes to” and right hand side contains the expression or Statement block.
A simple Lambda expression:
x => x * 2
This Lambda expression is read as “x goes to x times 2”. Lambda Operator “=>” has the same precedence as assignment “=” operator. This simple expression takes one parameter “x” and returns the value “x*2”.
Parameters Type
The parameters of the lambda expression can be explicitly or implicitly typed. For example:
(int p) => p * 4; // Explicitly Typed Parameter
q => q * 4; // Implicitly Typed Parameter
Explicit typed parameter is the same as parameters of method where you explicitly specified the type of parameter. In an implicit typed parameter, the type of parameter is inferred from the context of lambda expression in which it occurs.
Type Inference is a new feature of C# 3.0. I will explain it in some other blog.
Use Simple Lambda Expression
Here is a simple example of Lambda Expression which returns a list of numbers greater than 8.
int[] numbers = {1,2,3,4,5,6,7,8,9,10 };
var returnedList = numbers.Where(n => (n > 8));
You can also use anonymous method for returning the same list.
int[] numbers = {1,2,3,4,5,6,7,8,9,10 };
var returnedList = numbers.Where(delegate(int i) { return i > 8; });
Use Statement Block in Lambda Expression
Here is a simple example to write a statement block in the lambda expression. This expression returns a list of numbers less than 4 and greater than 8.
int[] numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
var returnedList = numbers.Where(n =>
{
if (n < 4)
return true;
else if (n > 8)
return true;
return false;
}
);
Use Lambda with More than One Parameter
You can also write lambda which takes more than one parameter. Here is an example of lambda which adds two integer numbers:
delegate int AddInteger(int n1, int n2);
AddInteger addInt = (x, y) => x + y;
int result = addInt(10,4); // return 14
Use Lambda with Zero Parameter
Here is an example of lambda which takes no parameter and returns new Guid.
delegate Guid GetNextGuid();
GetNextGuid getNewGuid = () => (Guid.NewGuid());
Guid newguid = getNewGuid();
Use Lambda that Returns Nothing
You can also write lambda which returns void
. Here is an example that lambda is only showing message and returns nothing.
delegate void ShowMessageDelegate();
ShowMessageDelegate msgdelegate = () => MessageBox.Show("It returns nothing.");
msgdelegate();
Some Build in Delegates
.NET Framework 3.0 provides some build in parameterized delegate types that is “Func<T>(...)
” and also provides some parameterized delegates that return void
that is “Action<T>(...)
”.